原地址:https://www.cnblogs.com/wyy123/p/6078593.html
【学会安装redis】
从redis.io下载最新版redis-X.Y.Z.tar.gz后解压,然后进入redis-X.Y.Z文件夹后直接make即可,安装非常简单。
make成功后会在src文件夹下产生一些二进制可执行文件,包括redis-server、redis-cli等等:
代码如下:
$ find . -type f -executable
./redis-benchmark //用于进行redis性能测试的工具
./redis-check-dump //用于修复出问题的dump.rdb文件
./redis-cli //redis的客户端
./redis-server //redis的服务端
./redis-check-aof //用于修复出问题的AOF文件
./redis-sentinel //用于集群管理
【学会启动redis】
启动redis非常简单,直接./redis-server就可以启动服务端了,还可以用下面的方法指定要加载的配置文件:
./redis-server ../redis.conf
默认情况下,redis-server会以非daemon的方式来运行,且默认服务端口为6379。
【使用redis客户端】
//这样来启动redis客户端了
$ ./redis-cli
//用set指令来设置key、value
127.0.0.1:6379> set name "roc"
OK
//来获取name的值
127.0.0.1:6379> get name
"roc"
//通过客户端来关闭redis服务端
127.0.0.1:6379> shutdown
127.0.0.1:6379>
【redis数据结构 – 简介】
redis是一种高级的key:value存储系统,其中value支持五种数据类型:
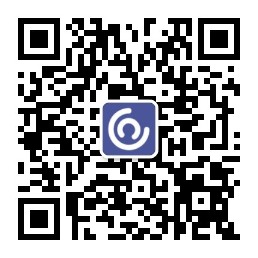
1.字符串(strings):由于INCR等指令本身就具有原子操作的特性,所以我们完全可以利用redis的INCR、INCRBY、DECR、DECRBY等指令来实现原子计数的效果
2.字符串列表(lists):lists的常用操作包括LPUSH、RPUSH、LRANGE等。我们可以用LPUSH在lists的左侧插入一个新元素,用RPUSH在lists的右侧插入一个新元素,用LRANGE命令从lists中指定一个范围来提取元素。
我们可以利用lists来实现一个消息队列,而且可以确保先后顺序,不必像MySQL那样还需要通过ORDER BY来进行排序。
利用LRANGE还可以很方便的实现分页的功能。
在博客系统中,每片博文的评论也可以存入一个单独的list中
3.字符串集合(sets)
4.有序字符串集合(sorted sets):很多时候,我们都将redis中的有序集合叫做zsets,这是因为在redis中,有序集合相关的操作指令都是以z开头的,比如zrange、zadd、zrevrange、zrangebyscore等等
5.哈希(hashes)
而关于key,有几个点要提醒大家:
1.key不要太长,尽量不要超过1024字节,这不仅消耗内存,而且会降低查找的效率;
2.key也不要太短,太短的话,key的可读性会降低;
3.在一个项目中,key最好使用统一的命名模式,例如user:10000:passwd。
【聊聊redis的事务处理】
众所周知,事务是指“一个完整的动作,要么全部执行,要么什么也没有做”。
在聊redis事务处理之前,要先和大家介绍四个redis指令,即MULTI、EXEC、DISCARD、WATCH。这四个指令构成了redis事务处理的基础。
1.MULTI用来组装一个事务;
2.EXEC用来执行一个事务;
3.DISCARD用来取消一个事务;
4.WATCH用来监视一些key,一旦这些key在事务执行之前被改变,则取消事务的执行。
redis存在的好处就在于无需频繁的访问数据库,当一些数据不会发生变化时可以直接添加到redis中,比如聊天一些历史记录等,类似于缓存,这样大大的提高了性能,建议在使用redis最好结合着数据库一起使用。
Redis使用示例:
int user_id = 2;//用户id String user_name;//用户名 if(jedis.hexists("user"+user_id, "user_name")){//判断jedis中是否存在"user"+user_id哈希表并且value值为user_name user_name =jedis.hget("user"+user_id, "user_name");//如果存在直接获取然后登陆 System.out.println("欢迎来到Redis登陆: "+user_name); }else{//如果不存在,则需要查询数据库判断用户名密码是否正确,如果存在则登陆,并且将其信息保存至redis String sql = "select user_name from user_t where id = "+user_id+""; try { ResultSet rs = conn.createStatement().executeQuery(sql); if(rs.next()){ jedis.hset("user"+user_id, "user_name", rs.getString("user_name"));//保存至redis中 System.out.println("欢迎来到MySql登陆: "+rs.getString("user_name")); }else{ System.out.println("欢迎 注册"); } } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); }
Redis工具类:
/** * */ package com.ai.toptea.basic.util; import java.util.Arrays; import java.util.HashMap; import java.util.HashSet; import java.util.List; import java.util.Map; import java.util.Set; import org.apache.commons.logging.Log; import org.apache.commons.logging.LogFactory; import org.apache.commons.pool2.impl.GenericObjectPoolConfig; import com.ai.toptea.basic.spring.IReloadablePropertyBean; import redis.clients.jedis.Jedis; import redis.clients.jedis.JedisPool; import redis.clients.jedis.JedisSentinelPool; import redis.clients.jedis.Pipeline; import redis.clients.jedis.Protocol; import redis.clients.jedis.SortingParams; import redis.clients.jedis.exceptions.JedisConnectionException; import redis.clients.util.Pool; /** * * redis链接类 * * @author <a href="mailto:[email protected]">FluteD</a> * @version 2014-4-10 */ public class RedisUtil implements IReloadablePropertyBean { public static final int DB_TEMP = 0; public static final int DB_COMMON = 3; public static final int DB_SYSMGR = 4; public static final int DB_RESM = 5; public static final int DB_ITSM = 6; public static final int DB_BSM = 7; public static final int DB_KBM = 8; public static final int DB_CTLM = 9; public static final int DB_CCP = 10; // 所有的DB索引 private static Map<String, String> DB = new HashMap<String, String>(); private static Log log = LogFactory.getLog(RedisUtil.class); protected static Set<String> sentinels = new HashSet<String>(); private static String sentinelsAddrs = ""; private static String masterName = "topteaRedisMaster"; private static String masterPass = ""; /** * key="master_dbnum/slave_dbnum",value=JedisSentinelPool/JedisPool */ private static Map<String, Pool<Jedis>> jedisPool = new HashMap<String, Pool<Jedis>>();// 连接池 public static void