通过阅读源码可以看到,HashTable相对以前版本来说变化很小,而ConcurrentHashMap在jdk1.8的实现方式上有很大的变化,过去的ConcurrentHashMap是采用分段锁的segment来对一小段数组头节点进行加锁然后put、remove操作,而jdk1.8中的ConcurrentHashMap再次缩小了锁的粒度,结合了volatile,在每次put、remove操作时,对要操作的节点进行加锁,另外在扩容操作上也有新的改善,需要注意的是CAS操作在jdk1.8中ConcurrentHashMap的使用非常频繁。
HashTable相比HashMap是如何实现线程同步的
HashTable的实现相对HashMap非常简单,写过一篇HashMap的源码浅析,HashMap的源码就不分析了,这里介绍一下HashTable的实现。
介绍三个重要方法,put(K key, V value)/remove(Object key)/rehash()
与HashMap不同的是,HashTable采用的数据结构只有一个结构,即数组+链表结构,少了红黑树的转化等操作后代码真是少了非常多啊。
源码很容易读懂,这三个方法唯一需要解释的是rehash(),这个方法实际上是HashTable的扩容,而并没有被分离出来,每次扩容都是在put和remove等操作中,而加锁的方式是在put、remove等方法上加上Synchronize,对整个方法进行加锁,显而易见,锁的粒度是非常大的,只适用于小数据量的并发map。
ConcurrentHashMap源码浅析(一)put/remove方法中如何加锁
put方法
public V put(K key, V value) {
return putVal(key, value, false);
}
final V putVal(K key, V value, boolean onlyIfAbsent) {
if (key == null || value == null) throw new NullPointerException();
int hash = spread(key.hashCode());//再hash
int binCount = 0;
for (Node<K,V>[] tab = table;;) {
Node<K,V> f; int n, i, fh;
if (tab == null || (n = tab.length) == 0)
tab = initTable();//初始化表
else if ((f = tabAt(tab, i = (n - 1) & hash)) == null) {
if (casTabAt(tab, i, null,
new Node<K,V>(hash, key, value, null)))//使用cas给数组tab[i]赋值,创建新节点,防止重新创建新节点的操作
break; // no lock when adding to empty bin
}
else if ((fh = f.hash) == MOVED)//tab[i]该节点正处于扩容状态,帮助其进行扩容,后续会介绍扩容来解释
tab = helpTransfer(tab, f);
else {
V oldVal = null;
synchronized (f) {//加锁粒度是给产生hash冲突的tab[i]节点加锁,里面的操作和HashMap并没有多大的区别所以就不做介绍了
if (tabAt(tab, i) == f) {
if (fh >= 0) {
binCount = 1;
for (Node<K,V> e = f;; ++binCount) {
K ek;
if (e.hash == hash &&
((ek = e.key) == key ||
(ek != null && key.equals(ek)))) {
oldVal = e.val;
if (!onlyIfAbsent)
e.val = value;
break;
}
Node<K,V> pred = e;
if ((e = e.next) == null) {
pred.next = new Node<K,V>(hash, key,
value, null);
break;
}
}
}
else if (f instanceof TreeBin) {
Node<K,V> p;
binCount = 2;
if ((p = ((TreeBin<K,V>)f).putTreeVal(hash, key,
value)) != null) {
oldVal = p.val;
if (!onlyIfAbsent)
p.val = value;
}
}
}
}
if (binCount != 0) {
if (binCount >= TREEIFY_THRESHOLD)
treeifyBin(tab, i);//改造成红黑树
if (oldVal != null)
return oldVal;
break;
}
}
}
addCount(1L, binCount);//后面贴一下代码,实现的是实际容量sizeCtl + 1
return null;
}
private final void addCount(long x, int check) {//check if <0, don't check resize, if <= 1 only check if uncontended
CounterCell[] as; long b, s;
if ((as = counterCells) != null ||
!U.compareAndSwapLong(this, BASECOUNT, b = baseCount, s = b + x)) {
CounterCell a; long v; int m;
boolean uncontended = true;
if (as == null || (m = as.length - 1) < 0 ||
(a = as[ThreadLocalRandom.getProbe() & m]) == null ||
!(uncontended =
U.compareAndSwapLong(a, CELLVALUE, v = a.value, v + x))) {
fullAddCount(x, uncontended);//在有竞争条件下进行addCount操作,即多个线程执行该操作
return;
}
if (check <= 1)
return;
s = sumCount();//统计实际容量
}
if (check >= 0) {//是否处于扩容状态
Node<K,V>[] tab, nt; int n, sc;
