开始水一波博客
A. Choosing Ice Cream
题意就是n个冰淇淋,骰子有k个面,问你是否能在公平的概率下转几次骰子能确定买哪个冰淇淋。
举个例子,假设我只有一个冰淇淋,我不用转骰子直接就会买这个,所以转骰子的次数是0,如果我有4个冰淇淋,2个骰子面,我可以先把冰淇淋abcd分成两部分,ab一组,cd一组,这是等概率的,我先转一次骰子确定是选ab组还是cd组,然后再转一次就可以确定买哪个了。如果我有6个冰淇淋,12个面,我可以每一种冰淇淋贴2个面,转一次就可以确定了。个人理解是这种意思。
这个题读题猜题意猜了好久(捂脸==),题意猜对之后还是没过,最后发现是gcd是变化的,所以每循环一次就要重新算一遍gcd,1的情况特判一下就可以了。
代码:

1 #include<iostream> 2 #include<algorithm> 3 #include<cstring> 4 #include<iomanip> 5 #include<stdio.h> 6 #include<math.h> 7 #include<cstdlib> 8 #include<set> 9 #include<map> 10 #include<ctime> 11 #include<stack> 12 #include<queue> 13 #include<vector> 14 #include<set> 15 #define ll long long int 16 #define INF 0x7fffffff 17 #define LIT 0x3f3f3f3f 18 #define mod 1000000007 19 #define me(a,b) memset(a,b,sizeof(a)) 20 #define PI acos(-1.0) 21 #define ios ios::sync_with_stdio(0),cin.tie(0); 22 using namespace std; 23 const int maxn=1e5+5; 24 int main(){ 25 int t,n,k; 26 scanf("%d",&t); 27 while(t--){ 28 scanf("%d%d",&n,&k); 29 if(n==1){ 30 printf("0\n"); 31 continue; 32 } 33 int c=__gcd(n,k); 34 if(c==1){ 35 printf("unbounded\n"); 36 continue; 37 } 38 int ans=0; 39 while(c!=1){ 40 n/=c; 41 c=__gcd(n,k); 42 ans++; 43 } 44 if(n==1) 45 printf("%d\n",ans); 46 else 47 printf("unbounded\n"); 48 } 49 }
扫描二维码关注公众号,回复:
1946373 查看本文章
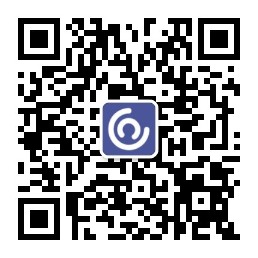
B. Failing Components
题意就是单向图,从起点开始找最短路,然后统计一下个数就可以。方向是从b到a,权值为s。
直接最短路跑迪杰斯特拉,一开始用数组版的没过,换了一个队列版的过了。
代码:

1 //B 2 #include<iostream> 3 #include<cstdio> 4 #include<cstring> 5 #include<cmath> 6 #include<cstdlib> 7 #include<queue> 8 #include<cstring> 9 #include<algorithm> 10 using namespace std; 11 typedef long long ll; 12 const int maxn=1e5+10; 13 const double eps=1e-7; 14 const int N=1e5+10; 15 const int INF=0x3f3f3f3f; 16 int head[N*2], nex[N*2], to[N*2], val[N*2], dis[N], vis[N], tot; 17 struct cmp{ 18 bool operator()(int a,int b) { 19 return dis[a]>dis[b]; 20 } 21 }; 22 priority_queue<int, vector<int>, cmp > Q; 23 void init() { 24 tot = 0; 25 while(!Q.empty()) Q.pop(); 26 memset(head, -1, sizeof(head)); 27 memset(dis, INF, sizeof(dis)); 28 memset(vis, 0, sizeof(vis)); 29 } 30 void addedge(int u, int v, int w) { 31 to[tot] = v; 32 nex[tot] = head[u]; 33 val[tot] = w; 34 head[u] = tot++; 35 } 36 void Dijkstra(int S) { 37 Q.push(S); 38 dis[S] = 0; 39 while(!Q.empty()) { 40 int u = Q.top(); 41 Q.pop(); 42 for(int i=head[u]; i!=-1; i=nex[i]) { 43 int v = to[i]; 44 if(!vis[v] && dis[u]+val[i] < dis[v]) { 45 dis[v] = dis[u]+val[i]; 46 Q.push(v); 47 } 48 } 49 } 50 } 51 int main(){ 52 int t; 53 scanf("%d",&t); 54 while(t--){ 55 init(); 56 int n,p,k; 57 scanf("%d%d%d",&n,&p,&k); 58 int h,l,val; 59 for(int i=0;i<p;i++){ 60 scanf("%d%d%d",&h,&l,&val); 61 addedge(l,h,val); 62 } 63 Dijkstra(k); 64 int num=0; 65 int maxx=-1; 66 for(int i=1;i<=n;i++) 67 { 68 if(dis[i]!=INF) 69 {num++;maxx=max(maxx,dis[i]);} 70 } 71 cout<<num<<" "<<maxx; 72 cout<<endl; 73 } 74 return 0; 75 }
F. Runway Planning
题意简直就是有毒,中间bb一堆都是没用的,主要的意思就是度数大于180度的就先减去180度,然后除以10,四舍五入的值就是答案。如果最后结果是0就输出18就可以,其他没了,看懂题意就是水题。
代码:

1 #include<iostream> 2 #include<cstdio> 3 #include<cstring> 4 #include<cmath> 5 #include<algorithm> 6 #include<cstdlib> 7 using namespace std; 8 typedef long long ll; 9 const int maxn=1e5+10; 10 int main(){ 11 int t; 12 scanf("%d",&t); 13 while(t--){ 14 int n,ans; 15 scanf("%d",&n); 16 if(n>=180)n-=180; 17 int cnt=n%10; 18 if(cnt>=5)ans=n/10+1; 19 else ans=n/10; 20 if(ans==0){ 21 if(cnt>=5)ans=1; 22 else ans=18; 23 } 24 printf("%02d\n",ans); 25 } 26 }
其他题并不想补题,因为不想看题目猜题意,去看别的东西了。
吐槽一下,这场比赛全是阅读理解题,我看不懂题意,以后要训练一下读题能力,英语等级考试水平和能不能读懂题一毛钱关系都没有。
Debug能力也要训练一下,因为自己Debug能力实在是差到变形。
受不了,为什么我队友还不理我,简直要哭死了(我错了,我错了,小声bb。。。)