day01
删除索引:
drop index 索引字段 on 表名;
drop index idnumber on student;
删除自增:
alter table 表名 modify 字段 字段约束;
alter table student modify id int;
删除主键:
alter table 表名 drop primary key;
alter table student drop primary key;
一.索引
(1)MySQL中表的某些字段通过排序后生成一个固定格式的数据文件,这个文件称作索引文件,能够提高查询的速度
(2)为什么有了索引,查询的速度就会加快?
原理: B + 树
(3)创建索引:
普通索引:create index 索引名称 on 表名(字段列表); 所有字段都可以设置主键
唯一索引:create unique index 索引名称 on 表名(字段列表); 字段的值要有唯一性
主键索引:alter table 表名 add primary key(字段名); 主键一般设置一个,在id上,自增
全文索引:alter table 表名 add fulltext index 索引名称(字段名);
(4)优化select语句
查看查询语句的性能:explain
explain select...;
type---All
possible_keys---null
key---null
rows---1234 (遍历数据条数)
extra---using where
using filesort(表外排序,使用order by时出现,需要优化掉)
using temporary(使用group by时出现,需要优化掉)
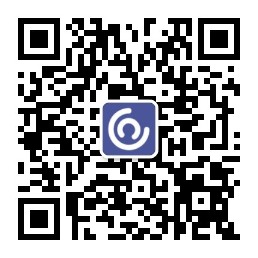
1.查询5班男生中,年龄最小的10人:
create index i_classid_sex_age on student(classid,sex,age); 创建联合索引
explain select * from student where classid=5 and sex=1 order by age asc limit 10;
2.查询5班每个年龄对应多少学生:
create index i_classid_age on student(classid,age);
explain select age,count(`age`) as num from student where classid=5 group by age;
强制使用某索引:
select * from student force index(i_classid_age) where......;
3.查询年龄最小的10人,要求显示班级的名称
create index i_age on student(age);
explain select s.sname,s.age,c.cname from student as s
join class as c
on s.classid=c.id
order by s.age
limit 10;
索引创建的原则:
1.where子句中出现的字段要创建索引
2.order by子句中出现的字段要创建索引
3.group by 多个子句同时出现或where ,order by, group by 中一个子句出现多个字段都要创建联合索引
4.join要优化每个表
5.如果extra中using filesort ,using temporary 一定要优化掉
day02
1.分区的原则:
页面展示数据根据哪个字段查询,就根据哪个字段进行拆分
2.分区表类型及创建
1.RANGE分区:根据一个字段值的范围,分配给不同的分区进行存储
2.LIST分区:把一个字段相同值对应的记录,放到一个分区进行存储
3.HASH分区:把一个字段的值进行hash散列,然后根据余数值分区存储
4.KEY分区:类似hash分区,key函数中包含字段至少有一个是整数字段
3.存储过程
1.创建存储过程:
create procedure 名称(in|out|inout 名称 类型,......)
begin
过程体;
end
过程体中可以使用所有的SQL,变量,运算,流程控制器语句,函数,
存储过程没有返回值.
delimiter(定界符) 改变sql语句的结束符
2.声明变量
declare 变量名称 类型(长度) default 默认值;
3.运算
比较运算:
=(等于)
=(赋值,使用set):set 变量=值;
逻辑运算:
and or not
4.条件语句
if 条件 then
select xxx;
elseif 条件
select yyy;
else
select zzz;
end if;
case 变量
when 值1 then
select '男';
when 值2 then
select '女';
else
select '保密';
end case;
5.循环语句
while 循环条件 do
变换步长;
end while;
repeat
变换步长;
until 终止条件;
end repeat;
6.函数
1.字符串函数
CONCAT(string2 [,...]) 连接子串
REPLACE(str,search_str,replace_str) 在str中用replace_str替换search_str
SUBSTRING(str,position[,length]) 从str的position开始,取length个字符
2.数学函数
CEILING(num2) 向上取整
FLOOR(num2) 向下取整
RAND([seed]) 随机数
ROUND(num[,decimals]) 四舍五入,decimals为小数位数
3.时间日期函数
CURRENT_DATE() 当前日期
CURRENT_TIME() 当前时间
NOW() 当前日期+时间
CURRENT_TIMESTAMP() 当前时间戳
day03
1.触发器
1.创建触发器
create trigger 触发器名称 before|after 事件 on 表名 for each row
begin
事件触发语句;
end//
2.事务
事务的特点:
原子性 一致性 隔离性 持久性
$pdo->beginTransaction(); //开启事务
$pdo->commit(); //提交事务
$pdo->rollback(); //事务回滚
3.用户权限
1.创建用户
create user 用户名@localhost identified by '密码';
2.分配权限
grant 权限 on 库.表 to 用户@localhost';
权限值:
all
insert,update,delete,select,create,alter,drop...
库.* //某个库中所有的表
*.* //所有库的所有表
3.回收权限
revoke 权限 on 库.表 from 用户@localhost;
4.重置密码
set password = password('新密码');
5.删除用户
drop user 用户名@localhost;
4.数据库的备份及恢复
备份数据库
mysqldump -u用户名 -p密码 数据库名>path/名称.sql
恢复数据库
use 数据库
source path/名称.sql
5.视图
一个虚拟的表,由一个查询语句产生.
create view 视图名称 as select 语句;
优点:简化查询语句,保护数据
----------------------------------------------------------------------------------------------
如有错误语法,请指正!!谢谢!!!