while (s >= (long)(sc = sizeCtl) && (tab = table) != null &&
(n = tab.length) < MAXIMUM_CAPACITY) {
int rs = resizeStamp(n);
if (sc < 0) {
if ((sc >>> RESIZE_STAMP_SHIFT) != rs || sc == rs + 1 ||
sc == rs + MAX_RESIZERS || (nt = nextTable) == null ||
transferIndex <= 0)
break;
if (U.compareAndSwapInt(this, SIZECTL, sc, sc + 1))
transfer(tab, nt);//元素的转移,后面的扩容专题会介绍
}
else if (U.compareAndSwapInt(this, SIZECTL, sc,
(rs << RESIZE_STAMP_SHIFT) + 2))
transfer(tab, null);
s = sumCount();
}
}
}
remove方法
和put方法的实现有很多共同点,就不具体注释了
public V remove(Object key) {
return replaceNode(key, null, null);
}
final V replaceNode(Object key, V value, Object cv) {
int hash = spread(key.hashCode());//再hash
for (Node<K,V>[] tab = table;;) {
Node<K,V> f; int n, i, fh;
if (tab == null || (n = tab.length) == 0 ||
(f = tabAt(tab, i = (n - 1) & hash)) == null)
break;
else if ((fh = f.hash) == MOVED)
tab = helpTransfer(tab, f);
else {
V oldVal = null;
boolean validated = false;
synchronized (f) {//加锁的粒度与put一样
if (tabAt(tab, i) == f) {
if (fh >= 0) {
validated = true;
for (Node<K,V> e = f, pred = null;;) {
K ek;
if (e.hash == hash &&
((ek = e.key) == key ||
(ek != null && key.equals(ek)))) {
V ev = e.val;
if (cv == null || cv == ev ||
(ev != null && cv.equals(ev))) {
oldVal = ev;
if (value != null)
e.val = value;
else if (pred != null)
pred.next = e.next;
else
setTabAt(tab, i, e.next);
}
break;
}
pred = e;
if ((e = e.next) == null)
break;
}
}
else if (f instanceof TreeBin) {
validated = true;
TreeBin<K,V> t = (TreeBin<K,V>)f;
TreeNode<K,V> r, p;
if ((r = t.root) != null &&
(p = r.findTreeNode(hash, key, null)) != null) {
V pv = p.val;
if (cv == null || cv == pv ||
(pv != null && cv.equals(pv))) {
oldVal = pv;
if (value != null)
p.val = value;
else if (t.removeTreeNode(p))
setTabAt(tab, i, untreeify(t.first));
}
}
}
}
}
if (validated) {
if (oldVal != null) {
if (value == null)
addCount(-1L, -1);//实际容量减一
return oldVal;
}
break;
}
}
}
return null;
}
ConcurrentHashMap源码浅析(二)扩容的实现
先介绍一个静态内部类ForwardingNode
static final class ForwardingNode<K,V> extends Node<K,V> {
final Node<K,V>[] nextTable;
ForwardingNode(Node<K,V>[] tab) {
super(MOVED, null, null, null);
this.nextTable = tab;
}
Node<K,V> find(int h, Object k) {
// loop to avoid arbitrarily deep recursion on forwarding nodes
outer: for (Node<K,V>[] tab = nextTable;;) {
Node<K,V> e; int n;
if (k == null || tab == null || (n = tab.length) == 0 ||
(e = tabAt(tab, (n - 1) & h)) == null)
return null;
for (;;) {
int eh; K ek;
if ((eh = e.hash) == h &&
((ek = e.key) == k || (ek != null && k.equals(ek))))
return e;
if (eh < 0) {
if (e instanceof ForwardingNode) {
tab = ((ForwardingNode<K,V>)e).nextTable;
continue outer;
}
else
return e.find(h, k);
}
if ((e = e.next) == null)
return null;
}
}
}
}
可以看到该类中维护了一个对象,该对象存储的是map扩容后的数组tab,只在扩容的时候不为空。
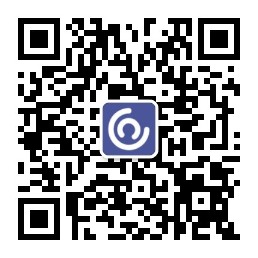
主类ConcurrentHashMap中也维护了一个私有的全局变量
/**
* The next table to use; non-null only while resizing.
