1.forward_list内存结构
forward_list是单向链表,内存结构与list类似,只不过是单向的
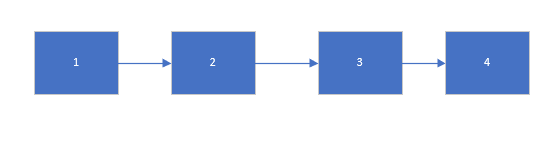
2.forward_list容量操作
函数 |
功能 |
bool empty() |
检查容器是否为空 |
size_t max_size() |
返回容器由于系统或库的实现所限制可容纳的元素最大数量 |
void resize(size_t new_size, const T& x) |
重设容器的size大小,如果size变大,则以默认值填充变长的位置,如果size变小,则删除变短那部分的尾部元素 |
void test_capacity(){
forward_list<int> test;
cout << "*************************after init****************************\n";
cout << "empty(): " << test.empty() << " max_size(): " << test.max_size() << endl;
cout << "*************************after push_back****************************\n";
test.push_front(1);
cout << "empty(): " << test.empty() << " max_size(): " << test.max_size() << endl;
cout << "*************************after resize(5)****************************\n";
test.resize(5);
cout << "empty(): " << test.empty() << " max_size(): " << test.max_size() << endl;
}
结果:
*************************after init****************************
empty(): 1 max_size(): 576460752303423487
*************************after push_back****************************
empty(): 0 max_size(): 576460752303423487
*************************after resize(5)****************************
empty(): 0 max_size(): 576460752303423487
3.forward_list构造
函数 |
功能 |
list() |
默认构造函数,构造一个空容器 |
list(size_t n) |
构造具有n个默认值的容器 |
list(size_t n, const T& value) |
构造具有n个值为value的容器 |
list(first, last) |
构造拥有范围 [first, last) 内容的容器 |
list( const forward_list& other ) |
拷贝构造函数,构造一个拥有other内容的容器 |
list( forward_list&& other ) |
移动构造函数,用移动语义构造拥有other内容的容器,移动后other为empty() |
list( std::initializer_list init) |
构造拥有initializer_list init内容的容器 |
void test_construct(){
cout << "*************************forward_list()****************************\n";
forward_list<int> test;
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************forward_list(10)****************************\n";
forward_list<int> test1(10);
for(auto iter=test1.cbegin(); iter!=test1.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************forward_list(10,2)****************************\n";
forward_list<int> test2(10,2);
for(auto iter=test2.cbegin(); iter!=test2.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************forward_list(test2.begin(), test2.end())****************************\n";
forward_list<int> test3(test2.begin(), test2.end());
for(auto iter=test3.cbegin(); iter!=test3.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************forward_list(test2)****************************\n";
forward_list<int> test4(test2);
for(auto iter=test4.cbegin(); iter!=test4.cend(); ++iter){
cout << *iter << " ";
}
cout <<endl;
cout << "*************************forward_list(std::move(test2))****************************\n";
forward_list<int> test5(std::move(test2));
for(auto iter=test5.cbegin(); iter!=test5.cend(); ++iter){
cout << *iter << " ";
}
cout <<endl;
cout << "*************************forward_list{1,2,3,4,5}****************************\n";
forward_list<int> test6{
1,2,3,4,5};
for(auto iter=test6.cbegin(); iter!=test6.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
}
*************************forward_list()****************************
*************************forward_list(10)****************************
0 0 0 0 0 0 0 0 0 0
*************************forward_list(10,2)****************************
2 2 2 2 2 2 2 2 2 2
*************************forward_list(test2.begin(), test2.end())****************************
2 2 2 2 2 2 2 2 2 2
*************************forward_list(test2)****************************
2 2 2 2 2 2 2 2 2 2
*************************forward_list(std::move(test2))****************************
2 2 2 2 2 2 2 2 2 2
*************************forward_list{
1,2,3,4,5}****************************
1 2 3 4 5
4.forward_list元素访问
void test_access(){
forward_list<int>test{
1,2,3,4,5};
cout<< " front:" << test.front() << endl;
}
front:1
5.forward_list元素修改
函数 |
功能 |
push_front(const T& value) |
在头部插入新元素value |
insert_after(iterator pos, const T& value) |
在pos后插入元素value |
insert_after(iterator pos, size_t n, const T& value) |
在pos后插入n个元素value |
insert_after(iterator pos, iterator first, iterator last) |
在pos后插入来自范围 [first, last)的元素 |
erase_after(iterator pos) |
移除后随pos处的元素 |
erase_after(iterator first, iterator last) |
移除后随first且于last之前的元素 |
emplace_front(Args&&… args) |
在容器头部就地构造元素 |
pop_front() |
删除头部元素 |
swap(forward_list& other) |
将内容与other交换。不在单个元素上调用任何移动、复制或交换操作 |
clear() |
清除所有元素 |
void test_modifier(){
forward_list<int>test;
cout << "*************************push_front(2)****************************\n";
test.push_front(2);
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************insert_after(test.begin(),2)****************************\n";
test.insert_after(test.begin(), 2);
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************insert_after(test.begin(),3,4)****************************\n";
test.insert_after(test.begin(), 3, 4);
