前言:学习 Service 需要明白3个问题,Service 是什么?Service 拿来干什么? Service 怎么使用?
服务是什么?
Service(服务) 是一种计算型组件,用于在后台执行一系列计算任务。由于Service 组件工作在后台,故用户无法直接感知到他的存在。Service 组件和Activity 组件略有不同,Activity组件只有一种运行模式,即Activity 处于启动状态,但Service 组件却有两种状态:启动状态和绑定状态。拿来干什么?
当Service 组件处于启动状态时,这个时候Service 内部可以做一些后台计算,并不需要和外界有直接交互。尽管Service 组件是用于执行后台计算的,但它本身是运行在主线程中的,因此耗时的后台计算仍然需要在单独的线程中去完成。当Service 组件处于绑定状态时,这个时候Service 内部同样可以进行后台计算,但是处于这种状态下的Service 可以很方便的和外界进行通信。Service 组件也可以停止,停止一个Service 需要灵活的采用 stopService 和unBinderService 两个方法才能完全的停止 Service 组件
服务并不是运行在一个独立的进程当中的,而是依赖于创建服务时所在的应用程序进程。当某个应用程序进程被杀掉时,所有依赖于该进程的服务也会停止运行。是Android中实现程序后台运行的解决方案,它非常适合用于去执行那些不需要和用户交互而且还要求长期运行的任务。
怎么用?服务的基本用法
定义一个服务首先看一下如何在项目中定义一个服务。新建一个ServiceTest项目,然后在这个项目中新增一个名为MyService的类,并让它继承自Service,完成后的代码如下所示:
public class MyService extends Service {
@Override
public IBinder onBind(Intent intent) {
return null;
}
}
onBind()方法方法是Service中唯一的一个抽象方法,所以必须要在子类里实现。这里暂不解释其用法,后面会详细解释既然是定义一个服务,自然应该在服务中去处理一些事情了,那处理事情的逻辑应该写在哪里呢?这时就可以重写Service中的另外一些方法了,如下所示:
public class MyService extends Service {
@Override
public IBinder onBind(Intent intent) {
return null;
}
@Override
public void onCreate() {
super.onCreate();
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
return super.onStartCommand(intent, flags, startId);
}
@Override
public void onDestroy() {
super.onDestroy();
}
}
这里我们又重写了onCreate()、onStartCommand()和onDestroy()这三个方法,它们是每个服务中最常用到的三个方法了。其中onCreate()方法会在服务创建的时候调用,onStartCommand()方法会在每次服务启动的时候调用,onDestroy()方法会在服务销毁的时候调用。通常情况下,如果我们希望服务一旦启动就立刻去执行某个动作,就可以将逻辑写在onStartCommand()方法里。而当服务销毁时,我们又应该在onDestroy()方法中去回收那些不再使用的资源。
onCreate()方法是在服务第一次创建的时候调用的,而onStartCommand()方法则在每次启动服务的时候都会调用。
启动和停止服务
startService()和stopService()方法都是定义在Context类中的,所以我们在活动里可以直接调用这两个方法。注意,这里完全是由活动来决定服务何时停止的,如果没有点击Stop Service按钮,服务就会一直处于运行状态。在MyService的任何一个位置调用stopSelf()方法就能让这个服务自已停止下来注意虽然每调用一次startService()方法,onStartCommand()就会执行一次,但实际上每个服务都只会存在一个实例。所以不管你调用了多少次startService()方法,只需调用一次stopService()或stopSelf()方法,服务就会停止下来了。
活动和服务进行通信(通过Binder)
通过onBind()方法实现步骤:Service中
1、 在服务中自定义一个继承Binder的类myBinder,并创建myBinder类的实例对象2、在myBinder类中定义书写和Activity 交互的方法
3、在IBinder中返回myBinder类的实例对象
Activity中
1、创建一个ServiceConnection的匿名类对象,里面重写了onServiceConnected()方法和onServiceDisconnected()方法,这两个方法分别会在活动与服务成功绑定以及解除绑定的时候调用。2、在onServiceConnected()方法中,我们又通过向下转型得到了myBinder的实例,通过myBinder对象调用myBinder中的任何public方法
3、绑定服务
4、解绑服务
比如说目前我们希望在MyService里提供一个下载功能,然后在活动中可以决定何时开始下载,以及随时查看下载进度。实现这个功能的思路是创建一个专门的Binder对象来对下载功能进行管理,修改MyService中的代码,如下所示:
public class MyService extends Service {
private DownloadBinder mBinder = new DownloadBinder();
class DownloadBinder extends Binder {
public void startDownload() {
Log.d("MyService", "startDownload executed");
}
public int getProgress() {
Log.d("MyService", "getProgress executed");
return 0;
}
}
