学习参考自NAYNEHC博客园
该框架使用方法有很多,当前使用 [javaBean]Mapper.xml 简单映射接口实现增删改查的方法:
配置mybatis有---<configuration>
-------><properties>属性文件配置,如数据库,日记记录
-------><environments>配置环境(可多个,如各种数据库厂商)
-----------------------------><environment>其中一个
--------------------------------------------------><transactionManager>事务管理
--------------------------------------------------><dataSource>配置数据源
-------><mappers>映射注册配置(可多个)
----------------------><mapper>一个映射类位置
配置映射类 [javaBean]Mapper.xml,对数据库sql语句映射 如:
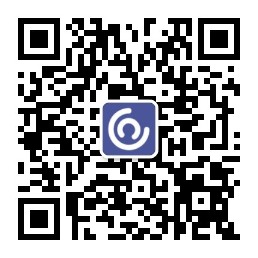
<insert id="insertStudent">
insert into student(sno,sname,score) values(#{sno},#{sname},#{score})
</insert>
具体实现例子如下:
1、结构目录,需要导入mybatis、mysql的jar包
2、mybatis.cfg.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!-- 引入外部文件 -->
<properties resource="mysql.properties"/>
<environments default="develpment">
<environment id="develpment">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="${driver}"/>
<property name="url" value="${url}"/>
<property name="username" value="${user}"/>
<property name="password" value="${password}"/>
</dataSource>
</environment>
</environments>
<mappers>
<mapper resource="com/crud/mapper/StudentMapper.xml"/>
<!-- <package name="com/crud/mapper"/> -->
</mappers>
</configuration>
3、mysql.properties
4、Student实体类
package com.crud.model;
public class Student {
private int sno;
private String sname;
private double score;
@Override
public String toString() {
return "Student [sno=" + sno + ", sname=" + sname + ", score=" + score + "]";
}
public Student() {
super();
}
public Student(int sno, String sname, double score) {
super();
this.sno = sno;
this.sname = sname;
this.score = score;
}
public int getSno() {
return sno;
}
public void setSno(int sno) {
this.sno = sno;
}
public String getSname() {
return sname;
}
public void setSname(String sname) {
this.sname = sname;
}
public double getScore() {
return score;
}
public void setScore(double score) {
this.score = score;
}
}
5、mapper包下的crud方法接口类,实现类、对象的sql映射文件
package com.crud.mapper;
import java.util.List;
import com.crud.model.Student;
public interface InterStudentMapper {
public int insertStudent(Student student);
public int deleteStudent(int sno);
public int updateStudent(Student student);
public Student selectStudent(int sno);
public List<Student> selectAllStudent();
}
package com.crud.mapper;
import java.util.List;
import org.apache.ibatis.session.SqlSession;
import com.crud.model.Student;
import com.crud.tool.DBtool;
public class StudentMapperImpl implements InterStudentMapper {
@Override
public int insertStudent(Student student) {
SqlSession sqlSession=DBtool.getSqlSession();
InterStudentMapper mapper=sqlSession.getMapper(InterStudentMapper.class);
try{ //线程不安全类型,放在方法体中。
mapper.insertStudent(student);
sqlSession.commit();
System.out.println("had inserted:"+student);
}catch(Exception e){
e.printStackTrace();
sqlSession.rollback();
return 1;
}finally{
sqlSession.close();
}
return 0;
}
@Override
public int deleteStudent(int sno) {
SqlSession sqlSession=DBtool.getSqlSession();
InterStudentMapper mapper=sqlSession.getMapper(InterStudentMapper.class);
try{
mapper.deleteStudent(sno);
sqlSession.commit();
System.out.println("had deleted:"+sno);
}catch(Exception e){
e.printStackTrace();
sqlSession.rollback();
return 1;
}finally{
sqlSession.close();
}
return 0;
}
@Override
public int updateStudent(Student student) {
SqlSession sqlSession=DBtool.getSqlSession();
