实现效果:一款轻快简洁的记事本App,值得拥有!
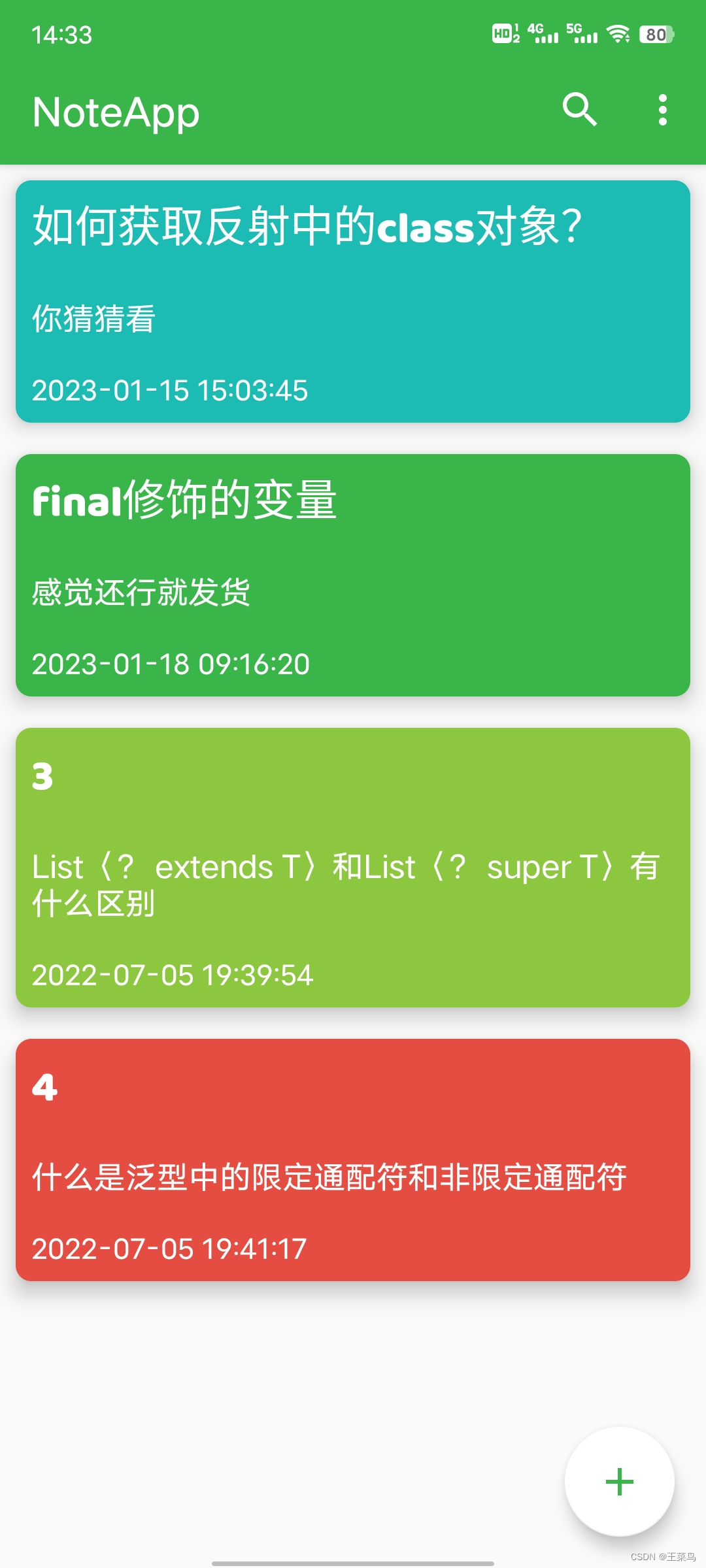
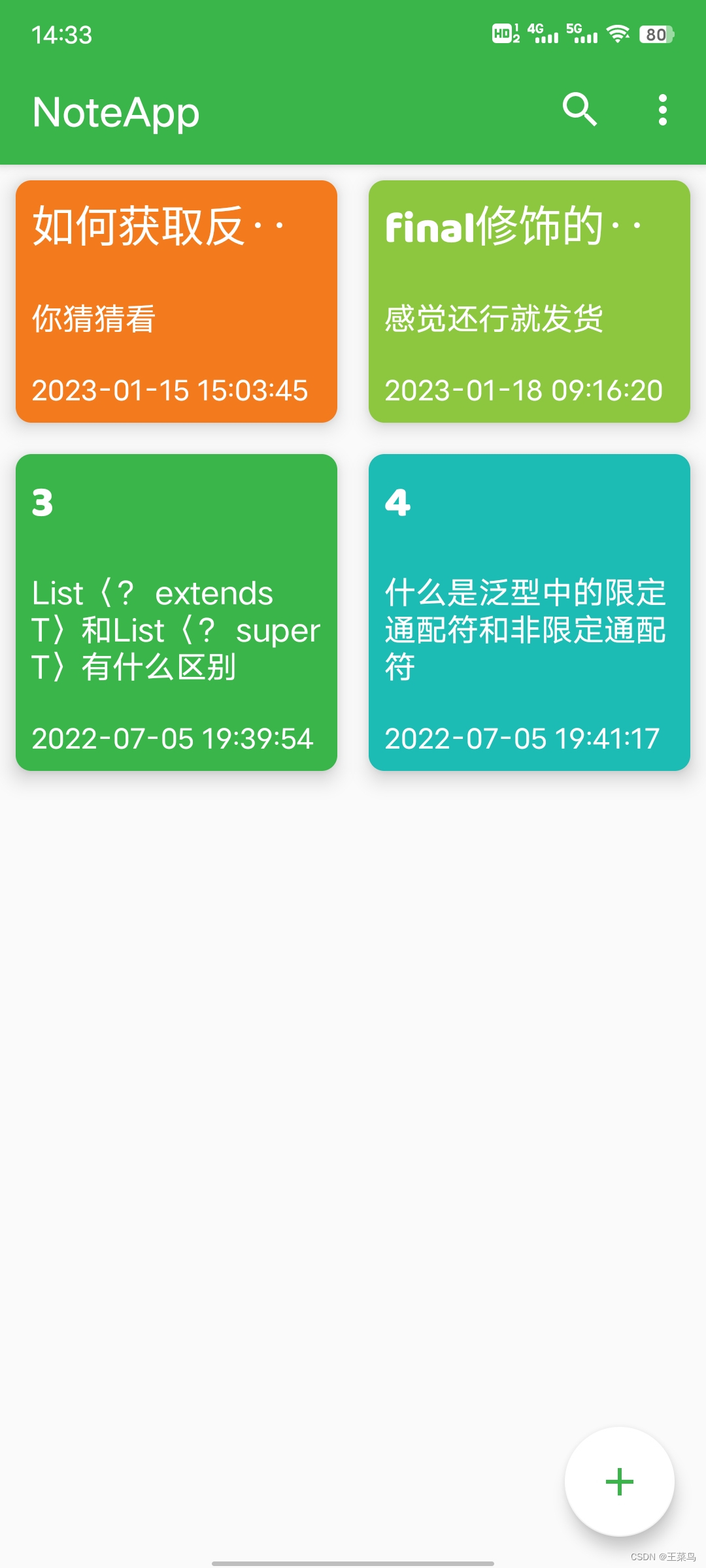
此音乐App开发环境:
Win10+Android Studio2020+JDK1.8 + Gradle6.5.1 +Android SDK32 + buildToolVersion32
所需依赖如下:
dependencies {
implementation fileTree(dir: "libs", include: ["*.jar"])
implementation 'androidx.appcompat:appcompat:1.1.0'
implementation 'androidx.constraintlayout:constraintlayout:1.1.3'
implementation 'androidx.recyclerview:recyclerview:1.1.0'
implementation 'com.google.android.material:material:1.0.0'
testImplementation 'junit:junit:4.12'
androidTestImplementation 'androidx.test.ext:junit:1.1.1'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0'
}
AndroidManifest配置了NoteApp的基本信息
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.xxxx.noteapp">
<!-- 存储权限 -->
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".AddActivity"></activity>
<activity android:name=".EditActivity" />
<activity android:name=".MainActivity"
android:exported="true"
android:requestLegacyExternalStorage="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<meta-data
android:name="preloaded_fonts"
android:resource="@array/preloaded_fonts" />
</application>
</manifest>
MainActivity的部分代码:
package com.xxxx.noteapp;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.appcompat.widget.SearchView;
import androidx.recyclerview.widget.GridLayoutManager;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import android.content.Intent;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import com.google.android.material.floatingactionbutton.FloatingActionButton;
import com.xxxx.noteapp.adapter.MyAdapter;
import com.xxxx.noteapp.bean.Note;
import com.xxxx.noteapp.db.NoteDBOpenHelper;