@Override
public IBinder onBind(Intent intent) {
return mBinder;
}
……
}
这里我们新建了一个DownloadBinder类,并让它继承自Binder,然后在它的内部提供了开始下载以及查看下载进度的方法。当然这只是两个模拟方法,并没有实现真正的功能,我们在这两个方法中分别打印了一行日志。
接着,在MyService中创建了DownloadBinder的实例,然后在onBind()方法里返回了这个实例,这样MyService中的工作就全部完成了。
当一个活动和服务绑定了之后,就可以调用该服务里的Binder提供的方法了。修改MainActivity中的代码,如下所示:
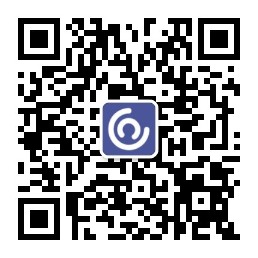
public class MainActivity extends Activity implements OnClickListener {
private Button startService;
private Button stopService;
private Button bindService;
private Button unbindService;
private MyService.DownloadBinder downloadBinder;
private ServiceConnection connection = new ServiceConnection() {
@Override
public void onServiceDisconnected(ComponentName name) {
}
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
downloadBinder = (MyService.DownloadBinder) service;
downloadBinder.startDownload();
downloadBinder.getProgress();
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
……
bindService = (Button) findViewById(R.id.bind_service);
unbindService = (Button) findViewById(R.id.unbind_service);
bindService.setOnClickListener(this);
unbindService.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
……
case R.id.bind_service:
Intent bindIntent = new Intent(this, MyService.class);
bindService(bindIntent, connection, BIND_AUTO_CREATE); // 绑定服务
break;
case R.id.unbind_service:
unbindService(connection); // 解绑服务
break;
default:
break;
}
}
}
这里我们首先创建了一个ServiceConnection的匿名类,在里面重写了onServiceConnected()方法和onServiceDisconnected()方法,这两个方法分别会在活动与服务成功绑定以及解除绑定的时候调用。
在onServiceConnected()方法中,我们又通过向下转型得到了DownloadBinder的实例,有了这个实例,活动和服务之间的关系就变得非常紧密了。现在我们可以在活动中根据具体的场景来调用DownloadBinder中的任何public方法,即实现了指挥服务干什么,服务就去干什么的功能。这里仍然只是做了个简单的测试,在onServiceConnected()方法中调用了DownloadBinder的startDownload()和getProgress()方法。
在活动中这里我们仍然是构建出了一个Intent对象,然后调用bindService()方法将MainActivity和MyService进行绑定。bindService()方法接收三个参数,第一个参数就是刚刚构建出的Intent对象,第二个参数是前面创建出的ServiceConnection的实例,第三个参数则是一个标志位,这里传入BIND_AUTO_CREATE表示在活动和服务进行绑定后自动创建服务。这会使得MyService中的onCreate()方法得到执行,但onStartCommand()方法不会执行。然后如果我们想解除活动和服务之间的绑定只要调用一下unbindService()方法就可以了,这也是Unbind Service按钮的点击事件里实现的功能。
另外需要注意,任何一个服务在整个应用程序范围内都是通用的,即MyService不仅可以和MainActivity绑定,还可以和任何一个其他的活动进行绑定,而且在绑定完成后它们都可以获取到相同的DownloadBinder实例。
LOG 日志如下:
服务的生命周期
一旦在项目的任何位置调用了Context的startService()方法,相应的服务就会启动起来,并回调onStartCommand()方法。如果这个服务之前还没有创建过,onCreate()方法会先于onStartCommand()方法执行。服务启动了之后会一直保持运行状态,直到stopService()或stopSelf()方法被调用。
注意虽然每调用一次startService()方法,onStartCommand()就会执行一次,但实际上每个服务都只会存在一个实例。所以不管你调用了多少次startService()方法,只需调用一次stopService()或stopSelf()方法,服务就会停止下来了。
另外,还可以调用Context的bindService()来获取一个服务的持久连接,这时就会回调服务中的onBind()方法。类似地,如果这个服务之前还没有创建过,onCreate()方法会先于onBind()方法执行。之后,调用方可以获取到onBind()方法里返回的IBinder对象的实例,这样就能自由地和服务进行通信了。只要调用方和服务之间的连接没有断开,服务就会一直保持运行状态。当调用了startService()方法后,又去调用stopService()方法,这时服务中的onDestroy()方法就会执行,表示服务已经销毁了。类似地,当调用了bindService()方法后,又去调用unbindService()方法,onDestroy()方法也会执行,这两种情况都很好理解。但是需要注意,我们是完全有可能对一个服务既调用了startService()方法,又调用了bindService()方法的,这种情况下该如何才能让服务销毁掉呢?根据Android系统的机制,一个服务只要被启动或者被绑定了之后,就会一直处于运行状态,必须要让以上两种条件同时不满足,服务才能被销毁。所以,这种情况下要同时调用unbindService() 和 stopService() 方法。
使用前台服务(防止因内存不足被系统回收,利用Notification(通知))
服务几乎都是在后台运行的,一直以来它都是默默地做着辛苦的工作。但是服务的系统优先级还是比较低的,当系统出现内存不足的情况时,就有可能会回收掉正在后台运行的服务。如果你希望服务可以一直保持运行状态,而不会由于系统内存不足的原因导致被回收,就可以考虑使用前台服务。前台服务和普通服务最大的区别就在于,它会一直有一个正在运行的图标在系统的状态栏显示,下拉状态栏后可以看到更加详细的信息,非常类似于通知的效果。当然有时候你也可能不仅仅是为了防止服务被回收掉才使用前台服务的,有些项目由于特殊的需求会要求必须使用前台服务,比如说音乐播放软件,它的服务在后台播放音乐的同时,还会在系统状态栏一直显示当前的音乐信息。
创建一个前台服务吧,其实并不复杂,修改MyService中的代码,如下所示:
public class MyService extends Service {
……
@Override
public void onCreate() {
super.onCreate();
Notification notification = new Notification(R.drawable.ic_launcher,
"Notification comes", System. currentTimeMillis());
Intent notificationIntent = new Intent(this, MainActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, notificationIntent, 0);
notification.setLatestEventInfo(this, "This is title", "This is content", pendingIntent);
startForeground(1, notification);
Log.d("MyService", "onCreate executed");
}
……
}
只不过这次在构建出Notification对象后并没有使用NotificationManager来将通知显示出来,而是调用了startForeground()方法。这个方法接收两个参数,第一个参数是通知的id,类似于notify()方法的第一个参数,第二个参数则是构建出的Notification对象。调用startForeground()方法后就会让MyService变成一个前台服务,并在系统状态栏显示出来。
使用IntentService
服务中的代码都是默认运行在主线程当中的,如果直接在服务里去处理一些耗时的逻辑,就很容易出现ANR(Application Not Responding)的情况。所以这个时候就需要用到Android多线程编程的技术了,我们应该在服务的每个具体的方法里开启一个子线程,然后在这里去处理那些耗时的逻辑。因此,一个比较标准的服务就可以写成如下形式:
public class MyService extends Service {
@Override
public IBinder onBind(Intent intent) {
return null;
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
new Thread(new Runnable() {
@Override
public void run() {
// 处理具体的逻辑
}
}).start();
return super.onStartCommand(intent, flags, startId);
}
}
但是,这种服务一旦启动之后,就会一直处于运行状态,必须调用stopService()或者stopSelf()方法才能让服务停止下来。所以,如果想要实现让一个服务在执行完毕后自动停止的功能,就可以这样写:
public class MyService extends Service {
@Override
public IBinder onBind(Intent intent) {
return null;
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
new Thread(new Runnable() {
@Override
public void run() {
// 处理具体的逻辑
stopSelf();
}
}).start();
return super.onStartCommand(intent, flags, startId);
}
}
虽说这种写法并不复杂,但难免有一些意外情况,比如忘记开启线程,或者忘记调用stopSelf()方法。为了可以简单地创建一个异步的、会自动停止的服务,Android专门提供了一个IntentService类,这个类就很好地解决了前面所提到的两种尴尬,新建一个MyIntentService类继承自IntentService,代码如下所示:
public class MyIntentService
extends IntentService
{
//必须要有默认的无参构造函数,并在里面调用父类有参的构造函数
public MyIntentService() {
super("MyIntentService");
}
/**
* Creates an IntentService. Invoked by your subclass's constructor.