*/
private transient volatile Node<K,V>[] nextTable;
该类的用途主要是为了标识ConcurrentHashMap中的数组节点是否处于扩容的状态,如果处于扩容的状态,就会在内部维护一个nextTable[]数组对象,那么为什么要维护这样一个对象呢,继续往后看。
transfer()和helpTransfer()
前面put操作中执行了helpTransfer(),而在addCount()中执行了transfer(),transfer()是map扩容时做的操作,不多说贴代码:
private final void transfer(Node<K,V>[] tab, Node<K,V>[] nextTab) {
int n = tab.length, stride;
if ((stride = (NCPU > 1) ? (n >>> 3) / NCPU : n) < MIN_TRANSFER_STRIDE)
stride = MIN_TRANSFER_STRIDE; // subdivide range stride是最小的transfer单位
if (nextTab == null) { // initiating,如果nextTab为空,默认进行两倍的扩容
try {
@SuppressWarnings("unchecked")
Node<K,V>[] nt = (Node<K,V>[])new Node<?,?>[n << 1];
nextTab = nt;
} catch (Throwable ex) { // try to cope with OOME
sizeCtl = Integer.MAX_VALUE;
return;
}
nextTable = nextTab;
transferIndex = n;
}
int nextn = nextTab.length;
ForwardingNode<K,V> fwd = new ForwardingNode<K,V>(nextTab);//创建ForwardingNode节点,用于标识tab[i]处于扩容状态
boolean advance = true;//advance指的是做完了一个位置的迁徙工作,可以准备做下一个位置的了
boolean finishing = false; // to ensure sweep before committing nextTab
for (int i = 0, bound = 0;;) {
Node<K,V> f; int fh;
while (advance) {
int nextIndex, nextBound;
if (--i >= bound || finishing)
advance = false;
else if ((nextIndex = transferIndex) <= 0) {
i = -1;
advance = false;
}
else if (U.compareAndSwapInt
(this, TRANSFERINDEX, nextIndex,
nextBound = (nextIndex > stride ?
nextIndex - stride : 0))) {// 实施任务分派,更新了 TRANSFERINDEX 其他线程能看到
bound = nextBound;
i = nextIndex - 1;
advance = false;
}
}
if (i < 0 || i >= n || i + n >= nextn) {
int sc;
if (finishing) {//若扩容完成
nextTable = null;
table = nextTab;
sizeCtl = (n << 1) - (n >>> 1);
return;
}
if (U.compareAndSwapInt(this, SIZECTL, sc = sizeCtl, sc - 1)) {// 任务结束,方法退出
if ((sc - 2) != resizeStamp(n) << RESIZE_STAMP_SHIFT)
return;
finishing = advance = true;
i = n; // recheck before commit
}
}
else if ((f = tabAt(tab, i)) == null)
advance = casTabAt(tab, i, null, fwd);//cas操作替换为tab[i]=fwd,防止多个线程重复替换
else if ((fh = f.hash) == MOVED)
advance = true; // already processed
else {
synchronized (f) {//把该正在扩容状态的节点上锁
if (tabAt(tab, i) == f) {//链表结构
Node<K,V> ln, hn;
if (fh >= 0) {
int runBit = fh & n;
Node<K,V> lastRun = f;
for (Node<K,V> p = f.next; p != null; p = p.next) {//找到最后一段无需更改位置的有序节点
int b = p.hash & n;
if (b != runBit) {
runBit = b;
lastRun = p;
}
}
if (runBit == 0) {
ln = lastRun;
hn = null;
}
else {
hn = lastRun;
ln = null;
}
for (Node<K,V> p = f; p != lastRun; p = p.next) {//对lastRun前的节点进行重连接
int ph = p.hash; K pk = p.key; V pv = p.val;
if ((ph & n) == 0)
ln = new Node<K,V>(ph, pk, pv, ln);
else
hn = new Node<K,V>(ph, pk, pv, hn);
}
setTabAt(nextTab, i, ln);
