在异常处理之中,出了我们自己主动捕获处理异常,还有一种处理方式,那就是抛出异常。抛出就是抛出给JVM,然后打印错误信息到控制台,中断程序的运行。
1.先来看看之前我们做过的不抛出异常
这里利用Person这个类,在对年龄进行赋值的时候,进行判断在一个合理范围,如果超过范围就提示年龄非法。之前我们没有学习异常是,通过打印语句来实现输出年龄非法的消息。
package exception;
public class Demo1_Exception {
public static void main(String[] args) {
Person p = new Person();
p.setAge(-18);
System.out.println(p.getAge());
}
}
class Person {
private String name;
private int age;
public Person() {
super();
}
public Person(String name, int age) {
super();
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
if(age>0 && age < 150) {
this.age = age;
} else {
System.out.println("年龄非法");
}
}
}
运行确实可以看到提示年龄非法,但是还是可以继续执行main方法的打印输出年龄语句。这里简单提一下,为什么提供的是-18,结果得到的是0,因为走了if判断语句,但是else语句块没有对age进行赋值操作,所以在Person这个类中age这个属性由于是整形,默认初始化值就是0.
2.抛出异常
现在我们想把这个年龄非法的通过异常抛出来,修改代码如下。
package exception;
public class Demo1_Exception {
public static void main(String[] args) throws Exception {
Person p = new Person();
p.setAge(-18);
System.out.println(p.getAge());
}
}
class Person {
private String name;
private int age;
public Person() {
super();
}
public Person(String name, int age) {
super();
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) throws Exception {
if(age>0 && age < 150) {
this.age = age;
} else {
throw new Exception("年龄非法");
}
}
}
注意,我们在else语句块通过throw关键字来抛出一个异常对象,这个异常默认是编译时异常。而且关注写法,在setAge()方法和main方法都进行了抛异常操作。但是方法里面抛异常使用的关键字是throws,而不是throw,关于throw我们下篇介绍。
3.编译时异常和运行时异常的处理区别
扫描二维码关注公众号,回复:
1888867 查看本文章
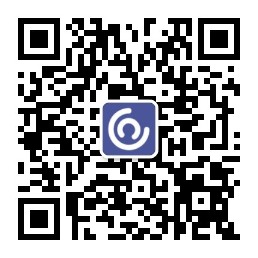
如果我们把else代码块中异常种类改成运行时异常,那么我们在该方法和main方法就可以不进行抛异常。
package exception;
public class Demo1_Exception {
public static void main(String[] args) {
Person p = new Person();
p.setAge(-18);
System.out.println(p.getAge());
}
}
class Person {
private String name;
private int age;
public Person() {
super();
}
public Person(String name, int age) {
super();
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
if(age>0 && age < 150) {
this.age = age;
} else {
throw new RuntimeException("年龄非法");
}
}
}
所以,这里总结下编译时异常和运行时异常抛出的处理区别:编译时异常抛出必须对其进行处理,而运行时异常不需要处理,当然添加上处理也没问题。