init() { sentinels.clear(); sentinels.addAll(Arrays.asList(sentinelsAddrs.split(","))); jedisPool.clear(); DB.put("" + DB_TEMP, "0"); DB.put("" + DB_COMMON, "3"); DB.put("" + DB_SYSMGR, "4"); DB.put("" + DB_RESM, "5"); DB.put("" + DB_ITSM, "6"); DB.put("" + DB_BSM, "7"); DB.put("" + DB_KBM, "8"); DB.put("" + DB_CTLM, "9"); } /** * 建立连接池 */ private static synchronized JedisSentinelPool initMasterPool(int dbIndex) { if (sentinels.size() == 0) { init(); } JedisSentinelPool jedisSentinelPool = getMasterPool(dbIndex); if (jedisSentinelPool != null) return jedisSentinelPool; if (DB.containsKey("" + dbIndex)) { jedisSentinelPool = new JedisSentinelPool(masterName, sentinels, new GenericObjectPoolConfig(), Protocol.DEFAULT_TIMEOUT, masterPass, dbIndex); } else { jedisSentinelPool = new JedisSentinelPool(masterName, sentinels, masterPass); dbIndex = 0; } jedisPool.put("master_" + dbIndex, jedisSentinelPool); return jedisSentinelPool; } /** * 建立连接池 */ private static JedisPool initSlavePool() { JedisPool jedisSlavePool = getSlavePool(); if (jedisSlavePool != null) { try { Jedis slave = jedisSlavePool.getResource(); slave.close(); return jedisSlavePool; } catch (JedisConnectionException e) { jedisSlavePool = null; log.error(e.getMessage(),e); } // return jedisSlavePool; } for (String sentinel : sentinels) { List<String> sentinelAddr = Arrays.asList(sentinel.split(":")); try { Jedis jedis = new Jedis(sentinelAddr.get(0), Integer.parseInt(sentinelAddr.get(1))); List<Map<String, String>> salves = jedis.sentinelSlaves(masterName); if (salves == null || salves.size() == 0) { continue; } int randomIndex = 0; while (randomIndex < salves.size()) { Map<String, String> salvesAttr = salves.get(randomIndex); // 当slave处于下线状态或者连接不上时,flags字段里会有s_down,disconnected字样 String flages = salvesAttr.get("flags"); if (flages.indexOf("s_down") != -1 || flages.indexOf("disconnected") != -1) { randomIndex++; continue; } jedisSlavePool = new JedisPool(new GenericObjectPoolConfig(), salvesAttr.get("ip"), Integer.parseInt(salvesAttr.get("port")), Protocol.DEFAULT_TIMEOUT, masterPass); break; } jedis.close(); break; } catch (JedisConnectionException e) { // log.info("Cannot connect to sentinel running @ " + sentinel + ". Trying next one."); } } if (jedisSlavePool == null) { try { Jedis master = getMasterResource(DB_TEMP); jedisSlavePool = new JedisPool(new GenericObjectPoolConfig(), master.getClient().getHost(), master.getClient().getPort(), Protocol.DEFAULT_TIMEOUT, masterPass); returnMasterResource(master); } catch (Exception e) { log.error(e.getMessage(),e); } } jedisPool.put("slave", jedisSlavePool); return jedisSlavePool; } /** * 获取jedis资源,调用此方法后,使用完毕,需要调用returnMasterResource方法 * * @return * @throws Exception */ public static Jedis getMasterResource(int dbIndex) throws Exception { JedisSentinelPool jedisSentinelPool = initMasterPool(dbIndex); Jedis jedis = null; int index = 0; while (index < 6) { try { jedis = jedisSentinelPool.getResource(); int dbi = jedis.getDB().intValue(); if (dbi != dbIndex) jedis.select(dbIndex); break; } catch (JedisConnectionException e) { log.error(e.getMessage(),e); log.info("Cannot connect to redis master. Sleeping 5000ms and retrying.."); try { Thread.sleep(5000); } catch (InterruptedException e1) { log.error(e.getMessage(),e); } } index++; if (index >= 6) { log.info("Cannot connect to redis master."); throw new Exception("Cannot connect to redis master in 30s"); } } return jedis; } /** * 获取jedis slave资源,调用此方法后,使用完毕,需要调用returnSlaveResource方法 * * @return */ public static Jedis getSlaveResource() { JedisPool jedisSlavePool = initSlavePool(); Jedis slave = null; try { slave = jedisSlavePool.getResource(); } catch (JedisConnectionException e) { } return slave; } public static Jedis getSlaveResource(int dbIndex) { Jedis jedis = getSlaveResource(); if (jedis != null) { jedis.select(dbIndex); } return jedis; } public static Jedis getJedisSlave(int dbIndex) { Jedis jedis = getSlaveResource(); if (jedis != null) { jedis.select(dbIndex); } return jedis; } /** * * @param dbIndex * @param key * @param value * @throws Exception */ public static String set(int dbIndex, String key, String value) throws Exception { Jedis jedis = null; String ret = ""; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.set(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } public static String set(int dbIndex, byte[] key, byte[] value) throws Exception { Jedis jedis = null; String ret = ""; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.set(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 链表数组,左拼接 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long lpush(int dbIndex, String key, String... value) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lpush(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 链表数组,左拼接 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long lpush(int dbIndex, byte[] key, byte[]... value) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lpush(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 链表数组,左拼接 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long lpushx(int dbIndex, byte[] key, byte[]... value) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lpushx(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 链表数组,左拼接 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long lpushx(int dbIndex, String key, String value) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lpushx(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 链表数组,右拼接 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long rpush(int dbIndex, String key, String... value) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.rpush(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 链表数组,右拼接 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long rpushx(int dbIndex, String key, String... value) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.rpushx(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 链表数组,右拼接 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long rpush(int dbIndex, byte[] key, byte[]... value) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.rpush(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 链表数组,右拼接 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long rpushx(int dbIndex, byte[] key, byte[]... value) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.rpushx(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 从左侧开始获取链表数据 * * @param dbIndex * @param key * @param start * @param end * @return * @throws Exception */ public static List<byte[]> lrange(int dbIndex, byte[] key, long start, long end) { Jedis jedis = null; List<byte[]> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lrange(key, start, end); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 从左侧开始获取链表数据 * * @param dbIndex * @param key * @param start * @param end * @return * @throws Exception */ public static List<String> lrange(int dbIndex, String key, long start, long end) { Jedis jedis = null; List<String> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lrange(key, start, end); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 从右侧删除数据并返回 * * @param dbIndex * @param key * @return * @throws Exception */ public static String rpop(int dbIndex, String key) { Jedis jedis = null; String ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.rpop(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 从右侧删除数据并返回 * * @param dbIndex * @param key * @return * @throws Exception */ public static byte[] rpop(int dbIndex, byte[] key) { Jedis jedis = null; byte[] ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.rpop(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 从左侧删除数据并返回 * * @param dbIndex * @param key * @return * @throws Exception */ public static String lpop(int dbIndex, String key) { Jedis jedis = null; String ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lpop(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 从左侧删除数据并返回 * * @param dbIndex * @param key * @return * @throws Exception */ public static byte[] lpop(int dbIndex, byte[] key) { Jedis jedis = null; byte[] ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lpop(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 返回链表长度 * @param dbIndex * @param key * @return */ public static long llen(int dbIndex, byte[] key) { Jedis jedis = null; long ret = 0; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.llen(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 返回链表长度 * @param dbIndex * @param key * @return */ public static long llen(int dbIndex, String key) { Jedis jedis = null; long ret = 0; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.llen(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 删除指定数量(count)的指定项(value) * * @param dbIndex * @param key * @param count * @param value * @return * @throws Exception */ public static Long lrem(int dbIndex, String key, long count, String value) { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lrem(key, count, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 删除指定数量(count)的指定项(value) * * @param dbIndex * @param key * @param count * @param value * @return * @throws Exception */ public static Long lrem(int dbIndex, byte[] key, long count, byte[] value) { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lrem(key, count, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 更新制定位置(index)的值 * * @param dbIndex * @param key * @param index * @param value * @return * @throws Exception */ public static String lset(int dbIndex, byte[] key, long index, byte[] value) throws Exception { Jedis jedis = null; String ret = null; int retryCount = 0; while (retryCount < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lset(key, index, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } retryCount++; } returnMasterResource(jedis); if (retryCount >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 更新制定位置(index)的值 * * @param dbIndex * @param key * @param index * @param value * @return * @throws Exception */ public static String lset(int dbIndex, String key, long index, String value) throws Exception { Jedis jedis = null; String ret = null; int retryCount = 0; while (retryCount < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.lset(key, index, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } retryCount++; } returnMasterResource(jedis); if (retryCount >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long append(int dbIndex, String key, String value) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.append(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long append(int dbIndex, byte[] key, byte[] value) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.append(key, value); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 删除一个键值 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long del(int dbIndex, byte[] key) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.del(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 删除一个键值 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long del(int dbIndex, byte[]... key) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.del(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 删除一个键值 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long del(int dbIndex, String key) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.del(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 删除一个键值 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long del(int dbIndex, String... key) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.del(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 删除一个键值 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long hdel(int dbIndex, String key, String... fields) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.hdel(key, fields); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 删除一个键值 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long hdel(int dbIndex, byte[] key, byte[]... fields) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.hdel(key, fields); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 设置过期时间 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long expire(int dbIndex, String key, int seconds) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.expire(key, seconds); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 设置过期时间 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long expireAt(int dbIndex, String key, long unixTime) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.expireAt(key, unixTime); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 设置过期时间 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long expire(int dbIndex, byte[] key, int seconds) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.expire(key, seconds); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 设置过期时间 * * @param dbIndex * @param key * @param value * @return * @throws Exception */ public static Long expireAt(int dbIndex, byte[] key, long unixTime) throws Exception { Jedis jedis = null; Long ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.expireAt(key, unixTime); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * 获取一个键值 * * @param dbIndex * @param key * @return */ public static String get(int dbIndex, String key) { Jedis jedis = null; String ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.get(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnSlaveResource(jedis); return