使用hibernate肯定要配置hibernate环境,之前的文章里提过,这里就不过多的赘述,直接进行增删改查的程序
首先是书写单例模式的sessionfactory
- package Usertest;
- import org.hibernate.HibernateException;
- import org.hibernate.SessionFactory;
- import org.hibernate.cfg.Configuration;
- public class HibernateUtil {
- private static Configuration cfg;
- private static SessionFactory sf;
- static
- {
- try {
- cfg = new Configuration().configure();
- sf = cfg.buildSessionFactory();
- } catch (HibernateException e) {
- // TODO Auto-generated catch block
- e.printStackTrace();
- }
- }
- public static SessionFactory getSessionFactory(){
- return sf;
- }
- }
测试类:
- package Usertest;
- import org.hibernate.HibernateException;
- import org.hibernate.Session;
- import org.hibernate.SessionFactory;
- import org.hibernate.Transaction;
- import org.hibernate.cfg.Configuration;
- import org.junit.Test;
- import text.User;
- public class UserDAO {
- @Test
- public void SaveUser(){//保存用户
- // TODO Auto-generated method stub
- SessionFactory sf = null;
- Session session = null;
- Transaction ts = null;
- try {
- sf = HibernateUtil.getSessionFactory();
- session = sf.getCurrentSession();
- ts = session.beginTransaction();
- User user = new User();
- user.setAge(18);
- user.setUsername("小王");
- user.setGender("男");
- user.setPassword("1245456");
- session.save(user);
- ts.commit();
- } catch (HibernateException e) {
- // TODO Auto-generated catch block
- if(ts != null)
- {
- ts.rollback();
- }
- e.printStackTrace();
- }
- }
- @Test
- public void getUser(){//查询用户
- // TODO Auto-generated method stub
- SessionFactory sf = null;
- Session session = null;
- Transaction ts = null;
- try {
- sf = HibernateUtil.getSessionFactory();
- session = sf.getCurrentSession();
- ts = session.beginTransaction();
- User user =session.get(User.class, 1);//session的get方法
- System.out.println(user.getUsername());
- ts.commit();
- } catch (HibernateException e) {
- // TODO Auto-generated catch block
- if(ts != null)
- {
- ts.rollback();
- }
- e.printStackTrace();
- }
- }
- @Test
- public void updateUser(){//修改用户
- // TODO Auto-generated method stub
- SessionFactory sf = null;
- Session session = null;
- Transaction ts = null;
- try {
- sf = HibernateUtil.getSessionFactory();
- session = sf.getCurrentSession();
- ts = session.beginTransaction();
- User user1=session.get(User.class, 2);
- user1.setPassword("1514010601");
- ts.commit();
- } catch (HibernateException e) {
- // TODO Auto-generated catch block
- if(ts != null)
- {
- ts.rollback();
- }
- e.printStackTrace();
- }
- }
- @Test
- public void delUser(){//删除用户
- // TODO Auto-generated method stub
- SessionFactory sf = null;
- Session session = null;
- Transaction ts = null;
- User user =new User();
- user.setUsername("Jenny");
- user.setAge(11);
- user.setGender("male");
- user.setPassword("123456");
- try {
- sf = HibernateUtil.getSessionFactory();
- session = sf.getCurrentSession();
- ts = session.beginTransaction();
- session.delete(user);
- System.out.println("删除成功了!");
- ts.commit();
- } catch (HibernateException e) {
- // TODO Auto-generated catch block
- if(ts != null)
- {
- ts.rollback();
- }
- e.printStackTrace();
- }
- }
- }
增加
删除
修改
查询
扫描二维码关注公众号,回复:
1573829 查看本文章
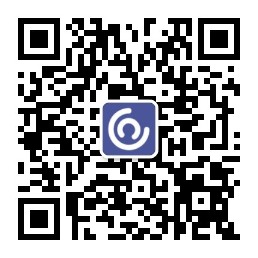