前言
WebView
作为控件,除了继承了View
的特性外,其自身功能也十分强大,还提供了网页加载、前进后退、网页缩放、搜索等功能。- 本节整理了
WebView
控件提供的常用API使用,主要划分为以下几部分内容。
一、 常用配置
WebView
常用配置API主要包含对 WebSettings
、 WebViewClient
、 WebChromeClient
配置操作。
1.1 获取WebSettings对象
WebSettings
是专门 对WebView
控件配置和管理的类。通过 getSettings()
方法获取该对象。
public WebSettings getSettings()
1.2 配置和获取WebViewClient对象
WebViewClient
是辅助WebView处理各种通知,请求等事件的一个类,按需求配置自定义的WebViewClient
对象。
public void setWebChromeClient(@Nullable WebChromeClient client)
@NonNull
public WebViewClient getWebViewClient()
1.3 配置和获取WebChromeClient对象
WebChromeClient
是辅助WebView
处理Javascript
的对话框、网站图标、网站title、加载进度等的类,按需求配置自定义的WebChromeClient
对象。
public void setWebChromeClient(@Nullable WebChromeClient client)
@Nullable
public WebChromeClient getWebChromeClient()
二 、资源加载
WebView
资源加载主要提供了loadUrl()
、loadData()
、loadDataWithBaseURL()
个方法。
2.1 loadUrl()
该方法用于加载指定的url,是日常开发中,最常用的资源加载API。
loadUrl(String url)
1.1.1 示例
- 页面加载
webview.loadUrl("https://www.baidu.com");
- 加载asset资源文件
webview.loadUrl("file://android_assets/demo.html");
- 加载设备本地资源文件
webview.loadUrl("/storage/emulated/0/demo/demo.html");
1.1.2 其他补充
此外官方还提供了重载方法,新增 additionalHttpHeaders
参数,支持在请求URL同时,带上Http请求头。
loadUrl(String url , Map<String,String> additionalHttpHeaders)
2.2 loadData()
loadData(String data,String mimeType,String encoding)
官方解释:
Loads the given data into this WebView using a ‘data’ scheme URL.
翻译过来就是:
加载Data URL scheme
格式的数据到WebView中。
该方法提供了三个参数:
data
表示要加载的内容。mimeType
表示数据类型,如:text/html
,image/jpeg
。encoding
表示字符编码格式,如:UTF-8
。
值得注意的点:
- 该方法来主要用于装载
Data URL scheme
格式的数据(RFC2397协议
),且不支持加载网络数据。 - 该方法不能加载图片,如果需要可以改用
loadDataWithBaseURL()
。
2.3 loadDataWithBaseURL()
loadDataWithBaseURL(String baseUrl, String data, String mimeType, String encoding, String historyUrl)
官方解释:
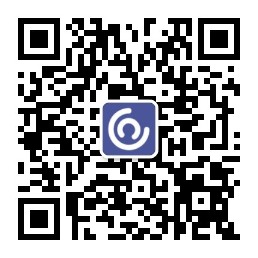
Loads the given data into this WebView, using baseUrl as the base URL for the content.
翻译过来:
将给定的数据加载到此WebView中,使用baseUrl
作为内容的基本URL。
与loadData()
方法比较,该方法新增了两个参数:
baseUrl
表示基础的网页,既用于解析相对URL,又用于应用JavaScript的同源策略。historyUrl
表示可用历史记录,可以为null值。
loadDataWithBaseURL()
比loadData()
更加强大,类似它的plus版,且支持图片加载。
三、 页面操作
WebView
中关于页面操作的API主要包含以下几块。
3.1 页面前进后退操作
首先,页面之所以能够前进后退,是因为WebView中缓存了一个前进后退请求历史记录列表,对应的类为WebBackForwardList
。
而为了便于存取,该列表中存放的元素为请求历史记录项的快照,对应的类为WebHistoryItem
,其中包含历史页面url、原始url、网页标题、网页图标等信息。
WebBackForwardList
作为列表用于存放WebHistoryItem
对象, 也相应提供了获取当前页面快照、当前页面快照下标、指定下标快照和列表长度方法。
通过历史列表的设计,页面前进后退操作就转化成对应快照的切换,判断能否前进后退则转化为是否有前进或者后台的历史记录。效果如下图:
3.1.1 判断页面能否前进
- 返回值为布尔型,
true
表示存在可前进的历史界面。
public boolean canGoForward()
3.1.2 页面前进
public void goForward()
3.1.3 判断页面能否后退
- 返回值为布尔型,
true
表示存在可后退的历史界面。
public boolean canGoBack()
3.1.4 页面后退
public void goBack()
3.1.5 判断页面能否前进或后退
- 参数
steps
表示前进或后退的步进,正数则为前进,负数为后退。 - 返回值为布尔型,
true
表示存在可前进或后退的历史界面。
public boolean canGoBackOrForward(int steps)
3.1.6 页面前进或后退
- 参数
steps
表示前进或后退的步进,正数则为前进,负数为后退。
public void goBackOrForward(int steps)
3.1.7 获取页面前进后退列表(即历史记录列表)
public WebBackForwardList copyBackForwardList()
3.2 页面缩放操作
- 在操作页面缩放前,需要设置WebView控件支持缩放。
webView.getSettings().setSupportZoom(true);
3.2.1 页面放大
- 返回值为布尔型,
true
表示界面放大成功。
public boolean zoomIn()
3.2.2 页面缩小
- 返回值为布尔型,
true
表示界面缩小成功。
public boolean zoomOut()
3.2.3 页面按指定比例缩放
- 参数
zoomFactor
表示界面需要缩放比例,范围为0.01
到100.0
。
public void zoomBy(float zoomFactor)
3.3 页面加载操作
3.3.1 重新加载页面
- 注意:当前页面的所有资源都会重新加载。
public void reload()
3.3.2 停止加载页面
public void stopLoading()
3.4 文件下载操作
Registers the interface to be used when content can not be handled by the rendering engine, and should be downloaded instead. This will replace the current handler.
- 当需要通过下载内容实现操作,而非渲染引擎渲染内容时,官方提供了一个
DownloadListener
接口供开发者配置实现。
public void setDownloadListener(@Nullable DownloadListener listener)
public interface DownloadListener {
public void onDownloadStart(String url, String userAgent,
String contentDisposition, String mimetype, long contentLength);
}
3.5 文本匹配查找操作
3.5.1 查找匹配文本并高亮显示
Finds all instances of find on the page and highlights them, asynchronously. Notifies any registered WebView.FindListener. Successive calls to this will cancel any pending searches.
public void findAllAsync(@NonNull String find)
- 异步查找页面上匹配数据并高亮显示。
- 连续调用该方法,将取消之前任何挂起的搜索。
3.5.2 清除查找匹配的高亮文本
public void clearMatches()
3.5.3 上下查找匹配文本
Highlights and scrolls to the next match found by findAllAsync, wrapping around page boundaries as necessary. Notifies any registered FindListener. If findAllAsync has not been called yet, or if clearMatches has been called since the last find operation, this function does nothing.
public void findNext(boolean forward)
- 该方法使用需要在调用
findAllAsync
之后。 - 调用后将高亮显示并滚动到下一匹配项,并通知任何已注册的
FindListener
。 - 在
clearMatches
之后调用,将不执行任何操作。
3.5.4 配置查找匹配监听器
Registers the listener to be notified as find-on-page operations progress. This will replace the current listener.