import com.xxxx.noteapp.utils.SpfUtil;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class MainActivity extends AppCompatActivity {
private RecyclerView recyclerView;
private FloatingActionButton floatBtn;
private List<Note> notes;
private MyAdapter myAdapter;
private NoteDBOpenHelper noteHelper;
public static final int MODE_LINEAR = 0;
public static final int MODE_GRID = 1;
public static final String KEY_LAYOUT_MODE = "key_layout_mode";
private int currentListLayoutMode = MODE_LINEAR;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
initData();
initEvent();
}
@Override
protected void onResume() {
super.onResume();
refreshData();
setListLayout();
}
// 初始化视图
private void initView() {
recyclerView = findViewById(R.id.reView);
}
// 初始化数据
private void initData() {
notes = new ArrayList<>();
noteHelper = new NoteDBOpenHelper(this);
for (int i = 0; i < 10; i++) {
Note note = new Note();
note.setTitle("这是标题");
note.setContent("这是内容");
note.setCreateTime(getCurrentTimeFormat());
notes.add(note);
}
}
private void initEvent() {
myAdapter = new MyAdapter(this, notes);
recyclerView.setAdapter(myAdapter);
setListLayout();
}
// 获取系统时间
private String getCurrentTimeFormat() {
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("YYYY年MM月dd日 HH:mm:ss");
Date date = new Date();
return simpleDateFormat.format(date);
}
// 刷新数据
private void refreshData() {
notes = getAllNote();
myAdapter.refresh(notes);
}
// 配置布局样式
public void setListLayout() {
currentListLayoutMode = SpfUtil.getIntWithDefault(this, KEY_LAYOUT_MODE, MODE_LINEAR);
if (currentListLayoutMode == MODE_LINEAR) {
setToLinearList();
} else {
setToGridList();
}
}
// 获取所有日记
private List<Note> getAllNote() {
return noteHelper.queryAll();
}
private void setToLinearList() {
