过渡动画
过渡动画是在Android 4.4(api>=19)引入的新的动画框架,本质上是对属性动画的封装,方便实现Activity或者View的过渡动画效果。
Scene
Scene定义了页面的当前状态信息,Scene的实例化通过静态工厂方法实现
/*
* @param sceneRoot The root of the hierarchy in which scene changes
* and transitions will take place.
* @param layoutId The id of a standard layout resource file.
* @param context The context used in the process of inflating
* the layout resource.
* @return The scene for the given root and layout id
*/
public static Scene getSceneForLayout(ViewGroup sceneRoot, int layoutId, Context context)
Transition
Transition定义了界面之前切换的动画信息
TransitionManager
TransitionManager用于控制Scene之间的切换动画效果,切换的常用方法如下:
/*
* Convenience method to simply change to the given scene using
* the default transition for TransitionManager.
*
* @param scene The Scene to change to
*/
public static void go(Scene scene) {
changeScene(scene, sDefaultTransition);
}
/**
* Convenience method to simply change to the given scene using
* the given transition.
*
* <p>Passing in <code>null</code> for the transition parameter will
* result in the scene changing without any transition running, and is
* equivalent to calling {@link Scene#exit()} on the scene root's
* current scene, followed by {@link Scene#enter()} on the scene
* specified by the <code>scene</code> parameter.</p>
*
* @param scene The Scene to change to
* @param transition The transition to use for this scene change. A
* value of null causes the scene change to happen with no transition.
*/
public static void go(Scene scene, Transition transition) {
changeScene(scene, transition);
}
代码动画
首先定义同一页面的两个布局,分别是动画前和动画后的布局,布局如下
动画前:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/scene"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello Android" />
</RelativeLayout>
动画后:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/scene"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="Hello Android" />
</RelativeLayout>
动画前的TextView显示在左上角,动画后TextView居中显示
在Activity->onCreate方法中加入
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) {
final Scene scene2 = Scene.getSceneForLayout(container, R.layout.fragment_transition_scene_after, this);
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
TransitionManager.go(scene2);
}
}, 3000);
}
首先判断版本大于等于4.4,创建一个Scene对象,加载动画后的布局文件,延时3秒后执行动画 ,使用TransitionManager.go方法。
xml动画
在res目录下创建transition目录(存放过渡动画xml)
创建文件
<?xml version="1.0" encoding="utf-8"?>
<transitionManager xmlns:android="http://schemas.android.com/apk/res/android">
<transition
android:fromScene="@layout/fragment_transition_scene_before"
android:toScene="@layout/fragment_transition_scene_after" />
</transitionManager>
fromScene动画前布局文件
toScene动画后布局文件
Activity->onCreate方法中加入
ViewGroup container = findViewById(R.id.container1);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) {
TransitionInflater transitionInflater = TransitionInflater.from(this);
final TransitionManager mTransitionManager = transitionInflater.inflateTransitionManager(R.transition.transition_manager, container);
final Scene scene1 = Scene.getSceneForLayout(container, R.layout.fragment_transition_scene_before, this);
final Scene scene2 = Scene.getSceneForLayout(container, R.layout.fragment_transition_scene_after, this);
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
mTransitionManager.transitionTo(scene2);
}
}, 3000);
}
运行效果和上述代码方式一样,这里使用的默认AutoTransition动画。
扫描二维码关注公众号,回复:
2591772 查看本文章
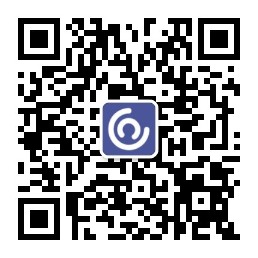