文章目录
优化设计TodoView
修复新增项目无法编辑问题
更新MyToDo.Api/Service/ToDoService.cs
public async Task<ApiReponse> AddAsync(Todo model)
{
try
{
var todo = mapper.Map<Todo>(model);
await work.GetRepository<Todo>().InsertAsync(todo);
if (await work.SaveChangesAsync() > 0)
return new ApiReponse(true, todo);
return new ApiReponse(false);
}
catch (Exception ex)
{
return new ApiReponse(false, ex);
}
}
更新MyToDo.Api/Service/MemoService.cs
public async Task<ApiReponse> AddAsync(Memo model)
{
try
{
var memo = mapper.Map<Memo>(model);
await work.GetRepository<Memo>().InsertAsync(memo);
if (await work.SaveChangesAsync() > 0)
return new ApiReponse(true, memo);
return new ApiReponse(false);
}
catch (Exception ex)
{
return new ApiReponse(false, ex);
}
}
增加了对完成状态的区分
更新MyToDo.Api/Service/TodoView.xaml
<ItemsControl.ItemTemplate>
<DataTemplate>
<Border MinWidth="200" Margin="10">
<Border.Style>
<Style TargetType="Border">
<Style.Triggers>
<DataTrigger Binding="{Binding Status}" Value="0">
<Setter Property="Background" Value="#1E90FF" />
</DataTrigger>
<DataTrigger Binding="{Binding Status}" Value="1">
<Setter Property="Background" Value="#3CB371" />
</DataTrigger>
</Style.Triggers>
</Style>
</Border.Style>
<Grid MinHeight="150">
增加了选项卡删除功能
更新删除请求URI
更新MyToDo.Api/Service/Baservice.cs
public async Task<ApiResponse> DeleteAsync(int id)
{
BaseRequest request = new BaseRequest();
request.Method = RestSharp.Method.DELETE;
request.Route = $"api/{ServiceName}/Delete?todoid={id}";
return await client.ExecuteAsync(request);
}
添加删除命令并初始化
更新文件:MyToDo/ViewModel/TodoViewModel.cs
添加内容:
/// <summary>
/// 删除项
/// </summary>
public DelegateCommand<ToDoDto> DeleteCommand { get; set; }
/// <summary>
/// 删除指定项
/// </summary>
/// <param name="dto"></param>
async private void DeleteItem(ToDoDto dto)
{
var delres = await service.DeleteAsync(dto.Id);
if (delres.Status)
{
var model = TodoDtos.FirstOrDefault(t => t.Id.Equals(dto.Id));
TodoDtos.Remove(dto);
}
}
更新内容
public TodoViewModel(ITodoService service,IContainerProvider provider) : base(provider)
{
//初始化对象
TodoDtos = new ObservableCollection<ToDoDto>();
RightContentTitle = "添加血雨待办";
//初始化命令
SelectedCommand = new DelegateCommand<ToDoDto>(Selected);
OpenRightContentCmd = new DelegateCommand(Add);
ExecuteCommand = new DelegateCommand<string>(ExceuteCmd);
DeleteCommand = new DelegateCommand<ToDoDto>(DeleteItem);
this.service = service;
}
UI添加删除按钮
更新文件:MyToDo/Views/TodoView.cs
更新内容:
扫描二维码关注公众号,回复:
15943283 查看本文章
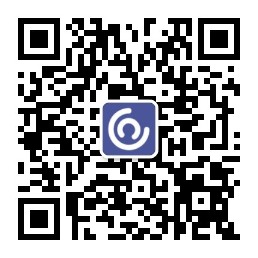
<Grid MinHeight="150">
<!-- 给项目添加行为 -->
<i:Interaction.Triggers>
<i:EventTrigger EventName="MouseLeftButtonUp">
<i:InvokeCommandAction Command="{Binding DataContext.SelectedCommand, RelativeSource={RelativeSource Mode=FindAncestor, AncestorType=ItemsControl}}" CommandParameter="{Binding}" />
</i:EventTrigger>
</i:Interaction.Triggers>
<Grid.RowDefinitions>
<RowDefinition Height="auto" />
<RowDefinition />
</Grid.RowDefinitions>
<DockPanel Panel.ZIndex="2" LastChildFill="False">
<TextBlock
Margin="10,10"
FontFamily="黑体"
FontSize="14"
Text="{Binding Title}" />
<!--<md:PackIcon
Margin="10,10"
VerticalContentAlignment="Top"
DockPanel.Dock="Right"
Kind="More" />-->
