SpringBoot+CXF+Maven:点击打开链接
源码(百度云):点击下载源码
以发布StudentService服务为例
properties文件
# The default port is 8080
server.port=8088
gradle文件
buildscript {
ext {
springBootVersion = '1.5.10.RELEASE'
}
repositories {
mavenCentral()
}
dependencies {
classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}")
}
}
apply plugin: 'java'
apply plugin: 'eclipse-wtp'
apply plugin: 'org.springframework.boot'
apply plugin: 'war'
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
configurations {
providedRuntime
}
dependencies {
compile('org.springframework.boot:spring-boot-starter-web')
providedRuntime('org.springframework.boot:spring-boot-starter-tomcat')
testCompile('org.springframework.boot:spring-boot-starter-test')
//cxf 依赖
compile('org.apache.cxf:cxf-spring-boot-starter-jaxws:3.1.11')
}
启动类
package springboot.cxf.gradle;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class CxfApplication {
// SpringBoot 启动的地方
public static void main(String[] args) {
SpringApplication.run(CxfApplication.class, args);
}
}
配置类
package springboot.cxf.gradle.conf;
import javax.xml.ws.Endpoint;
import org.apache.cxf.Bus;
import org.apache.cxf.jaxws.EndpointImpl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springboot.cxf.gradle.services.SchoolService;
import springboot.cxf.gradle.services.StudentService;
/**
* Cxf 配置 ,等同于写XML文件,参照官网的demo
*/
@Configuration
public class CxfConfig {
@Autowired
private Bus bus; //必须有的
@Autowired
private StudentService studentService;
@Autowired
private SchoolService schoolService;
/**
* studentService
*
* @return
*/
@Bean
public Endpoint studentServiceEndpoint() {//发布studentService
EndpointImpl studentServiceEndpoint = new EndpointImpl(bus, studentService);
studentServiceEndpoint.publish("/studentService");
return studentServiceEndpoint;
}
/**
* schoolService
*
* @return
*/
@Bean
public Endpoint schoolServiceEndpoint() {
EndpointImpl schoolServiceEndpoint = new EndpointImpl(bus, schoolService);
schoolServiceEndpoint.publish("/schoolService");
return schoolServiceEndpoint;
}
}
实体类
package springboot.cxf.gradle.entities;
import java.io.Serializable;
public class Student implements Serializable {
private static final long serialVersionUID = 1L;// 反序列化用的,可以不写
private String name; // 姓名
private Integer age; // 年龄
private String gender; // 性别
public Student() {
super();
}
public Student(String name, Integer age, String gender) {
super();
this.name = name;
this.age = age;
this.gender = gender;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
@Override
public String toString() {
return "Student [name=" + name + ", age=" + age + ", gender=" + gender + "]";
}
}
接口类
package springboot.cxf.gradle.services;
import java.util.List;
import java.util.Map;
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebService;
import springboot.cxf.gradle.entities.Student;
@WebService(targetNamespace = "http://services.gradle.cxf.springboot") // 接口包名倒着写
public interface StudentService {
@WebMethod
public Integer getStudentAge(@WebParam(name = "name") String name);
@WebMethod
public String getStudentName();
@WebMethod
public Student getOneStudentInfo();
@WebMethod
public List<Student> getAllStudentsInfo();
@WebMethod
public Map<String, Object> getSchoolInfo();
}
接口实现类
扫描二维码关注公众号,回复:
2451752 查看本文章
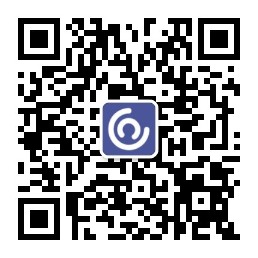
package springboot.cxf.gradle.services.impl;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.jws.WebService;
import org.springframework.stereotype.Service;
import springboot.cxf.gradle.entities.Student;
import springboot.cxf.gradle.services.StudentService;
@Service
@WebService(serviceName = "StudentService", // 服务名
targetNamespace = "http://impl.services.gradle.cxf.springboot", // 实现类包名倒写
endpointInterface = "springboot.cxf.gradle.services.StudentService") // 接口的全路径
public class StudentServiceImpl implements StudentService {
@Override
public Integer getStudentAge(String name) {
return new Student(name, 18, "男").getAge();
}
@Override
public String getStudentName() {
return "斗战神佛";
}
@Override
public Student getOneStudentInfo() {
Student stu = new Student("紫霞仙子", 18, "女");
return stu;
}
@Override
public List<Student> getAllStudentsInfo() {
List<Student> stus = new ArrayList<>();
Student stu1 = new Student("唐三藏", 20, "男");
Student stu2 = new Student("孙悟空", 20, "男");
Student stu3 = new Student("猪八戒", 20, "男");
Student stu4 = new Student("沙和尚", 20, "男");
stus.add(stu1);
stus.add(stu2);
stus.add(stu3);
stus.add(stu4);
return stus;
}
@Override
public Map<String, Object> getSchoolInfo() {
Map<String, Object> school = new HashMap<>();
school.put("name", "XX大学");
school.put("location", "广东");
return school;
}
}
接着启动SpringBoot
在浏览器输入:http://localhost:8088/services
调用服务
package springboot.cxf.gradle;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import springboot.cxf.gradle.entities.Student;
import springboot.cxf.gradle.services.SchoolService;
import springboot.cxf.gradle.services.StudentService;
public class CxfClientApplication {
public static void main(String[] args) {
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.setServiceClass(StudentService.class);
factory.setAddress("http://localhost:8088/services/studentService?wsdl");
StudentService service = (StudentService) factory.create();
String name = service.getStudentName();
System.out.println("student name:"+name);
Student stu = service.getOneStudentInfo();
System.out.println("学生信息:"+stu);
factory.setServiceClass(SchoolService.class);
factory.setAddress("http://localhost:8088/services/schoolService?wsdl");
SchoolService service2 = (SchoolService) factory.create();
String name1 = service2.getSchoolName();
System.out.println("学校名字:"+name1);
}
}
如果你是调用别人系统提供的webservice接口,那么你需要根据wsdl生成对应的接口类,这里我用jdk自带的wsimport工具生成
wsimport http://localhost:9020/services/studentService?wsdl -s D:\wsdl
-d:生成客户端执行类的class文件的存放目录
-s:生成客户端执行类的源文件的存放目录
-p:定义生成类的包名
异常:
Could not load Webservice SEI
检查 对应的接口实现类的endpointInterface的值是否写错,多了空格或者没写全