- 监听接口提供了页面查找进度回调,将替换原有查找匹配监听器。
public void setFindListener(@Nullable FindListener listener)
public interface FindListener {
public void onFindResultReceived(int activeMatchOrdinal, int numberOfMatches,
boolean isDoneCounting);
}
四、 生命周期
4.1 onPause()
Does a best-effort attempt to pause any processing that can be paused safely, such as animations and geolocation. Note that this call does not pause JavaScript. To pause JavaScript globally, use pauseTimers. To resume WebView, call onResume.
public void onPause()
- **页面被失去焦点或者被切换到后台,处于不可见状态时,**则会执行
onPause()
。 - 该方法尽可能暂停任何可以安全暂停的处理,例如动画和地理定位。
- 注意,这个调用不会暂停
JavaScript
。要全局暂停JavaScript
,请使用pauseTimers()
。 - 调用
onResume()
, 即可恢复WebView
,。
4.2 onResume()
- 从
onPause()
状态中恢复。
public void onResume()
4.3 pauseTimers()
Pauses all layout, parsing, and JavaScript timers for all WebViews. This is a global requests, not restricted to just this WebView. This can be useful if the application has been paused.
public void pauseTimers()
pauseTimers()
会暂停所有WebView
的布局显示、解析、延时,降低CPU功耗。- 当应用程序被切换到后台时,该方法不仅仅影响当前界面的
WebView
,而是全局应用的WebView
。
4.4 resumeTimers()
Resumes all layout, parsing, and JavaScript timers for all WebViews.This will resume dispatching all timers.
public void resumeTimers()
- 从
pauseTimers()
状态中恢复。 - 调用该方法将恢复所有
Webview
的布局、解析和JavaScript
定时器。
4.5 destroy()
Destroys the internal state of this WebView. This method should be called
after this WebView has been removed from the view system. No other methods may be called on this WebView after destroy.
public void destroy()
- 无论是官网还是代码注释,对该方法的解释均为销毁
WebView
的内部状态。 - 若在关闭了
Activity
时,WebView
的音乐或视频,还在播放,则必须销毁WebView
的内部状态。 - 调用
destroy()
方法前,需要先从父容器中移除WebView
。
rootLayout.removeView(webView);
webView.destroy();
- 调用
destroy()
方法后,该WebView
的其他方法均不能调用。
五、缓存清理
WebView
关于缓存清理的API主要包括网页访问、历史记录及表单数据缓存清理几块。
5.1 清除网页访问的缓存
- 由于内核缓存是全局的,因此这个方法清除的是整个应用程序页面访问缓存。
- 参数
includeDiskFiles
表示是否包含磁盘文件的缓存,如果为false
,则不包含,只清除RAM缓存。
public void clearCache(boolean includeDiskFiles)
#### 5.2 清除历史记录 - 只清除当前`WebView`访问的历史记录。 ``` public void clearHistory() ```
5.3 表单数据清除
- 该方法仅清除自动完成填充的表单数据,并不会清除WebView存储到本地的数据。
public void clearFormData()
六、 获取页面信息
WebView
中获取页面信息的API主要包含以下几块。
6.1 获取当前页URL
Gets the URL for the current page. This is not always the same as the URL passed to WebViewClient.onPageStarted because although the load for that URL has begun, the current page may not have changed.
public String getUrl()
- 值得注意的,通过
WebViewClient
的onPagStarted()
获取的URL,与getUrl()
获取到的不一定相同。因为尽管该URL已经开始加载,但当前页面可能还未改变。
6.2 获取当前页原始URL
Gets the original URL for the current page. Also, there may have been redirects resulting in a different URL to that originally requested.
public String getOriginalUrl()
- 考虑到页面重定向,该方法返回原始请求的URL。
6.3 获取当前网页标题
public String getTitle()
6.4 获取当前网页图标
public Bitmap getFavicon()
6.5 获取当前页SSL证书
public SslCertificate getCertificate()
6.6 获取HTML的高度
- 获取的是HTML原始高度,不包括缩放后的高度。
public int getContentHeight()
6.7 获取HTML的宽度
- 获取的是HTML原始宽度,不包括缩放后的宽度。
public int getContentWidth()