RecyclerView.LayoutManager linearLayoutManager = new LinearLayoutManager(this, LinearLayoutManager.VERTICAL, false);
recyclerView.setLayoutManager(linearLayoutManager);
myAdapter.setViewType(MyAdapter.TYPE_LINEAR_LAYOUT);
myAdapter.notifyDataSetChanged();
}
private void setToGridList() {
RecyclerView.LayoutManager gridLayoutManager = new GridLayoutManager(this, 2);
recyclerView.setLayoutManager(gridLayoutManager);
myAdapter.setViewType(MyAdapter.TYPE_GRID_LAYOUT);
myAdapter.notifyDataSetChanged();
}
public void add(View view) {
Intent intent = new Intent(this, AddActivity.class);
startActivity(intent);
}
// 选择菜单
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_main, menu);
SearchView searchView = (SearchView) menu.findItem(R.id.menu_search).getActionView();
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
return false;
}
@Override
public boolean onQueryTextChange(String newText) {
notes = noteHelper.queryByTitle(newText);
myAdapter.refresh(notes);
return false;
}
});
return super.onCreateOptionsMenu(menu);
}
@Override
public boolean onOptionsItemSelected(@NonNull MenuItem item) {
item.setChecked(true);
switch (item.getItemId()) {
case R.id.menu_linear:
setToLinearList();
currentListLayoutMode = MODE_LINEAR;
SpfUtil.saveInt(this, KEY_LAYOUT_MODE, MODE_LINEAR);
return true;
case R.id.menu_grid:
setToGridList();
currentListLayoutMode = MODE_GRID;
SpfUtil.saveInt(this, KEY_LAYOUT_MODE, MODE_GRID);
return true;
default:
return super.onOptionsItemSelected(item);
}
}
@Override
public boolean onPrepareOptionsMenu(Menu menu) {
if (currentListLayoutMode == MODE_LINEAR) {
MenuItem item = menu.findItem(R.id.menu_linear);
item.setChecked(true);
} else {
menu.findItem(R.id.menu_grid).setChecked(true);
}
return super.onPrepareOptionsMenu(menu);
}
}
需要此应用源码,可以联系我!知识付费!拒绝白嫖!