You are given two squares, one with sides parallel to the coordinate axes, and another one with sides at 45 degrees to the coordinate axes. Find whether the two squares intersect.
The interior of the square is considered to be part of the square, i.e. if one square is completely inside another, they intersect. If the two squares only share one common point, they are also considered to intersect.
The input data consists of two lines, one for each square, both containing 4 pairs of integers. Each pair represents coordinates of one vertex of the square. Coordinates within each line are either in clockwise or counterclockwise order.
The first line contains the coordinates of the square with sides parallel to the coordinate axes, the second line contains the coordinates of the square at 45 degrees.
All the values are integer and between −100−100 and 100100.
Print "Yes" if squares intersect, otherwise print "No".
You can print each letter in any case (upper or lower).
0 0 6 0 6 6 0 6
1 3 3 5 5 3 3 1
YES
0 0 6 0 6 6 0 6
7 3 9 5 11 3 9 1
NO
6 0 6 6 0 6 0 0
7 4 4 7 7 10 10 7
YES
In the first example the second square lies entirely within the first square, so they do intersect.
In the second sample squares do not have any points in common.
Here are images corresponding to the samples:

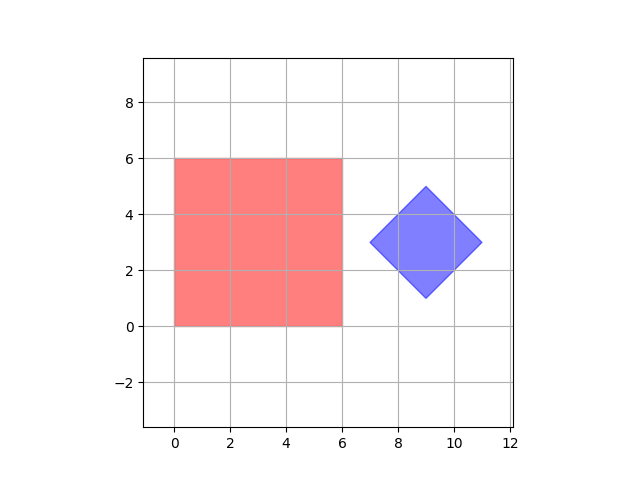
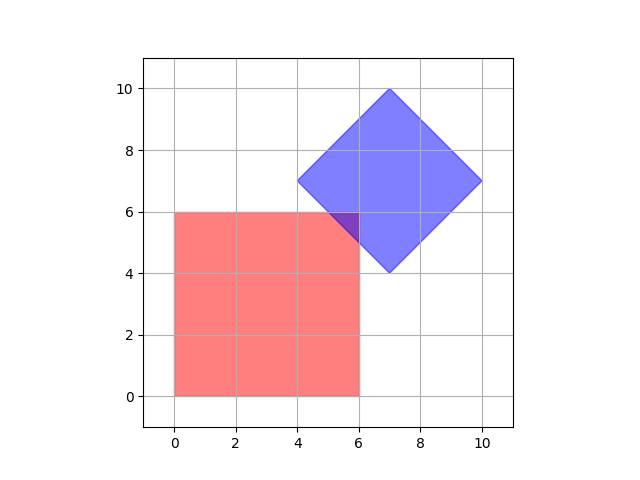
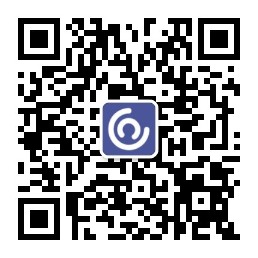
题意,两个正方形是否相交
题解:1.用叉积判断边是否相交
2.判断A的中心是否在B内或者B的中心是否在A内
叉积
判断两条线段是否相交
要判断两条线段是否相交,则需要检查每条线段是否跨越了另一条线段的直线。如果点p1位于某直线的一边,而点p2位于该直线的另一边,则称p1p2跨越了这条直线。两条线段相交,当且仅当下面两个条件至少成立一个:
每条线段都跨越了包含另一条线段的直线
一条线段的一个端点落在另一条线段上
#include<bits/stdc++.h> using namespace std; #define x first #define y second typedef pair<int, int> PII; int x[3][4], y[3][4];//两个正方形的坐标 PII p[3][4]; //叉积判断是否相交 int cross (PII p1, PII p2, PII p) { return (p2.x - p1.x) * (p.y - p1.y) - (p.x - p1.x) * (p2.y - p1.y); } bool ok (int i, int j) { int flag = 0; for (int k = 0; k < 4; k++) { //四个边用叉积判断是否相交或者同侧 if (cross (p[i][0], p[i][1], p[j][k]) *cross (p[i][2], p[i][3], p[j][k]) >= 0 && cross (p[i][1], p[i][2], p[j][k]) *cross (p[i][3], p[i][0], p[j][k]) >= 0) { //判断相交 //两边包一边的思想 flag = 1; } } //判断A中心是否在B内或者B中心是否在A内 int xx=(p[j][0].x + p[j][2].x) / 2; int yy=(p[j][1].y + p[j][3].y) / 2; PII px = PII ( xx, yy); if (cross (p[i][0], p[i][1], px) *cross (p[i][2], p[i][3], px) >= 0 && cross (p[i][1], p[i][2], px) *cross (p[i][3], p[i][0], px) >= 0) { //判断相交 flag = 1; } return flag; } int main() { int n, m; //把正方形的点封装到pair内去 for (int i = 0; i < 4; i++) { cin >> x[1][i] >> y[1][i]; p[1][i] = PII (x[1][i], y[1][i]); } for (int i = 0; i < 4; i++) { cin >> x[2][i] >> y[2][i]; p[2][i] = PII (x[2][i], y[2][i]); } int flag = 0; if (ok (1, 2) || ok (2, 1) ) flag = 1; printf ("%s\n", flag ? "YES" : "NO"); return 0; }