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************insert_after(test.begin(),test2.begin(),test2.end())****************************\n";
forward_list<int>test2{
1,2,3,4,5};
test.insert_after(test.begin(), test2.begin(), test2.end());
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************erase_after(test.begin())****************************\n";
test.erase_after(test.begin());
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************erase_after(test.begin(),test.end())****************************\n";
test.erase_after(test.begin(), test.end());
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
class Book{
public:
Book(const std::string& name):m_name(name){
}
inline std::string getName(){
return m_name;}
private:
std::string m_name;
};
forward_list<Book> test_book;
cout << "*************************emplace_front****************************\n";
test_book.emplace_front("Effective C++");
for(auto iter=test_book.begin(); iter!=test_book.end(); ++iter){
cout << iter->getName() << " ";
}
cout << endl;
for(int i=0;i<5;++i){
test.push_front(i);
}
cout << "*************************pop_front()****************************\n";
test.pop_front();
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************test.swap(test2)****************************\n";
test.swap(test2);
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************clear()****************************\n";
test.clear();
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
}
*************************push_front(2)****************************
2
*************************insert_after(test.begin(),2)****************************
2 2
*************************insert_after(test.begin(),3,4)****************************
2 4 4 4 2
*************************insert_after(test.begin(),test2.begin(),test2.end())****************************
2 1 2 3 4 5 4 4 4 2
*************************erase_after(test.begin())****************************
2 2 3 4 5 4 4 4 2
*************************erase_after(test.begin(),test.end())****************************
2
*************************emplace_front****************************
Effective C++
*************************pop_front()****************************
3 2 1 0 2
*************************test.swap(test2)****************************
1 2 3 4 5
*************************clear()****************************
6.forward_list操作
函数 |
功能 |
merge(forward_list& other) |
将两个已排序的链表合并为一个排序链表 |
splice_after(const iterator pos, forward_list& other) |
从other转移所有元素到本list,元素插入到pos之后,不复制或移动元素 |
splice_after(const iterator pos, forward_list& other, const iterator it) |
从other转移it指向的元素到本list,元素插入到pos之后,不复制或移动元素 |
splice_after(const iterator pos, forward_list& other, const iterator first, const iterator last) |
从other转移范围[first,last)的元素到本list,元素插入到pos之后,不复制或移动元素 |
reverse() |
逆转容器中的元素顺序 |
unique() |
移除元素中相继重复的元素 |
sort() |
元素排序 |
void test_operation(){
cout << "*************************merge()****************************\n";
forward_list<int> test{
1,3,5,7};
forward_list<int> test2{
2,3,4,5,8};
test.merge(test2);
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************splice_after(test.begin(),test3)****************************\n";
forward_list<int> test3{
7,8,9};
test.splice_after(test.begin(), test3);
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************splice_after(test.begin(),test4, test4.begin())****************************\n";
forward_list<int> test4{
7,8,9};
test.splice_after(test.begin(), test4, test4.begin());
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************splice_after(test.begin(),test4,test4.begin(),test4.end())****************************\n";
test.splice_after(test.begin(), test4, test4.begin(), test4.end());
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************reverse()****************************\n";
test.reverse();
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************unique()****************************\n";
test.unique();
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
cout << "*************************sort()****************************\n";
test.sort();
for(auto iter=test.cbegin(); iter!=test.cend(); ++iter){
cout << *iter << " ";
}
cout << endl;
}
*************************merge()****************************
1 2 3 3 4 5 5 7 8
*************************splice_after(test.begin(),test3)****************************
1 7 8 9 2 3 3 4 5 5 7 8
*************************splice_after(test.begin(),test4, test4.begin())****************************
1 8 7 8 9 2 3 3 4 5 5 7 8
*************************splice_after(test.begin(),test4,test4.begin(),test4.end())****************************
1 9 8 7 8 9 2 3 3 4 5 5 7 8
*************************reverse()****************************
8 7 5 5 4 3 3 2 9 8 7 8 9 1
*************************unique()****************************
8 7 5 4 3 2 9 8 7 8 9 1
*************************sort()****************************
1 2 3 4 5 7 7 8 8 8 9 9