“掌握知识点的最好办法就是看官方注释 /官方示例.”
上面那句话是我自己说的….
欢迎关注我的 github, 提供各类技术点的Demo: https://github.com/YouCii/LearnApp
总览
常用的数据结构都是从 Collection 和 Map 接口发散的.
这两个接口在 java sdk 和 android sdk 里各有一份, android sdk 的实现类如下:
这里面的重复类也都是 java sdk 和 android sdk各有一份, android sdk 对 java sdk 进行了删减与扩展, 这篇文章只整理 android sdk 中的.
其中 Collection 的实现类里包括了平时常见的 List, Queue, Set 等.
List
List 接口的官方注释
A {@code List} is a collection which maintains an ordering for its elements. Every
element in the {@code List} has an index. Each element can thus be accessed by its
index, with the first index being zero. Normally, {@code List}s allow duplicate
elements, as compared to Sets, where elements have to be unique.
List是一个保持其元素顺序的集合. 每个List中的元素具有index索引, 每个元素都可以通过从0开始的index访问.
通常, List允许元素重复, 而Sets则要求元素必须唯一.
实现 List 接口中日常使用比较多的类有以下几个:
ArrayList, LinkedList(也同时实现了Queue), Vector, Stack, CopyOnWriteArrayList, VolatileSizeArrayList.
Queue
A collection designed for holding elements prior to processing.
Besides basic {@link java.util.Collection Collection} operations,
queues provide additional insertion, extraction, and inspection
operations. Each of these methods exists in two forms: one throws
an exception if the operation fails, the other returns a special
value (either <tt>null</tt> or <tt>false</tt>, depending on the
operation). The latter form of the insert operation is designed
specifically for use with capacity-restricted <tt>Queue</tt>
implementations; in most implementations, insert operations cannot
fail.
一种用于在处理前持有elements的集合。除了基本的Collection操作之外,
Queue提供了额外的插入、提取和检查操作。每个方法都有两种形式:一种
是操作失败时抛出异常, 另一种是返回model或者根据操作类型返回null以及false。
后一种插入操作形式是专门设计于Queue有容量限制的实现类, 因为在大多数实现类里,
插入操作不允许失败。
实现 Queue 接口的主要实现类有以下几个( 上方已提到的就不提了 ):
ArrayBlockingQueue, LinkedBlockingQueue, SynchronousQueue, DelayQueue, Deque相关
Set
A {@code Set} is a data structure which does not allow duplicate elements.
Set是一种不允许元素重复的数据结构.
主要实现类有: HashSet, LinkedHashSet, TreeSet, CopyOnWriteArraySet
Map
A {@code Map} is a data structure consisting of a set of keys and values
in which each key is mapped to a single value. The class of the objects
used as keys is declared when the {@code Map} is declared, as is the
class of the corresponding values.
A {@code Map} provides helper methods to iterate through all of the
keys contained in it, as well as various methods to access and update
the key/value pairs.
Map是由一组键/值组成的数据结构,其中每个键被映射到单个值。声明Map对象时, value相对应的
Key的类型也会一同声明.
Map提供帮助方法来遍历其中包含的所有键,以及各种方法来访问和更新键值对。
主要实现类有: HashMap, LinkedHashMap, TreeMap, LinkedHashTreeMap, ArrayMap, WeakHashMap, THashMap, ConcurrentHashMap, Hashtable.
其它类型
主要分析两个, SparseArray 和 Atomic.
SparseArray
Android 特有的数据类型.
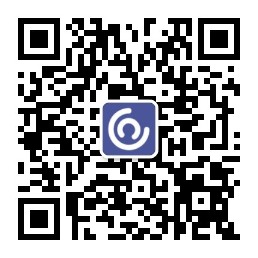
SparseArrays map integers to Objects. Unlike a normal array of Objects,
there can be gaps in the indices. It is intended to be more memory efficient
than using a HashMap to map Integers to Objects, both because it avoids
auto-boxing keys and its data structure doesn't rely on an extra entry object
for each mapping.
<p>Note that this container keeps its mappings in an array data structure,
using a binary search to find keys. The implementation is not intended to be appropriate for
data structures
that may contain large numbers of items. It is generally slower than a traditional
HashMap, since lookups require a binary search and adds and removes require inserting
and deleting entries in the array. For containers holding up to hundreds of items,
the performance difference is not significant, less than 50%.</p>
<p>To help with performance, the container includes an optimization when removing
keys: instead of compacting its array immediately, it leaves the removed entry marked
as deleted. The entry can then be re-used for the same key, or compacted later in
a single garbage collection step of all removed entries. This garbage collection will
need to be performed at any time the array needs to be grown or the the map size or
entry values are retrieved.</p>
<p>It is possible to iterate over the items in this container using
{@link #keyAt(int)} and {@link #valueAt(int)}. Iterating over the keys using
<code>keyAt(int)</code> with ascending values of the index will return the
keys in ascending order, or the values corresponding to the keys in ascending
order in the case of <code>valueAt(int)</code>.</p>
SparseArray将整数映射到对象。与普通的对象数组不同,SparseArray的索引有所不同, 这是为了比
HashMap<Integer,Objects>更加有内存效率,这既是因为它避免了作为Key的Integer的自动装箱,也因为它的数据结
构无需为每个键值对创建额外的entry对象(HashMap的内部类HashMapEntry).
请注意,这个容器使用一个"利用二分查找Key的数组"维护它的映射关系。该实现并不适合于可能包含大量items的
数据结构。它通常比传统的HashMap要慢,因为查找需要进行二分查找,并且添加和删除需要插入和删除数组中的条目。
对于容纳数百个项目的容器,性能差异并不显著,小于50%。
为了帮助性能,容器在删除键时包含了一个优化:它没有立即压缩它的数组,而是将被删除的条目标记为已删除。然后,
条目可以被重新用于相同的键,或者在所有删除条目的单个垃圾收集步骤中被压缩。这个垃圾收集需要在需要增加数组
的任何时候执行,或者检索映射的大小或入口值。
可以使用 keyAt(int)和 valueAt(int)来遍历这个容器中的条目。使用 keyAt(int)来
遍历键值,索引的提升值将按升序返回键值,或者在valueAt(int)的情况下以升序的方式返回键值。
Atomic
主要提供在多线程环境下无锁的进行原子操作;