ret; } public static String get(int dbIndex, String key, long startOffset, long endOffset) { Jedis jedis = null; String ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.getrange(key, startOffset, endOffset); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnSlaveResource(jedis); return ret; } public static String hget(int dbIndex, String key, String field) { Jedis jedis = null; String ret = ""; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.hget(key, field); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static byte[] hget(int dbIndex, byte[] key, byte[] field) { Jedis jedis = null; byte[] ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.hget(key, field); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static Map<byte[], byte[]> hgetAll(int dbIndex, byte[] key) { Jedis jedis = null; Map<byte[], byte[]> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.hgetAll(key); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static Map<String, String> hgetAll(int dbIndex, String key) { Jedis jedis = null; Map<String, String> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.hgetAll(key); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static boolean hexists(int dbIndex, String key, String field) { Jedis jedis = null; boolean ret = false; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.hexists(key, field); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static boolean hexists(int dbIndex, byte[] key, byte[] field) { Jedis jedis = null; boolean ret = false; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.hexists(key, field); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static boolean exists(int dbIndex, String key) { Jedis jedis = null; boolean ret = false; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.exists(key); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static boolean exists(int dbIndex, byte[] key) { Jedis jedis = null; boolean ret = false; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.exists(key); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static Set<String> keys(int dbIndex, String pattern) { Jedis jedis = null; Set<String> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.keys(pattern); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnSlaveResource(jedis); return ret; } public static Set<byte[]> keys(int dbIndex, byte[] pattern) { Jedis jedis = null; Set<byte[]> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.keys(pattern); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnSlaveResource(jedis); return ret; } /** * 获取一个键值 * * @param dbIndex * @param key * @return */ public static byte[] get(int dbIndex, byte[] key) { Jedis jedis = null; byte[] ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.get(key); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnSlaveResource(jedis); return ret; } public static byte[] get(int dbIndex, byte[] key, long startOffset, long endOffset) { Jedis jedis = null; byte[] ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.getrange(key, startOffset, endOffset); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnSlaveResource(jedis); return ret; } public static List<String> mget(int dbIndex, String... keys) { Jedis jedis = null; List<String> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.mget(keys); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnSlaveResource(jedis); return ret; } public static List<byte[]> mget(int dbIndex, byte[]... keys) { Jedis jedis = null; List<byte[]> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.mget(keys); break; } catch (Exception e) { log.info(e.getMessage()); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnSlaveResource(jedis); return ret; } /** * map 结构 * * @param dbIndex * @param key * @param field * @param value * @return * @throws Exception */ public static long hset(int dbIndex, String key, String field, String value) throws Exception { Jedis jedis = null; long ret = 0; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.hset(key, field, value); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } /** * map 结构 * * @param dbIndex * @param key * @param field * @param value * @return * @throws Exception */ public static long hset(int dbIndex, byte[] key, byte[] field, byte[] value) throws Exception { Jedis jedis = null; long ret = 0; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.hset(key, field, value); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); if (index >= 3) { throw new Exception("Cannot save data to redis."); } return ret; } public static long sort(int dbIndex, String key, String dstkey) { Jedis jedis = null; long ret = 0; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.sort(key, dstkey); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static long sort(int dbIndex, byte[] key, byte[] dstkey) { Jedis jedis = null; long ret = 0; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.sort(key, dstkey); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static List<String> sort(int dbIndex, String key) { Jedis jedis = null; List<String> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.sort(key); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static List<String> sort(int dbIndex, String key, SortingParams sp) { Jedis jedis = null; List<String> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.sort(key, sp); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static long sort(int dbIndex, String key, SortingParams sp, String dstkey) { Jedis jedis = null; long ret = 0; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.sort(key, sp, dstkey); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static long sort(int dbIndex, byte[] key, SortingParams sp, byte[] dstkey) { Jedis jedis = null; long ret = 0; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.sort(key, sp, dstkey); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static List<byte[]> sort(int dbIndex, byte[] key) { Jedis jedis = null; List<byte[]> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.sort(key); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static List<byte[]> sort(int dbIndex, byte[] key, SortingParams sp) { Jedis jedis = null; List<byte[]> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.sort(key, sp); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 执行脚本 * * @param dbIndex * @param script * @return */ public static Object eval(int dbIndex, String script, List<String> keys, List<String> args) { Jedis jedis = null; Object ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.eval(script, keys, args); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 执行脚本 * * @param dbIndex * @param script * @return */ public static Object eval(int dbIndex, String script) { Jedis jedis = null; Object ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.eval(script); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 执行脚本 * * @param dbIndex * @param scriptShaId * @return */ public static Object evalsha(int dbIndex, String scriptShaId, List<String> keys, List<String> args) { Jedis jedis = null; Object ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); if (jedis.scriptExists(scriptShaId)) ret = jedis.evalsha(scriptShaId, keys, args); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 执行脚本 * * @param dbIndex * @param scriptShaId * @return */ public static Object evalsha(int dbIndex, String scriptShaId) { Jedis jedis = null; Object ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); if (jedis.scriptExists(scriptShaId)) ret = jedis.evalsha(scriptShaId); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 注册脚本 * * @param script * @return 脚本 sha id */ public static String scriptLoad(int dbIndex, String script) { Jedis jedis = null; String ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); ret = jedis.scriptLoad(script); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 管道方式,批量获取键值对象 * * @param dbIndex * @param keys * @return */ public static List<Object> pipeGet(int dbIndex, String... keys) { Jedis jedis = null; List<Object> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); Pipeline pipe = jedis.pipelined(); for (String key : keys) { pipe.get(key); } ret = pipe.syncAndReturnAll(); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } public static List<Object> pipeGet(int dbIndex, List<String> keys) { if (keys == null) return null; return pipeGet(dbIndex, keys.toArray(new String[0])); } /** * 管道方式,批量获取键值对象 * * @param dbIndex * @param keys * @return */ public static List<Object> pipeGet(int dbIndex, byte[]... keys) { Jedis jedis = null; List<Object> ret = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); Pipeline pipe = jedis.pipelined(); for (byte[] key : keys) { pipe.get(key); } ret = pipe.syncAndReturnAll(); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); return