<md:PopupBox
Margin="5"
Panel.ZIndex="1"
DockPanel.Dock="Right">
<Button
Panel.ZIndex="2"
Command="{Binding DataContext.DeleteCommand, RelativeSource={RelativeSource Mode=FindAncestor, AncestorType=ItemsControl}}"
CommandParameter="{Binding}"
Content="删除" />
</md:PopupBox>
</DockPanel>
更改控制器
更新文件:MyToDo.Api/Controllers/TodoController.cs
更新内容:
public async Task<ApiReponse> Delete(int todoid)=> await service.DeleteAsync(todoid);
增加查询结果为空的图片
增加转换器
添加文件:MyToDo/Common/Converters/IntToVisibilityConveter.cs
更新内容:
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Data;
using System.Windows.Media;
namespace Mytodo.Common.Converters
{
[ValueConversion(typeof(Color), typeof(Brush))]
public class ColorToBrushConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (value is Color color)
{
return new SolidColorBrush(color);
}
return Binding.DoNothing;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
if (value is SolidColorBrush brush)
{
return brush.Color;
}
return default(Color);
}
}
}
修改UI
添加资源、命名空间
更新文件:MyToDo/Views/Converters/TodoView.xaml.cs
更新内容:
xmlns:cv="clr-namespace:Mytodo.Common.Converters"
<UserControl.Resources>
<ResourceDictionary>
<cv:IntToVisibilityConveter x:Key="IntToVisility" />
</ResourceDictionary>
</UserControl.Resources>
添加相关元素
FontSize="14" />
<StackPanel
Grid.Row="1"
VerticalAlignment="Center"
Visibility="{Binding TodoDtos.Count, Converter={StaticResource IntToVisility}}">
<Image
Width="120"
Height="120"
Source="/Images/nores.jpg" />
<TextBlock
Margin="0,10"
HorizontalAlignment="Center"
FontSize="18"
Text="尝试添加一些待办事项,以便在此处查看它们。" />
</StackPanel>
<ItemsControl
增加了根据状态查询的功能
Mytodo.Service/ITodoService增加GetAllFilterAsync接口
using Mytodo.Common.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using MyToDo.Share.Models;
using MyToDo.Share.Contact;
using MyToDo.Share.Parameters;
using MyToDo.Share;
namespace Mytodo.Service
{
public interface ITodoService:IBaseService<ToDoDto>
{
Task<ApiResponse<PagedList<ToDoDto>>> GetAllFilterAsync(TodoParameter parameter);
}
}
修改了控制器
MyToDo.Api.Controllers/TodoController
[HttpGet]
public async Task<ApiReponse> GetAll([FromQuery] TodoParameter param) => await service.GetAllAsync(param);
增加IToDoService接口
MyToDo.Api.Service/IToDoService
namespace MyToDo.Api.Service
{
public interface IToDoService : IBaseService<Todo>
{
Task<ApiReponse> GetAllAsync(TodoParameter parameter);
}
}
增加所需字段,属性,以及所需要的方法
更新文件:Mytodo.ViewModels/TodoViewModel.cs
/// <summary>
/// 项目状态
/// </summary>
public int SelectIndex
{
get { return selectIndex; }
set { selectIndex = value; RaisePropertyChanged(); }
}
private int selectIndex;
/// <summary>
/// 保存消息
/// </summary>
private async void Save()
{
try
{
if (string.IsNullOrWhiteSpace(CurrDto.Title) || string.IsNullOrWhiteSpace(CurrDto.Content))
return;
UpdateLoding(true);
if(CurrDto.Id>0) //编辑项
{
var updateres = await service.UpdateAsync(CurrDto);
if (updateres.Status)
{
UpdateDataAsync();
}
else
{
MessageBox.Show("更新失败");
}
}
else
{
//添加项
var add_res = await service.AddAsync(CurrDto);
//刷新
if (add_res.Status) //如果添加成功
{
TodoDtos.Add(add_res.Result);
}
else
{
MessageBox.Show("添加失败");
}
}
}
catch
{
}
finally
{
IsRightOpen = false;
//卸载数据加载窗体
UpdateLoding(false);
}
}
/// <summary>
/// 打开待办事项弹窗
/// </summary>
void Add()
{
CurrDto = new ToDoDto();
IsRightOpen = true;
}
private void Query()
{
GetDataAsync();
}
/// <summary>
/// 根据条件更新数据
/// </summary>
async void UpdateDataAsync()
{
int? Status = SelectIndex == 0 ? null : SelectIndex == 2 ? 1 : 0;
var todoResult = await service.GetAllFilterAsync(new MyToDo.Share.Parameters.TodoParameter { PageIndex = 0, PageSize = 100, Search = SearchString, Status = Status });
if (todoResult.Status)
{
todoDtos.Clear();
foreach (var item in todoResult.Result.Items)
todoDtos.Add(item);
}
}
/// <summary>
/// 获取所有数据
/// </summary>
async void GetDataAsync()
{
//调用数据加载页面
UpdateLoding(true);
//更新数据
UpdateDataAsync();
//卸载数据加载页面
UpdateLoding(false);
}
UI层增加绑定的界面
<ComboBox Margin="5" SelectedIndex="{Binding CurrDto.Status}">
<ComboBoxItem Content="已完成" FontSize="12" />
<ComboBoxItem Content="未完成" FontSize="12" />
</ComboBox>
<StackPanel
Grid.Row="1"
VerticalAlignment="Center"
Visibility="{Binding TodoDtos.Count, Converter={StaticResource IntToVisility}}">
<md:PackIcon
Width="120"
Height="120"
HorizontalAlignment="Center"
Kind="ClipboardText" />
<TextBlock
Margin="0,10"
HorizontalAlignment="Center"
FontSize="18"
Text="尝试添加一些待办事项,以便在此处查看它们。" />
增加了IToDoService接口所对应的实现
public async Task<ApiReponse> GetAllAsync(QueryParameter parameter)
{
try
{
var repository = work.GetRepository<Todo>();
var todos = await repository.GetPagedListAsync(predicate:
x => string.IsNullOrWhiteSpace(parameter.Search) ? true : x.Title.Contains(parameter.Search),
pageIndex: parameter.PageIndex,
pageSize: parameter.PageSize,
orderBy: source => source.OrderByDescending(t => t.CreateDate));
return new ApiReponse(true, todos);
}
catch (Exception ex)
{
return new ApiReponse(ex.Message,false);
}
}
修复更新操作无法更新状态的bug
public async Task<ApiReponse> UpdateAsync(Todo model)
{
try
{
var dbtodo = mapper.Map<Todo>(model);
//获取数据
var resposity = work.GetRepository<Todo>();
//
var todo = await resposity.GetFirstOrDefaultAsync(predicate: x => x.Id.Equals(dbtodo.Id));
if(todo == null)
return new ApiReponse("修改失败,数据库中无给定条件的数据项",false);
todo.Title= dbtodo.Title;
todo.UpdateDate=DateTime.Now;
todo.CreateDate = dbtodo.CreateDate;
todo.Content = dbtodo.Content;
todo.Status = dbtodo.Status;
resposity.Update(todo);
if (await work.SaveChangesAsync() > 0)
return new ApiReponse(true);
return new ApiReponse(false);
}
catch (Exception ex)
{
return new ApiReponse(ex.Message, false);
}
}
修复当Search为空时查询失败的bug
Mytodo.Service/TodoService.cs
public async Task<ApiResponse<PagedList<ToDoDto>>> GetAllFilterAsync(TodoParameter parameter)
{
BaseRequest request = new BaseRequest();
request.Method = RestSharp.Method.GET;
var parameter_search = parameter.Search;
if(parameter_search==null)
{
request.Route = $"api/ToDo/GetAll?pageIndex={parameter.PageIndex}" +
$"&pageSize={parameter.PageSize}" +
$"&status={parameter.Status}";
}
else
request.Route = $"api/ToDo/GetAll?pageIndex={parameter.PageIndex}" +
$"&pageSize={parameter.PageSize}" +
$"&search={parameter.Search}" +
$"&status={parameter.Status}";
return await client.ExecuteAsync<PagedList<ToDoDto>>(request);
}