模块开发之antd(Ant Design of React)使用(四)
前言
antd是Ant Design of React缩写.
专门为React开发的ant Design,由阿里平台开发并分享.开发和服务于企业级后台产品.
特性
- 提炼自企业级中后台产品的交互语言和视觉风格。
- 开箱即用的高质量 React 组件。
- 使用 TypeScript 构建,提供完整的类型定义文件。
- 全链路开发和设计工具体系。
是一款企业级的UI组件.没有其它特别的语法和概念.只需要简单配置即可使用.
初看
import React, { Component } from 'react';
import logo from './logo.svg';
import './App.css';
import { Button } from 'antd';
class App extends Component {
render() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<h1 className="App-title">Welcome to React</h1>
</header>
<p className="App-intro">
To get started, edit <code>src/App.js</code> and save to reload.
</p>
<Button type="primary" type="primary">Button</Button>
</div>
);
}
}
export default App;
引入import React, { Component } from 'react';
和import { Button } from 'antd';
再加上一些配置即可直接使用antd的UI库.
安装
命令
cnpm install antd -g
使用create-react-app脚手架
在create-react-app
脚手架中作用antd
组件.
安装脚本架脚本
npm install create-react-app -g
创建项目
创建demo
create-react-app demo
引入antd依赖
成功创建demo
项目,引入antd
依赖模块
在项目根目录中执行
cnpm install antd --save
按需加载
因为如果直接引入import { Button } from ‘antd’,虽然我们只使用Button但整个antd文件都会加载下来,在前端是不合适的,同时css文件也一同全部加载.
下面两种方式都可以只加载用到的组件。
组件按需加载
方式一、babel-plugin-import(推荐)
添加依赖
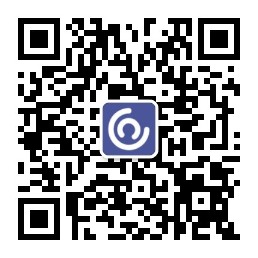
cnpm install babel-plugin-import --save
在.babelrc文件或babel-loader的配置里.
{
"plugins": [
["import", { "libraryName": "antd", "libraryDirectory": "es", "style": "css" }] // `style: true` 会加载 less 文件
]
}
然后只需从 antd 引入模块即可,无需单独引入样式。
import { Button} from 'antd';
,只会加载你Button
方式二、手动引入组件
import Buttonfrom 'antd/lib/button'; // 加载 JS
import 'antd/lib/button/style/css'; // 加载 CSS
样式按需加载
使用 react-app-rewired解决方案,此方案只适用于react-react-app脚本架生成的项目。
添加依赖
cnpm install react-app-rewired --save
cnpm install babel-plugin-import --save
package.json里的scrips脚本修改:
“scripts”: {
+ “start”: “react-app-rewired start”,
+ “build”: “react-app-rewired build”,
+ “test”: “react-app-rewired test –env=jsdom”,
}
在项目根目录下创建config-overrides.js,内容如下:
const { injectBabelPlugin } = require('react-app-rewired');
module.exports = function override(config, env) {
config = injectBabelPlugin(['import', { libraryName: 'antd', libraryDirectory: 'es', style: 'css' }], config);
return config;
};
直接引入整个antd模块,使用如下:
import { Button } from 'antd';
插件会帮你转换成 antd/lib/xxx 的写法,antd 组件的 js 和 css 代码都会按需加载
国际化
使用antd的LocaleProvide组件
如下即可
import {LocaleProvider} from 'antd'
import zhCN from 'antd/lib/locale-provider/zh_CN';
return (
<LocaleProvider locale={zhCN}>
<App />
</LocaleProvider>
);