Given a binary tree, find the largest subtree which is a Binary Search Tree (BST), where largest means subtree with largest number of nodes in it.
Note:
A subtree must include all of its descendants.
Here's an example:
10 / \ 5 15 / \ \ 1 8 7
The Largest BST Subtree in this case is the highlighted one.
The return value is the subtree's size, which is 3.
题意:
给定二叉树,找出最大的二叉搜索树子树。
思路:
代码:
1 class Solution { 2 public int largestBSTSubtree(TreeNode root) { 3 if(root == null) return 0; 4 return dfs(root)._size; 5 } 6 7 private Result dfs(TreeNode root){ 8 if(root == null) return new Result (true, 0, 999, -999); 9 10 Result leftResult = dfs(root.left); 11 Result rightResult = dfs(root.right); 12 13 boolean isBST = ((root.right == null ||(rightResult._isBST) && (rightResult._min > root.val)) && (root.left == null || (leftResult._isBST)&&(leftResult._max < root.val ))); 14 int size = isBST ? (leftResult._size + rightResult._size +1) : Math.max(leftResult._size, rightResult._size); 15 int min = root.left == null ? root.val : leftResult._min; 16 int max = root.right == null ? root.val : rightResult._max; 17 return new Result(isBST, size, min, max); 18 19 } 20 21 private class Result{ 22 boolean _isBST; 23 int _size; 24 int _min; 25 int _max; 26 private Result(boolean isBST, int size, int min, int max){ 27 _isBST = isBST; 28 _size = size; 29 _min = min; 30 _max = max; 31 } 32 } 33 }
扫描二维码关注公众号,回复:
1579559 查看本文章
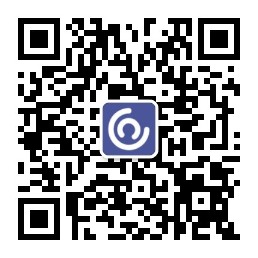