1 必须安装
jdk1.8、maven、idea、mysql。
2 使用idea创建一个maven项目。其pom文件的内容如下:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>cn.ancony</groupId>
<artifactId>beetlsql</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<!--原生beetlSql的必备依赖-->
<dependency>
<groupId>com.ibeetl</groupId>
<artifactId>beetl-framework-starter</artifactId>
<version>1.1.40.RELEASE</version>
</dependency>
<!--Mysql数据库驱动依赖-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>6.0.5</version>
</dependency>
</dependencies>
<!-- Package as an executable jar -->
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3 编写应用程序。代码如下:
package cn.ancony.beetlsql;
import org.beetl.sql.core.*;
import org.beetl.sql.core.db.DBStyle;
import org.beetl.sql.core.db.MySqlStyle;
public class Application {
//数据库的配置参数
static String driver = "com.mysql.jdbc.Driver";
static String url = "jdbc:mysql://127.0.0.1:3306/orm?useUnicode=true&characterEncoding=UTF-8";
static String userName = "root";
static String password = "root";
public static void main(String[] args) throws Exception {
//通过ConnectionSourceHelper获得ConnectionSource的引用。
ConnectionSource source = ConnectionSourceHelper.getSimple(driver, url, userName, password);
//连接的数据库是mysql,使用MySqlStyle。
DBStyle mysql = new MySqlStyle();
// 最后,创建一个SQLManager
SQLManager sqlManager = new SQLManager(mysql, source);
gen(sqlManager);
}
//该方法会生成user的pojo对象到控制台。
public static void gen(SQLManager sqlManager) throws Exception {
sqlManager.genPojoCodeToConsole("user");
}
}
首先定义好数据库访问的配置。driver、url、username、password。
第二步,使用ConnectionSourceHelper类的getSimple方法得到一个ConnectionSource对象。
第三步,创建DBStyle,因为我们使用的是mysq数据库,所以创建的对象是MySqlStyle。
第四步,使用ConnectionSource和DBStyle对象创建SQLManager对象。
第五步,使用创建的SQLManager对象尽情地操作sql语句。
3 创建数据库表。
在数据库orm中,创建user表。建表语句如下:
扫描二维码关注公众号,回复:
2310533 查看本文章
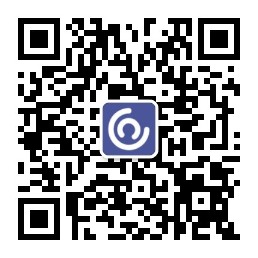
CREATE TABLE `user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(45) COLLATE utf8_bin DEFAULT NULL COMMENT '名称',
`department_id` int(11) DEFAULT NULL,
`create_time` date DEFAULT NULL COMMENT '创建时间',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_bin;
CREATE TABLE `department` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(45) COLLATE utf8_bin DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_bin;
INSERT INTO `user` VALUES (1, 'ancony', 1, '2018-7-18 11:52:41');
INSERT INTO `user` VALUES (1, 'conan', 1, '2018-7-18 11:52:41');
INSERT INTO `user` VALUES (1, 'david', 1, '2018-7-18 11:52:41');
INSERT INTO `department` VALUES ('1', '研发部门');
4 执行应用程序代码。
程序报错,错误内容简单如下:
Loading class `com.mysql.jdbc.Driver'. This is deprecated. The new driver class is `com.mysql.cj.jdbc.Driver'. The driver is automatically registered via the SPI and manual loading of the driver class is generally unnecessary.
Exception in thread "main" java.lang.RuntimeException: java.sql.SQLException: The server time zone value '�й���ʱ��' is unrecognized or represents more than one time zone. You must configure either the server or JDBC driver (via the serverTimezone configuration property) to use a more specifc time zone value if you want to utilize time zone support.
第一个问题:提示`com.mysql.jdbc.Driver'这个驱动过时了,使用`com.mysql.cj.jdbc.Driver'来代替。
所以直接将driver的值修改为符合要求的就可以了。
static String driver = "com.mysql.cj.jdbc.Driver";
第二个问题,提示时区错误,改为北京东八区的时间即可。
static String url = "jdbc:mysql://127.0.0.1:3306/orm?useUnicode=true&characterEncoding=UTF-8&serverTimezone=GMT%2B8";
SQLManager对象的genPojoCodeToConsole方法会自动生成POJO对象,并将这个结果输出到控制台,这个方法的参数就是数据库表的表名。
执行结果如下:
package com.test;
import java.math.*;
import java.util.Date;
import java.sql.Timestamp;
/*
*
* gen by beetlsql 2018-07-18
*/
public class user {
private Integer id ;
private Integer department_id ;
//名称
private String name ;
//创建时间
private Date create_time ;
public user() {
}
public Integer getId(){
return id;
}
public void setId(Integer id ){
this.id = id;
}
public Integer getDepartment_id(){
return department_id;
}
public void setDepartment_id(Integer department_id ){
this.department_id = department_id;
}
/**名称
*@return
*/
public String getName(){
return name;
}
/**名称
*@param name
*/
public void setName(String name ){
this.name = name;
}
/**创建时间
*@return
*/
public Date getCreate_time(){
return create_time;
}
/**创建时间
*@param create_time
*/
public void setCreate_time(Date create_time ){
this.create_time = create_time;
}
}