ret; } /** * 管道方式删除键值对 * @param dbIndex * @param keysPattern 可以用前缀加*匹配多个,如:users*表示删除users开头的 * @return */ public static void pipeDel(int dbIndex, String keysPattern) { Jedis jedis = null; int index = 0; while (index < 3) { try { jedis = getJedisMaster(dbIndex); Set<String> keys = jedis.keys(keysPattern); Pipeline pipe = jedis.pipelined(); for (String key : keys) { pipe.del(key); } pipe.sync(); break; } catch (Exception e) { log.error(e.getMessage(),e); try { Thread.sleep(1000); } catch (InterruptedException e1) { log.error(e1.getMessage(),e1); } } index++; } returnMasterResource(jedis); } /** * 获取一个指定数据库的Jedis * * @param dbIndex * 数据库编号 * @return * @throws Exception */ public static Jedis getJedisMaster(int dbIndex) throws Exception { Jedis jedis = getMasterResource(dbIndex); return jedis; } public static Jedis getCommonJedisMaster() { Jedis jedis = null; try { jedis = getJedisMaster(DB_COMMON); } catch (Exception e) { log.error(e.getMessage(),e); } return jedis; } public static Jedis getSysmgrJedisMaster() { Jedis jedis = null; try { jedis = getJedisMaster(DB_SYSMGR); } catch (Exception e) { log.error(e.getMessage(),e); } return jedis; } public static Jedis getItsmJedisMaster() { Jedis jedis = null; try { jedis = getJedisMaster(DB_ITSM); } catch (Exception e) { log.error(e.getMessage(),e); } return jedis; } public static Jedis getResmJedisMaster() { Jedis jedis = null; try { jedis = getJedisMaster(DB_RESM); } catch (Exception e) { log.error(e.getMessage(),e); } return jedis; } public static Jedis getKbmJedisMaster() { Jedis jedis = null; try { jedis = getJedisMaster(DB_KBM); } catch (Exception e) { log.error(e.getMessage(),e); } return jedis; } public static Jedis getBsmJedisMaster() { Jedis jedis = null; try { jedis = getJedisMaster(DB_BSM); } catch (Exception e) { log.error(e.getMessage(),e); } return jedis; } /** * 归还jedis资源 * * @param jedis */ public static void returnMasterResource(Jedis jedis) { if (jedis == null) return; jedis.close(); // Long db = jedis.getDB(); // // JedisSentinelPool jedisSentinelPool = getMasterPool(db.intValue()); // // if (jedisSentinelPool == null) // return; // jedisSentinelPool.returnResource(jedis); } /** * 归还jedis slave资源 * * @param jedis */ public static void returnSlaveResource(Jedis jedis) { if (jedis == null) return; jedis.close(); // JedisPool jedisSlavePool = getSlavePool(); // // if (jedisSlavePool == null) // return; // jedisSlavePool.returnResource(jedis); } /** * @deprecated 对于我们的环境,连接池建立后一般不会销毁 */ public static void destroyMasterPool(int dbIndex) { JedisSentinelPool jedisSentinelPool = getMasterPool(dbIndex); if (jedisSentinelPool == null) return; jedisSentinelPool.destroy(); } private static JedisSentinelPool getMasterPool(int dbIndex) { JedisSentinelPool jedisSentinelPool = null; if (!DB.containsKey("" + dbIndex)) dbIndex = DB_TEMP; if (!jedisPool.containsKey("master_" + dbIndex)) return null; jedisSentinelPool = (JedisSentinelPool) jedisPool.get("master_" + dbIndex); return jedisSentinelPool; } private static JedisPool getSlavePool() { JedisPool jedisSlavePool = null; jedisSlavePool = (JedisPool) jedisPool.get("slave"); return jedisSlavePool; } /** * @param sentinelsAddrs * the sentinelsAddrs to set */ public static void setSentinelsAddrs(String sentinelsAddrs) { RedisUtil.sentinelsAddrs = sentinelsAddrs; } /** * @param masterPass * the masterPass to set */ public static void setMasterPass(String masterPass) { RedisUtil.masterPass = masterPass; } /** * @param masterName * the masterName to set */ public static void setMasterName(String masterName) { RedisUtil.masterName = masterName; } /* * (non-Javadoc) * * @see com.ai.toptea.basic.spring.IReloadablePropertyBean#beforePropertyReload(java.lang.String, java.lang.String) */ @Override public void beforePropertyReload(String propertyName, String newValue) { } /* * (non-Javadoc) * * @see com.ai.toptea.basic.spring.IReloadablePropertyBean#afterPropertyReload(java.lang.String, java.lang.String) */ @Override public void afterPropertyReload(String propertyName, String newValue) { // 没有变更直接返回 if ("sentinelsAddrs".equals(propertyName) && RedisUtil.sentinelsAddrs.equals(newValue)) return; if ("masterPass".equals(propertyName) && RedisUtil.masterPass.equals(newValue)) return; if ("masterName".equals(propertyName) && RedisUtil.masterName.equals(newValue)) return; init(); } }