要从服务器上下载PDF文件到本地,你可以使用Java的标准库和URL类来实现。以下是一个详细的代码示例:
import java.io.*;
import java.net.URL;
import java.net.URLConnection;
public class FileDownloader {
public static void main(String[] args) {
String fileUrl = "http://example.com/path/to/file.pdf";
String localFilePath = "/path/to/local/file.pdf";
try {
downloadFile(fileUrl, localFilePath);
System.out.println("File downloaded successfully.");
} catch (IOException e) {
System.err.println("Error downloading file: " + e.getMessage());
}
}
public static void downloadFile(String fileUrl, String localFilePath) throws IOException {
URL url = new URL(fileUrl);
URLConnection connection = url.openConnection();
try (InputStream inputStream = connection.getInputStream();
BufferedOutputStream outputStream = new BufferedOutputStream(new FileOutputStream(localFilePath))) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
}
}
}
在上述示例中,我们提供了要下载的PDF文件的URL和本地保存路径。
在downloadFile
方法中,我们首先创建一个URL对象,并通过URL对象打开连接(URLConnection)。
然后,我们使用输入流(inputStream)从连接中读取文件内容,并使用缓冲输出流(outputStream)将文件写入到本地文件路径。
通过逐块读取和写入数据,我们可以在整个文件下载完成后关闭输入流和输出流。
在主方法中,我们调用downloadFile
方法来执行文件下载操作,并在完成时输出成功消息。如果发生错误,我们将捕获并输出异常信息。
请注意,这是一个基本示例,没有包含身份验证或错误处理机制。在实际应用中,你可能需要添加适当的异常处理和错误处理逻辑,以及其他下载相关的功能,如进度监控等。
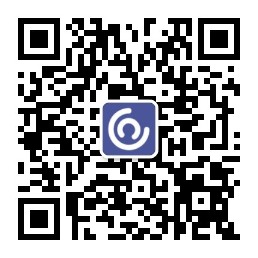