InterStudentMapper mapper=sqlSession.getMapper(InterStudentMapper.class);
try{
Student before=mapper.selectStudent(student.getSno());
mapper.updateStudent(student);
sqlSession.commit();
Student after=mapper.selectStudent(student.getSno());
sqlSession.commit();
System.out.println(before+" had changed:"+after);
}catch(Exception e){
e.printStackTrace();
sqlSession.rollback();
return 1;
}finally{
sqlSession.close();
}
return 0;
}
@Override
public Student selectStudent(int sno) {
Student stu=null;
SqlSession sqlSession=DBtool.getSqlSession();
InterStudentMapper mapper=sqlSession.getMapper(InterStudentMapper.class);
try{
stu=mapper.selectStudent(sno);
sqlSession.commit();
}catch(Exception e){
e.printStackTrace();
sqlSession.rollback();
return null;
}finally{
sqlSession.close();
}
return stu;
}
@Override
public List<Student> selectAllStudent() {
List<Student> list=null;
SqlSession sqlSession=DBtool.getSqlSession();
InterStudentMapper mapper=sqlSession.getMapper(InterStudentMapper.class);
try{
list=mapper.selectAllStudent();
sqlSession.commit();
}catch(Exception e){
e.printStackTrace();
sqlSession.rollback();
return null;
}finally{
sqlSession.close();
}
return list;
}
}
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org/DTD Mapper 3.0" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<!-- 对接口方法进行映射 -->
<mapper namespace="com.crud.mapper.InterStudentMapper">
<!-- 自定义resultMap结果集类型 -->
<resultMap id="StudentMap" type="com.crud.model.Student">
<id property="sno" column="sno" javaType="java.lang.Integer"/>
<result property="sname" column="sname" javaType="java.lang.String"/>
<result property="score" column="score" javaType="java.lang.Double"/>
</resultMap>
<!-- id值与接口方法同名 -->
<insert id="insertStudent">
<!-- 数据库字段名 实体类属性名(占位符) -->
insert into student(sno,sname,score) values(#{sno},#{sname},#{score})
</insert>
<!-- parameterType参数类型设置 -->
<update id="updateStudent" parameterType="com.crud.model.Student">
update student set sname=#{sname},score=#{score} where sno=#{sno}
</update>
<!-- 参数类型设置 -->
<delete id="deleteStudent" parameterType="int">
delete from student where sno=#{sno}
</delete>
<!-- resultType结果集类型(没有设置别名时,使用完全限定名) -->
<select id="selectStudent" resultType="com.crud.model.Student">
select * from student where sno=#{sno}
</select>
<select id="selectAllStudent" resultMap="StudentMap">
select * from student
</select>
</mapper>
6、数据库操作工具类
package com.crud.tool;
import java.io.IOException;
import java.io.Reader;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
public class DBtool {
public static SqlSessionFactory sqlSessionFactory;
static{
try {
Reader reader=Resources.getResourceAsReader("mybatis.cif.xml");
sqlSessionFactory=new SqlSessionFactoryBuilder().build(reader);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public static SqlSession getSqlSession(){
return sqlSessionFactory.openSession();
}
}
7、增删改查服务测试
package com.crud.service;
import java.util.List;
import com.crud.mapper.InterStudentMapper;
import com.crud.mapper.StudentMapperImpl;
import com.crud.model.Student;
public class StudentService{
public static void main(String[] args) {
InterStudentMapper stuMapper=new StudentMapperImpl();
stuMapper.insertStudent(new Student(1002,"gan",100));
stuMapper.deleteStudent(1100);
stuMapper.updateStudent(new Student(1000,"master",88));
Student stu=stuMapper.selectStudent(1001);
System.out.println(stu);
List<Student> list=stuMapper.selectAllStudent();
System.out.println(list);
}
}
8、结果
前
后