STL容器适配器
STL提供了三种容器适配器:stack,queue,priority_queue。
适配器并不是第一类容器,因为它们并没有提供与元素的保存形式有关的真正数据结构实现,并且适配器不支持迭代器。
适配器的优点是:能够使程序员选择一种合适的底层数据结构。
这三个适配器类都提供了成员函数push和pop,能够在每个适配器数据结构中正确地插入和删除元素。
1、 stack适配器
stack类允许在底层数据结构的一端执行插入和删除操作(先入后出)。堆栈能够用任何序列容器实现:vector、list、deque。
下面的demo1创建了三个整数堆栈,使用标准库的每种序列容器作为底层数据结构来实现堆栈。默认情况下,堆栈是用deque实现的。
堆栈的操作包括:
1.将一个元素插入到堆栈顶部的push函数(通过调用底层容器的push_back函数实现)
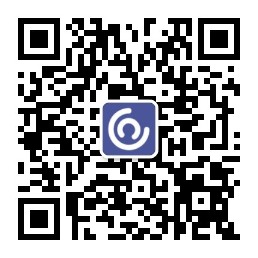
2.从堆栈的顶部删除一个元素的pop函数(通过调用底层元素的pop_back函数实现)
3.获取堆栈顶部元素引用的top函数(通过调用底层容器的back函数实现)
4.判断堆栈是否为空的empty函数(通过调用底层容器的empty函数实现)
5.获取堆栈元素数量的size函数(通过调用底层容器的size函数实现)
为了获得最佳性能,应使用vector类作为stack的底层容器
下面的demo演示了stack适配器类
- #include <iostream>
- #include <stack>
- #include <vector>
- #include <list>
- using namespace std;
- template <typename T> void pushElements(T &stackRef);
- template <typename T> void popElement(T &stackRef);
- int _tmain(int argc, _TCHAR* argv[])
- {
- stack<int> intDequeStack;
- stack<int,vector<int>> intVectorStack;
- stack<int,list<int>> intListStack;
- cout<<"pushing onto intDequeStack: ";
- pushElements(intDequeStack);
- cout<<"\npushing onto intVectorStack: ";
- pushElements(intVectorStack);
- cout<<"\npushing onto intListStack: ";
- pushElements(intListStack);
- cout<<endl<<endl;
- cout<<"poping from intDequeStack: ";
- popElement(intDequeStack);
- cout<<"\npoping from intVectorStack: ";
- popElement(intVectorStack);
- cout<<"\npoping from intListStack: ";
- popElement(intListStack);
- cout<<endl;
- system("pause");
- return 0;
- }
输出结果为:
2、 queue适配器
queue类允许在底层数据结构的末尾插入元素,也允许从前面插入元素(先入先出)。
队列能够用STL数据结构的list和deque实现,默认情况下是用deque实现的。
常见的queue操作:
1.在队列末尾插入元素的push函数(通过调用底层容器的push_back函数实现)
2.在队列前面删除元素的pop函数(通过调用底层容器的pop_back函数实现)
3.获取队列中第一个元素的引用的front函数(通过调用底层容器的front函数实现)
4.获取队列最后一个元素的引用的back(通过调用底层容器的back函数实现)
5.判断队列是否为空的empty函数(通过调用底层容器的empty函数实现)
6.获取队列元素数量的size函数(通过调用底层容器的size函数实现)
为了获得最佳性能,应使用deque类作为queue的底层容器
下面的denon2演示了queue适配器类
- #include <iostream>
- #include <queue>
- using namespace std;
- int _tmain(int argc, _TCHAR* argv[])
- {
- queue<double> values;
- //使用push函数,将元素添加到队列中
- values.push(3.2);
- values.push(9.8);
- values.push(5.4);
- cout<<"popping from values: ";
- //使用empty函数,判断队列是否为空
- while (!values.empty())
- {
- cout<<values.front()<<' '; //当队列还有其他元素时,使用front函数读取(但不删除)队列的第一个元素,用于输出
- values.pop(); //用pop函数,删除队列的第一个元素
- }
- cout<<endl;
- system("pause");
- return 0;
- }
输出结果为:
3、 priority_queue适配器
priority_queue类,能够按照有序的方式在底层数据结构中执行插入操作,也能从底层数据结构的前面执行删除操作。
priority_queue能够用STL的序列容器vector和deque实现。默认情况下使用vector作为底层容器的。当元素添加到priority_queue时,它们按优先级顺序插入。
这样,具有最高优先级的元素,就是从priority_queue中首先被删除的元素。通常这是利用堆排序来实现的。
堆排序总是将最大值(即优先级最高的元素)放在数据结构的前面。这种数据结构称为(heap)
默认情况下,元素的比较是通过比较器函数对象less<T>执行的。
priority_queue具有几个常见的操作:
1.根据priority_queue的优先级顺序在适当位置插入push函数(通过调用底层容器的push_back,然后使用堆排序为元素重新排序)
2.删除priority_queue的最高优先级元素的pop(删除堆顶元素之后通过调用底层容器的pop_back实现)
3.获取priority_queue的顶部元素引用的top函数(通过调用底层容器的front函数实现)
4.判断priority_queue是否为空的empty函数(通过调用底层容器的empty函数实现)
5.获取priority_queue元素数量的size函数(通过调用底层容器的size函数实现)
为了获取最佳性能,使用vector作为priority_queue的底层容器
下面demo3演示了priority_queue适配器类的用法
- #include <iostream>
- #include <queue>
- using namespace std;
- int _tmain(int argc, _TCHAR* argv[])
- {
- //实例化一个保存double值的priority_queue,并使用vector作为底层数据结构
- priority_queue<double> priorities;
- priorities.push(3.2);
- priorities.push(9.8);
- priorities.push(5.4);
- cout<<"Popping from priorities: ";
- while (!priorities.empty())
- {
- cout<<priorities.top()<<' '; //当priority_queue中还有其他元素时,使用priority_queue的top取得具有最高优先级的元素,用于输出
- priorities.pop(); //删除priority_queue中具有最高优先级的元素
- }
- cout<<endl;
- system("pause");
- return 0;
- }
输出结果为: