/*1.上传商户信息文件*/
private boolean upLoadFile(MerInfoTemp info,MultipartFile merInfoFile){
logger.info("上传商户信息文件: MerInfoTempController.insertByFile()");
ReturnData returnData = new ReturnData();
returnData.setSuccess(true);
if (merInfoFile == null || merInfoFile.getSize() == 0) {
logger.info("商户信息上传失败");
return false;
}
String resourcePath = stParamService.selectValueByCode(ConfigPropertyConstant.MERINFO_FILE_PATH); //绝对路径
String merName = "";
if(info != null && StringUtils.isNotBlank(info.getMerName())){
merName = info.getMerName();
}
String acquirerCode = info.getAcquirerCode();
String acquirerName = "";
if(acquirerCode != null && StringUtils.isNotBlank(acquirerCode)){
AcquirerInfo acquirerInfo =acquirerInfoService.view(acquirerCode);
if(acquirerInfo != null && StringUtils.isNotBlank(acquirerInfo.getAcquirerName())){
acquirerName =acquirerInfo.getAcquirerName();
}
}
//文件格式校验
String extName = merInfoFile.getOriginalFilename().substring(merInfoFile.getOriginalFilename().lastIndexOf(".")).toLowerCase();
if(!(".xls".equals(extName)) && !(".xlsx".equals(extName))){
logger.info("上传文件格式不正确,上传文件格式要求后缀为.xls或.xlsx");
return false;
}
String newFileName = merName+"_"+acquirerName+"_"+SerialNo.getUNID()+merInfoFile.getOriginalFilename().substring(merInfoFile.getOriginalFilename().lastIndexOf("."));
/*String newFileName = SerialNo.getUNID()
+ merInfoFile.getOriginalFilename().substring(merInfoFile.getOriginalFilename().lastIndexOf("."));*/
logger.info("生成的相对路径:{}",newFileName);
String dir_relative_path = resourcePath + newFileName; //完整路径
logger.info("存放的完整路径为:{}",dir_relative_path);
try{
info.setMerInfoFileAddress(dir_relative_path); //merInfoTemp新增字段
merInfoFile.transferTo(new File(dir_relative_path));
}catch(Exception e){
logger.info("文件上传失败",e);
return false;
}
return true;
}
/*全部文件,压缩下载(支持批量)*/
@RequestMapping("/downLoadFiles")
@ResponseBody
public Object downLoadFiles(String pkids,HttpServletResponse response)throws IOException{
MerInfoTempExample example = new MerInfoTempExample();
MerInfoTempExample.Criteria criteria = example.createCriteria();
if(pkids != null && StringUtils.isNotBlank(pkids)){
String[] pkidArr=pkids.split(Constant.ID_SPLIT);
List<String> pkidList = Arrays.asList(pkidArr);
criteria.andPkidIn(pkidList);
}
List<String> versions = Lists.newArrayList();
versions.add("2.5");
versions.add("3.0");
criteria.andVersionIn(versions);
StUser user = (StUser)ContextHolderUtils.getSession().getAttribute(Constant.USER_SESSION);
String chanId = user.getChanId();
if(user!=null && !"01".equals(chanId)){
List<String> sameDeptUserList = this.stDeptService.selectSameDeptUser(chanId);
criteria.andCreateUserIn(sameDeptUserList);
}
//商户文件地址非空
criteria.andMerInfoFileAddressIsNotNull();
List<MerInfoTemp> tempList = this.merInfoService.selectByExample(example);
if(tempList == null || tempList.size() == 0){
logger.info("商户没有上传信息文件,请先上传文件,再下载。");
response.setContentType("text/html;charset=UTF-8");
response.getWriter().write("商户没有上传信息文件,请先上传文件,再下载。");
return null;
}
logger.info("商户管理,批量或全部下载查询到的总条数:{}",tempList.size());
for(int i = 0; i < tempList.size();i++){
MerInfoTemp temp =(MerInfoTemp)tempList.get(i);
logger.info("对应商户的主键为:{}",temp.getPkid());
}
String zipName = "MerTempFiles.zip";
response.setContentType("APPLICATION/OCTET-STREAM");
response.setHeader("Content-Disposition","attachment; filename="+zipName);
ZipOutputStream out = new ZipOutputStream(response.getOutputStream());
try{
for(Iterator<MerInfoTemp> it = tempList.iterator();it.hasNext();){
MerInfoTemp temp = it.next();
ZipUtils.doCompress(temp.getMerInfoFileAddress(), out);
response.flushBuffer();
}
}catch(Exception e){
logger.info("下载批量文件异常",e);
response.setContentType("text/html;charset=UTF-8");
response.getWriter().write("下载批量文件异常。");
return null;
}finally{
out.close();
}
return null;
}
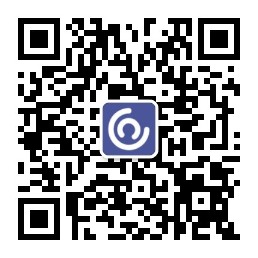
/*下载单个商户信息文件*/
@RequestMapping("/downLoadFile")
@ResponseBody
public Object downLoadFile(MerInfoTemp info,HttpServletResponse response) throws IOException{
response.setContentType("text/html;charset=UTF-8");
if(info == null){
logger.info("获取商户信息失败,无法下载。");
response.getWriter().write("获取商户信息失败,无法下载。");
return null;
}
logger.info("执行下载: MerInfoTempControler.downLoadFile()=>pkid="+info.getPkid());
try{
String pkid = info.getPkid();
MerInfoTemp temp = merInfoService.selectById(pkid);
//获取单个商户号的信息文件地址
String merInfoFileAddress = temp.getMerInfoFileAddress();
logger.info("下载商户信息文件的地址:{}",merInfoFileAddress);
if(merInfoFileAddress == null && StringUtils.isBlank(merInfoFileAddress)){
logger.info("商户没有上传信息文件,请先上传文件,再下载。");
response.getWriter().write("商户没有上传信息文件,请先上传文件,再下载。");
return null;
}
int num = merInfoFileAddress.lastIndexOf("/")+1;
String merInfoName = merInfoFileAddress.substring(num);
logger.info("商户信息文件名称:{}",merInfoName);
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
long fileLength = new File(merInfoFileAddress).length();
response.setHeader("content-disposition", "attachment;filename="+ new String(merInfoName.getBytes("gb2312"), "ISO8859-1"));
response.setHeader("Content-Length", String.valueOf(fileLength));
bis = new BufferedInputStream(new FileInputStream(merInfoFileAddress));
bos = new BufferedOutputStream(response.getOutputStream());
int bytesRead;
byte buff[] = new byte[2048];
while(-1 != (bytesRead = bis.read(buff, 0, buff.length))){
bos.write(buff, 0, bytesRead);
}
bis.close();
bos.close();
}catch(Exception e){
logger.info("下载商户信息异常",e);
response.getWriter().write("下载商户信息异常。");
return null;
}
return null;
}