setTabAt(nextTab, i + n, hn);
setTabAt(tab, i, fwd);
advance = true;
}
else if (f instanceof TreeBin) { // 红黑树的迁移,和链表一样,先分裂成两个树,再分别移植在tab[i]和tab[i+n],就不详细备注了
TreeBin<K,V> t = (TreeBin<K,V>)f;
TreeNode<K,V> lo = null, loTail = null;
TreeNode<K,V> hi = null, hiTail = null;
int lc = 0, hc = 0;
for (Node<K,V> e = t.first; e != null; e = e.next) {
int h = e.hash;
TreeNode<K,V> p = new TreeNode<K,V>
(h, e.key, e.val, null, null);
if ((h & n) == 0) {
if ((p.prev = loTail) == null)
lo = p;
else
loTail.next = p;
loTail = p;
++lc;
}
else {
if ((p.prev = hiTail) == null)
hi = p;
else
hiTail.next = p;
hiTail = p;
++hc;
}
}
ln = (lc <= UNTREEIFY_THRESHOLD) ? untreeify(lo) :
(hc != 0) ? new TreeBin<K,V>(lo) : t;
hn = (hc <= UNTREEIFY_THRESHOLD) ? untreeify(hi) :
(lc != 0) ? new TreeBin<K,V>(hi) : t;
setTabAt(nextTab, i, ln);
setTabAt(nextTab, i + n, hn);
setTabAt(tab, i, fwd);
advance = true;
}
}
}
}
}
}
网上引用这张图,很形象,帮助我们的理解。
再看一下helpTransfer,实际上就是用cas操作来帮助完成上面transfer中分解出来的小任务(以stribe为单位进行分解,stribe是tab上的一个短数组)
final Node<K,V>[] helpTransfer(Node<K,V>[] tab, Node<K,V> f) {
Node<K,V>[] nextTab; int sc;
if (tab != null && (f instanceof ForwardingNode) &&
(nextTab = ((ForwardingNode<K,V>)f).nextTable) != null) {//把正在transfer的nextTab赋值给nextTab
int rs = resizeStamp(tab.length);
while (nextTab == nextTable && table == tab &&
(sc = sizeCtl) < 0) {//当transfer还没有结束
if ((sc >>> RESIZE_STAMP_SHIFT) != rs || sc == rs + 1 ||
sc == rs + MAX_RESIZERS || transferIndex <= 0)
break;
if (U.compareAndSwapInt(this, SIZECTL, sc, sc + 1)) {//把SIZECTL加一,然后transfer
transfer(tab, nextTab);
break;
}
}
return nextTab;
}
return table;
}
ConcurrentHashMap源码浅析(三)静态内部类Traverser迭代器
用于containsValue(Object)方法的迭代器,在mapping中搜索该value,每次执行advance()操作都会更新使用最新的table继续遍历。对advance()的实现始终不太明白(e=null后e又在tabAt(t,i)中取值,那最后是由于++baseIndex>=baseLimit而return出去,那baseLimit即表示的是map当前总容量???),不过还是贴一下代码:
final Node<K,V> advance() {
Node<K,V> e;
if ((e = next) != null)
e = e.next;
for (;;) {
Node<K,V>[] t; int i, n; // must use locals in checks
if (e != null)
return next = e;
if (baseIndex >= baseLimit || (t = tab) == null ||
(n = t.length) <= (i = index) || i < 0)
return next = null;
if ((e = tabAt(t, i)) != null && e.hash < 0) {
if (e instanceof ForwardingNode) {//若该节点处于扩容状态
tab = ((ForwardingNode<K,V>)e).nextTable;//更新表
e = null;//重置e指针
pushState(t, i, n);//将此时的表状态push入栈
continue;
}
else if (e instanceof TreeBin)//若该节点是树节点
e = ((TreeBin<K,V>)e).first;//沿着树遍历
else
e = null;
}
if (stack != null)
recoverState(n);//从栈中恢复出当前的表长
else if ((index = i + baseSize) >= n)
index = ++baseIndex; // visit upper slots if present
}
}
有问题请指出,欢迎相互学习交流