目录
一、Spring Cache介绍
Spring cache是一个框架,实现了基于注解的缓存功能,只需要简单地加一个注解,就能实现缓存
功能。Spring Cache提供了一层抽象,底层可以切换不同的cache实现。具体就是CacheManager
扫描二维码关注公众号,回复:
16681868 查看本文章
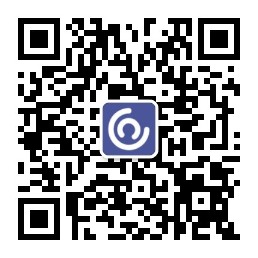
接口来统一不同的缓存技术。
CacheManager是Spring提供的各种缓存技术抽象接口。
针对不同的缓存技术需要实现不同的CacheManager:
CacheManager | 描述 |
EhcachecacheManager | 使用EhCache作为缓存技术 |
GuavaCacheManager | 使用Google的GuavaCache作为缓存技术 |
RedisCacheManager | 使用Redis作为缓存技术 |
1、Spring Cache常用注解
注解 | 说明 |
@EnableCaching | 开启缓存注解功能 |
@Cacheable | 在方法执行前spring先查看缓存中是否有数据,如果有数据,则直接返回缓存数据若没有数据,调用方法并将方法返回值放到缓存中 |
@CachePut | 将方法的返回值放到缓存中 |
@CacheEvict | 将一条或多条数据从缓存中删除 |
在spring boot项目中,使用缓存技术只需在项目中导入相关缓存技术的依赖包,并在启动类上使用
@EnableCaching开启缓存支持即可。
例如,使用Redis作为缓存技术,只需要导入Spring data Redis的maven坐标即可。
二、Spring Cache使用redis缓存步骤
1、添加依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
2、添加配置
spring
redis:
host: 172.17.2.94 #ip地址
port: 6379 #端口
password: root@123456 #redis密码
database: 0 #指定数据库
cache:
redis:
time-to-live: 1800000 #设置缓存过期时间,可选
3、使用注解
启动类加注解:@EnableCaching
@Slf4j
@SpringBootApplication
@EnableCaching
public class CacheDemoApplication {
public static void main(String[] args) {
SpringApplication.run(CacheDemoApplication.class,args);
log.info("项目启动成功...");
}
}
注解 @CachePut:将方法返回值放入缓存
/**
* CachePut:将方法返回值放入缓存
* value:缓存的名称,每个缓存名称下面可以有多个key
* key:缓存的key
*/
//@CachePut(value = "userCache",key = "#result.id")//result带表返回值
@CachePut(value = "userCache",key = "#user.id")//可以直接获取参数,参数名要保持一致
@PostMapping
public User save(User user){
userService.save(user);
return user;
}
key支持Spring的表达式语言,可以动态计算key值,通过#来获取,通常使用直接#+参数
result:表示返回值,可以通过获取返回值获取key
注解 @CacheEvict:清理指定缓存
/**
* CacheEvict:清理指定缓存
* value:缓存的名称,每个缓存名称下面可以有多个key
* key:缓存的key
*/
@CacheEvict(value = "userCache",key = "#p0")//p固定写法,0表示下表,第一个参数
//@CacheEvict(value = "userCache",key = "#root.args[0]")//固定写法,0表示下表,第一个参数
//@CacheEvict(value = "userCache",key = "#id")//直接获取参数
@DeleteMapping("/{id}")
public void delete(@PathVariable Long id){
userService.removeById(id);
}
//@CacheEvict(value = "userCache",key = "#p0.id")//p0第一个参数,id参数的属性
//@CacheEvict(value = "userCache",key = "#user.id")//直接获取参数属性
//@CacheEvict(value = "userCache",key = "#root.args[0].id")//获取第一个参数,id参数的属性
@CacheEvict(value = "userCache",key = "#result.id")//从返回值获取
@PutMapping
public User update(User user){
userService.updateById(user);
return user;
}
注解 @Cacheable:在方法执行前spring先查看缓存中是否有数据,如果有数据,则直接返回缓存数据若没有数据,调用方法并将方法返回值放到缓存中
/**
* Cacheable:在方法执行前spring先查看缓存中是否有数据,如果有数据,则直接返回缓存数据;若没有数据,调用方法并将方法返回值放到缓存中
* value:缓存的名称,每个缓存名称下面可以有多个key
* key:缓存的key
* condition:条件,满足条件时才缓存数据
* unless:满足条件则不缓存
*/
@Cacheable(value = "userCache",key = "#id",unless = "#result == null")
@GetMapping("/{id}")
public User getById(@PathVariable Long id){
User user = userService.getById(id);
return user;
}
@Cacheable(value = "userCache",key = "#user.id + '_' + #user.name")//动态拼接key
@GetMapping("/list")
public List<User> list(User user){
LambdaQueryWrapper<User> queryWrapper = new LambdaQueryWrapper<>();
queryWrapper.eq(user.getId() != null,User::getId,user.getId());
queryWrapper.eq(user.getName() != null,User::getName,user.getName());
List<User> list = userService.list(queryWrapper);
return list;
}