今天介绍最简单的Redis使用方法,注意细节和更多视频教程请关注下方二维码。
源码下载:https://download.csdn.net/download/cadn_jueying/10486599
一、引入Maven
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.ppl</groupId>
<artifactId>ppl-server</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>ppl-server</name>
<!-- Spring Boot -->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.4.RELEASE</version>
<relativePath />
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-eureka</artifactId>
</dependency>
<!-- Spring Boot Redis 依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
<!-- Spring Cloud -->
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>Dalston.SR4</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
</project>
二、application.yml中的配置
server:
port: 8901
#应用的名字
spring:
application:
name: ppl-server
#Redis配置
redis:
database: 0 # Redis数据库索引(默认为0)
host: 127.0.0.1 #Redis服务器地址
port: 6379 # Redis服务器连接端口
password: # Redis服务器连接密码(默认为空)
pool:
max-active: 20 # 连接池最大连接数(使用负值表示没有限制)
max-idle: 10 # 连接池中的最大空闲连接
max-wait: 1000 # 连接池最大阻塞等待时间(使用负值表示没有限制)
min-idle: 5 # 连接池中的最小空闲连接
timeout: 0 # 连接超时时间(毫秒)
eureka:
client:
serviceUrl:
defaultZone: http://localhost:8888/eureka/
三、新增RedisConfig.java配置文件
package com.ppl.config;
import org.apache.log4j.Logger;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Primary;
import org.springframework.data.redis.connection.jedis.JedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.serializer.Jackson2JsonRedisSerializer;
import com.fasterxml.jackson.annotation.PropertyAccessor;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import redis.clients.jedis.JedisPoolConfig;
/**
* Redis配置文件类
* @author ljl
*
*/
public class RedisConfig {
private static Logger logger = Logger.getLogger(RedisConfig.class);
@Bean
@ConfigurationProperties(prefix="spring.redis.pool")
public JedisPoolConfig getRedisConfig(){
JedisPoolConfig config = new JedisPoolConfig();
return config;
}
@Bean
@ConfigurationProperties(prefix="spring.redis")
public JedisConnectionFactory getConnectionFactory(){
JedisConnectionFactory factory = new JedisConnectionFactory();
JedisPoolConfig config = getRedisConfig();
factory.setPoolConfig(config);
logger.info("JedisConnectionFactory bean init success.");
return factory;
}
@Bean
@Primary
public RedisTemplate redisTemplate() {
JedisConnectionFactory jedisConnectionFactory = getConnectionFactory();
StringRedisTemplate template = new StringRedisTemplate(jedisConnectionFactory);
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jackson2JsonRedisSerializer.setObjectMapper(om);
template.setValueSerializer(jackson2JsonRedisSerializer);
template.afterPropertiesSet();
return template;
}
}
四、调用Redis
在其他要使用redis的类中,加入
@Autowiredprotected RedisTemplate redisTemplate;
使用示例:
package com.ppl.server.controller;
import java.util.concurrent.TimeUnit;
import org.apache.log4j.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ComputeController {
private final Logger logger = Logger.getLogger(getClass());
@Autowired
protected RedisTemplate redisTemplate;
@RequestMapping(value = "/add" ,method = RequestMethod.GET)
public Integer add(@RequestParam Integer a, @RequestParam Integer b) {
Integer r = a + b;
logger.info("/add, result:" + r);
return r;
}
@GetMapping("/hello")
public String sayHi(@RequestParam String name){
//从缓存中获取城市信息
String key = name;
ValueOperations<String, String> operations = redisTemplate.opsForValue();
//缓存存在
boolean hasKey = redisTemplate.hasKey(key);
if (hasKey) {
String userName = operations.get(key);
logger.info(" 从缓存中获取了姓名 >> " + userName);
return userName;
}
//缓存不存在,将数据存入缓存
operations.set(key, name, 10, TimeUnit.SECONDS);
logger.info("CityServiceImpl.findCityById() : 城市插入缓存 >> " +name);
return " Welcome: "+name;
}
}
每天坚持学习一小时
扫描二维码关注公众号,回复:
1934675 查看本文章
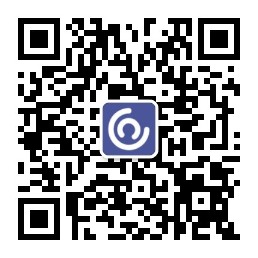