*
* @param name Used to name the worker thread, important only for debugging.
*/
public MyIntentService(String name) {
super(name);
}
@Override
protected void onHandleIntent(@Nullable Intent intent) {
//打印当前线程的 id
Logger.t("MyIntentService")
.d("Thread id is" + Thread.currentThread()
.getId());
}
@Override
public void onDestroy() {
super.onDestroy();
Logger.t("MyIntentService")
.d("onDestroy");
}
}
这里首先是要提供一个无参的构造函数,并且必须在其内部调用父类的有参构造函数。然后要在子类中去实现onHandleIntent()这个抽象方法,在这个方法中可以去处理一些具体的逻辑,而且不用担心ANR的问题,因为这个方法已经是在子线程中运行的了。这里为了证实一下,我们在onHandleIntent()方法中打印了当前线程的id。另外根据IntentService的特性,这个服务在运行结束后应该是会自动停止的,所以我们又重写了onDestroy()方法,在这里也打印了一行日志,以证实服务是不是停止掉了。接下来修改activity_main.xml中的代码,加入一个用于启动MyIntentService这个服务的按钮,如下所示:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
……
<Button
android:id="@+id/start_intent_service"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Start IntentService" />
</LinearLayout>
然后修改TestActivity中的代码,如下所示: 然后修改TestActivity中的代码,如下所示:
public class TestActivity
extends BaseActivity<ActivityTestBinding>
implements View.OnClickListener
{
private MyService.DownloadBinder mDownloadBinder;
private ServiceConnection mConnection = new ServiceConnection(){
//活动与服务绑定成功时调用
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
mDownloadBinder = (MyService.DownloadBinder) service;
mDownloadBinder.startDownload();
mDownloadBinder.getProgress();
}
//活动与服务解除绑定时调用
@Override
public void onServiceDisconnected(ComponentName name) {
}
};
@Override
protected int getLayoutResId() {
return R.layout.activity_test;
}
@Override
public void init() {
bindingView.startServiceBtn.setOnClickListener(this);
bindingView.endServiceBtn.setOnClickListener(this);
bindingView.intentServiceBtn.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.start_service_btn:
Intent startIntent = new Intent(this, MyService.class);
bindService(startIntent,mConnection,BIND_AUTO_CREATE);//绑定服务
break;
case R.id.end_service_btn:
Intent endIntent = new Intent(this, MyService.class);
unbindService(mConnection);
break;
case R.id.intent_service_btn:
//打印当前线程的 id
Logger.t("TestActivity")
.d("Thread id is" + Thread.currentThread()
.getId());
Intent intentService = new Intent(this, MyIntentService.class);
startService(intentService);
break;
default:
break;
}
}
}
可以看到,我们在Start IntentService按钮的点击事件里面去启动MyIntentService这个服务,并在这里打印了一下主线程的id,稍后用于和IntentService进行比对。你会发现,其实IntentService的用法和普通的服务没什么两样。最后在AndroidManifest.xml里注册的,如下所示:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.servicetest"
android:versionCode="1"
android:versionName="1.0" >
……
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
……
<service android:name=".MyIntentService"></service>
</application>
</manifest>
现在重新运行一下程序,LOG 日志如下所示:
可以看到,不仅MyIntentService和MainActivity所在的线程id不一样,而且onDestroy()方法也得到了执行,说明MyIntentService在运行完毕后确实自动停止了。