问题描述:
You are given coins of different denominations and a total amount of money amount. Write a function to compute the fewest number of coins that you need to make up that amount. If that amount of money cannot be made up by any combination of the coins, return -1
.
Example 1:
Input: coins =[1, 2, 5]
, amount =11
Output:3
Explanation: 11 = 5 + 5 + 1
Example 2:
Input: coins =[2]
, amount =3
Output: -1
Note:
You may assume that you have an infinite number of each kind of coin.
解题思路:
可以用dp来解
dp[i]代表的是前数为i是可用来换的最少的硬币。
因为题目中会给定coin的面值,所以我们需要对每一种面值进行尝试。
因为我设定的初始值为-1, 所以我在更新dp时
扫描二维码关注公众号,回复:
1638283 查看本文章
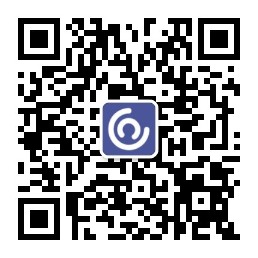
dp[i] = dp[i] == -1 ? dp[i-coins[j]]+1 : min(dp[i], dp[i-coins[j]]+1);
代码:
class Solution { public: int coinChange(vector<int>& coins, int amount) { vector<int> dp(amount+1, -1); sort(coins.begin(), coins.end()); dp[0] = 0; for(int i = 1; i <= amount; i++){ for(int j = 0; j < coins.size(); j++){ if(i - coins[j] > -1){ if(dp[i-coins[j]] != -1){ dp[i] = dp[i] == -1 ? dp[i-coins[j]]+1 : min(dp[i], dp[i-coins[j]]+1); } }else{ break; } } } return dp[amount]; } };