当项目中有多module时,在使用Butterknife的时候会发现在library模块中使用会出问题。当library模块中的页面通过butterknife找id的时候,就会报错。
如图,testbmodule模块plugin为library,也就是一个library的模块,然后看模块中的一个页面。
当使用butterknife插件找控件的时候就会报红线。
意思是@BindView的属性必须是一个常数,也就是说library module编译的时候,R文件中所有的数据并没有被加上final,也就是R文件中的数据并非常量。
@BindView
/**
* Bind a field to the view for the specified ID. The view will automatically be cast to the field
* type.
* <pre><code>
* {@literal @}BindView(R.id.title) TextView title;
* </code></pre>
*/
@Retention(CLASS) @Target(FIELD)
public @interface BindView {
/** View ID to which the field will be bound. */
@IdRes int value();
}
@IdRes
/**
* Bind a field to the view for the specified ID. The view will automatically be cast to the field
* type.
* <pre><code>
* {@literal @}BindView(R.id.title) TextView title;
* </code></pre>
*/
@Retention(CLASS) @Target(FIELD)
public @interface BindView {
/** View ID to which the field will be bound. */
@IdRes int value();
}
那么如何解决这个问题呢?我们可以利用一个butterknife的插件。下面来看看如何配置。
首先在项目的总build.gradle中添加classpath。
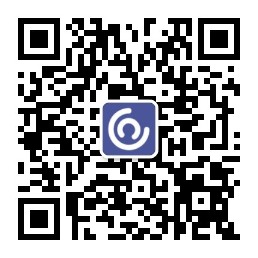
buildscript {
repositories {
jcenter()
mavenCentral()
// maven {
// url 'https://maven.google.com/'
// name 'Google'
// }
google()
}
dependencies {
classpath 'com.android.tools.build:gradle:3.0.1'
classpath 'com.jakewharton:butterknife-gradle-plugin:8.2.1'//添加butterknife插件的引用
}
在对应library模块的build.gradle中做如下配置。
apply plugin: 'com.android.library'
apply plugin: 'com.jakewharton.butterknife' //再次添加butterknife插件的应用
android {
compileSdkVersion rootProject.ext.compileSdkVersion
buildToolsVersion rootProject.ext.buildToolsVersion
defaultConfig {
minSdkVersion rootProject.ext.minSdkVersion
targetSdkVersion rootProject.ext.compileSdkVersion
multiDexEnabled true
versionCode 1
versionName "1.0"
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
}
signingConfigs {
release {
。。。。。。。。
}
}
buildTypes {
debug {
signingConfig signingConfigs.release
}
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
signingConfig signingConfigs.release
}
}
lintOptions {
abortOnError false
}
resourcePrefix "testbmodule_"
}
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
compile 'com.android.support.constraint:constraint-layout:1.0.2'
testCompile 'junit:junit:4.12'
androidTestCompile('com.android.support.test.espresso:espresso-core:3.0.1', {
exclude group: 'com.android.support', module: 'support-annotations'
})
compile "com.jakewharton:butterknife:$rootProject.butterknifeVersion" //添加butterknife的依赖
annotationProcessor "com.jakewharton:butterknife-compiler:$rootProject.butterknifeVersion" //添加butterknife相关依赖
}
这样基本就配置好了。来看看页面中如何使用吧。TestAActivity页面中。我们使用butterknife插件找到所有控件之后,把R改成R2,然后重新编译一下就可以了。
@Route(path = "/testa/testa")
public class TestAActivity extends BaseActivity {
@BindView(R2.id.check_login_button)
QMUIAlphaButton checkLoginButton;
@BindView(R2.id.check_camera_permission_button)
QMUIAlphaButton checkCameraPermissionButton;
@BindView(R2.id.arouter_jump_activity)
QMUIAlphaButton arouterJumpActivity;
@BindView(R2.id.arouter_iump_activity_with_data)
QMUIAlphaButton arouterIumpActivityWithData;
@BindView(R2.id.arouter_find_fragment)
QMUIAlphaButton arouterFindFragment;
@BindView(R2.id.arouter_find_service_button)
QMUIAlphaButton arouterFindServiceButton;
@BindView(R2.id.between_modules_button)
QMUIAlphaButton betweenModulesButton;
@BindView(R2.id.vc_button)
QMUIAlphaButton vcButton;
@BindView(R2.id.other_utils_and_widget_button)
QMUIAlphaButton otherUtilsAndWidgetButton;
@BindView(R2.id.single_click)
QMUIAlphaButton singleClickButton;
@Autowired(name = "testStr")
public String testStr;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
StatusBarUtils.translucent(this);
StatusBarUtils.setStatusBarLightMode(this);
但是,在使用的过程中还会有问题。
在使用switch的时候就会出问题。Resource IDs cannot be used in a switch statement in Android library modules......
那么解决方法就是,不使用switch case了呗,使用 if else 呗。
@OnClick({R2.id.single_click,R2.id.check_login_button, R2.id.check_camera_permission_button, R2.id.arouter_jump_activity, R2.id.arouter_iump_activity_with_data, R2.id.arouter_find_fragment, R2.id.arouter_find_service_button, R2.id.between_modules_button, R2.id.vc_button, R2.id.other_utils_and_widget_button, R2.id.data_base_example})
public void onViewClicked(View view) {
int i = view.getId();
if (i == R.id.check_login_button) {
checkLogin();
} else if (i == R.id.check_camera_permission_button) {
cameraPermission();
} else if (i == R.id.arouter_jump_activity) {
ARouter.getInstance().build("/testb/testb").navigation(this);
} else if (i == R.id.arouter_iump_activity_with_data) {
ARouter.getInstance().build("/testb/testb").withString("name", "这是一条数据").navigation(this);
} else if (i == R.id.arouter_find_fragment) {
ARouter.getInstance().build("/testb/tabsegment").navigation(this);
} else if (i == R.id.vc_button) {
ARouter.getInstance().build("/testa/testhttp").navigation(this);
} else if (i == R.id.data_base_example) {
这样,在library模块中,使用butterknife找控件报错的问题就解决了。