目录
R7-5 Count the letters in a string (统计字符串中的字符)
前言:
临近期末,我也更新一下PTA上的JAVA大题,希望各位都可以考出一个好的成绩。
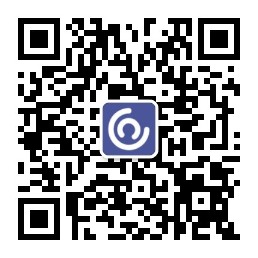
R7-5 Count the letters in a string (统计字符串中的字符)
(Count the letters in a string) (统计字符串中的字符)
Write a method that counts the number of letters in a string using the following header:
public static int countLetters(String s)
Write a test program that prompts the user to enter a string and displays the number of letters in the string.
(统计字符串中的字符) 使用以下标题编写一个计算字符串中字母数的方法:
public static int countLetters(String s)
编写一个程序,提示用户输入字符串并显示字符串中的字母数。
input style :
Enter a string
output style:
Displays the number of the letters in the string.
input sample:
qwe&&&,,;#@23qer
output sample:
The number of letters inside the string is: 6
代码:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
String s=sc.next();
System.out.println("The number of letters inside the string is: "+countLetters(s));
}
public static int countLetters(String s){
int c=0;
for (int i = 0; i < s.length(); i++) {
if(Character.isLetter(s.charAt(i))){
c++;
}
}
return c;
}
}
统计字符串中的字母其实非常简单,主要就是调用JAVA中的方法:
Character.isLetter(s.charAt(i))
根据它的返回值来判断字符串S中第i个字符是否是一个字母。
R7-1 找素数
请编写程序,从键盘输入两个整数m,n,找出等于或大于m的前n个素数。
输入格式:
第一个整数为m,第二个整数为n;中间使用空格隔开。例如:
103 3
输出格式:
从小到大输出找到的等于或大于m的n个素数,每个一行。例如:
103
107
109
输入样例:
9223372036854775839 2
输出样例:
9223372036854775907
9223372036854775931
这道题主要是需要调用JAVA MATH库中的BigInteger ,因为这里的例子都是超大数,因此需要用BigInteger来存储。
import java.util.Scanner;
import java.math.BigInteger;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
BigInteger m = input.nextBigInteger();
BigInteger n = input.nextBigInteger();
input.close();
BigInteger count = BigInteger.ZERO;
BigInteger num = m;
while (count.compareTo(n) < 0) {
if (num.isProbablePrime(100)) {
System.out.println(num);
count = count.add(BigInteger.ONE);
}
num = num.add(BigInteger.ONE);
}
}
}
R7-3 电话号码同步(Java)
文件phonebook1.txt和phonebook2.txt中有若干联系人的姓名和电话号码。请你设计一个程序,将这两个文件中的电话号码同步。(所谓同步,就是将两个文件中的电话号码合并后剔除相同的人名和电话号码。请将同步后的电话号码按照姓名拼音顺序排序后保存到文件phonebook3.txt中。)
由于目前的OJ系统暂时不能支持用户读入文件和写文件,我们编写程序从键盘输入文件中的姓名和电话号码,当输入的单词为end时,表示文件结束。将同步后的电话号码按照姓名拼音顺序排序后输出。
输入格式:
张三 13012345678
李四 13112340000
王五 13212341111
马六 13312342222
陈七 13412343333
孙悟空 13512345555
end (表示文件phonebook1.txt结束)
张三 13012345678
孙悟空 13512345555
王五 13212341111
陈七 13412343333
唐三藏 13612346666
猪悟能 13712347777
沙悟净 13812348888
end (表示文件phonebook2.txt结束)
输出格式:
陈七 13412343333
李四 13112340000
马六 13312342222
沙悟净 13812348888
孙悟空 13512345555
唐三藏 13612346666
王五 13212341111
张三 13012345678
猪悟能 13712347777
本题主要是考察我们对树这种数据结构的利用,其实不是很难。
import java.util.Iterator;
import java.util.Scanner;
import java.util.Set;
import java.util.TreeSet;
public class Main {
public static void main(String args[]){
Scanner in=new Scanner(System.in);
String string;
String string2;
Set<String> set=new TreeSet<String>();
Set<String> set2=new TreeSet<String>();
Set<String> set3=new TreeSet<String>();
while(in.hasNextLine()){
string=in.nextLine();
if(string.equals("end")){
break;
}
else{
set.add(string);
}
}
while(in.hasNextLine()){
string2=in.nextLine();
if(string2.equals("end")){
break;
}
else{
set2.add(string2);
}
}
set3.addAll(set);
set3.addAll(set2);
Iterator iterator=set3.iterator();
while(iterator.hasNext()){
System.out.println(iterator.next());
}
}
}
总结:
祝愿各位可以考出一个如意的成绩。暑假期间我会更新前端三件套,有兴趣的小伙伴可以和我一起学习,共同进步。
如果我的内容对你有帮助,请点赞,评论,收藏。创作不易,大家的支持就是我坚持下去的动力!