文件的上传下载是在web应用中常用的功能之一,这篇博客就简单的使用纯Jsp+Sevlet完成文件上传下载的功能。
平时在做一些数据提交的时候通常使用表单,在form表单有一个属性enctype
,该属性规定在发送到服务器之前应该如何对表单数据进行编码。属性通常取以下值:
值 | 描述 |
---|---|
application/x-www-form-urlencoded | 在发送前编码所有字符(默认) |
multipart/form-data | 不对字符编码。在使用包含文件上传控件的表单时,必须使用该值。 |
text/plain | 空格转换为 “+” 加号,但不对特殊字符编码。 |
默认地,表单数据会编码为 “application/x-www-form-urlencoded”。就是说,在发送到服务器之前,所有字符都会进行编码(空格转换为 “+” 加号,特殊符号转换为 ASCII HEX 值)。
以上表是表单提交数据的方式,文件上传时enctype属性必须为multipart/form-data,如下图,是上传一个文件时的抓包结果:
我们主要关注以下部分:
最上面第一行定义每个Part的分割线,我们再在chrome控制台查看一下上传一个文件的Request Headers部分和Request Payload部分:
Request Headers部分:
Accept:text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8
Accept-Encoding:gzip, deflate, br
Accept-Language:zh-CN,zh;q=0.8
Cache-Control:no-cache
Connection:keep-alive
Content-Length:9183
Content-Type:multipart/form-data; boundary=----WebKitFormBoundarypDuuIiu30BxbEwRk
Cookie:UM_distinctid=15b65518f3dabb-0fbc5896a8480e-317d0258-100200-15b65518f3ea83; CNZZDATA1260603825=1598551632-1492051621-%7C1492051621; JSESSIONID=1315720EE333FB179499CBC5144BB44B
Host:localhost:8080
Origin:http://localhost:8080
Pragma:no-cache
Referer:http://localhost:8080/jsp/01.jsp
Upgrade-Insecure-Requests:1
User-Agent:Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.81 Safari/537.36
Request Payload部分:
------WebKitFormBoundarypDuuIiu30BxbEwRk
Content-Disposition: form-data; name="myfile"; filename="examples.desktop"
Content-Type: application/x-desktop
------WebKitFormBoundarypDuuIiu30BxbEwRk--
也就是说在请求头中通过Content-Type:multipart/form-data; boundary=----WebKitFormBoundarypDuuIiu30BxbEwRk
这句定义了内容类型,并且定义了每个Part的分界线,所以我们就可以根据这种格式再在servlet中解析得到最终文件了。
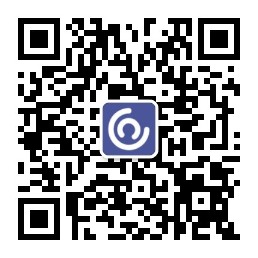
这里借用mooc上的一个教程代码(亲测可用)来说明问题,具体的含义在代码注释中很详细了:
UploadServlet.java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.RandomAccessFile;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class UploadServlet extends HttpServlet {
public void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doPost(req,resp);
}
public void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
//从request当中获取流信息
InputStream fileSource = req.getInputStream();
String tempFileName = "/home/zhuxinquan/temp/tempFile";
//tempFile指向临时文件
File tempFile = new File(tempFileName);
//outputStram文件输出流指向这个临时文件
FileOutputStream outputStream = new FileOutputStream(tempFile);
byte b[] = new byte[1024];
int n;
while(( n = fileSource.read(b)) != -1){
outputStream.write(b, 0, n);
}
//关闭输出流、输入流
outputStream.close();
fileSource.close();
//获取上传文件的名称
RandomAccessFile randomFile = new RandomAccessFile(tempFile,"r");
randomFile.readLine();
String str = randomFile.readLine();
int beginIndex = str.lastIndexOf("filename=\"") + 10;
int endIndex = str.lastIndexOf("\"");
String filename = str.substring(beginIndex, endIndex);
System.out.println("filename:" + filename);
//重新定位文件指针到文件头
randomFile.seek(0);
long startPosition = 0;
int i = 1;
//获取文件内容 开始位置
//开始位置的获取是因为Request Payload部分的第五行开始的
while(( n = randomFile.readByte()) != -1 && i <=4){
if(n == '\n'){
startPosition = randomFile.getFilePointer();
i ++;
}
}
startPosition = randomFile.getFilePointer() -1;
//获取文件内容 结束位置
//从后往前获取到倒数第二行结束
randomFile.seek(randomFile.length());
long endPosition = randomFile.getFilePointer();
int j = 1;
while(endPosition >=0 && j<=2){
endPosition--;
randomFile.seek(endPosition);
if(randomFile.readByte() == '\n'){
j++;
}
}
endPosition = endPosition -1;
//设置保存上传文件的路径
String realPath = getServletContext().getRealPath("/") + "images";
File fileupload = new File(realPath);
if(!fileupload.exists()){
fileupload.mkdir();
}
File saveFile = new File(realPath,filename);
RandomAccessFile randomAccessFile = new RandomAccessFile(saveFile,"rw");
//从临时文件当中读取文件内容(根据起止位置获取)
randomFile.seek(startPosition);
while(startPosition < endPosition){
randomAccessFile.write(randomFile.readByte());
startPosition = randomFile.getFilePointer();
}
//关闭输入输出流、删除临时文件
randomAccessFile.close();
randomFile.close();
tempFile.delete();
req.setAttribute("result", "上传成功!");
RequestDispatcher dispatcher = req.getRequestDispatcher("jsp/01.jsp");
dispatcher.forward(req, resp);
}
}
需要注意的是,上面的代码主要针对单个文件进行处理,若一次上传包含多个文件或字段可根据请求头中的Content-Type字段取得boundary值,将请求的多个字段分开,分别获取即可。
文件下载实现较为简单,前端页面自然需要将文件链接进行展示,后端就可根据下载请求的文件名参数获取到文件输出流即可,代码实现如下:
DownloadServlet.java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class DownloadServlet extends HttpServlet {
public void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
//获取文件下载路径
String path = getServletContext().getRealPath("/") + "images/";
String filename = req.getParameter("filename");
File file = new File(path + filename);
if(file.exists()){
//设置相应类型application/octet-stream
resp.setContentType("application/x-msdownload");
//设置头信息
resp.setHeader("Content-Disposition", "attachment;filename=\"" + filename + "\"");
InputStream inputStream = new FileInputStream(file);
ServletOutputStream ouputStream = resp.getOutputStream();
byte b[] = new byte[1024];
int n ;
while((n = inputStream.read(b)) != -1){
ouputStream.write(b,0,n);
}
//关闭流、释放资源
ouputStream.close();
inputStream.close();
}else{
req.setAttribute("errorResult", "文件不存在下载失败!");
RequestDispatcher dispatcher = req.getRequestDispatcher("jsp/01.jsp");
dispatcher.forward(req, resp);
}
}
public void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doGet(req,resp);
}
}
上面的请求处理过程很简单,就是获取请求下载的文件名,查找文件,并获取响应输出流并输出即可,需要注意的是,为了使文件下载,我们可以设置响应头部:resp.setContentType("application/x-msdownload");
、resp.setHeader("Content-Disposition", "attachment;filename=\"" + filename + "\"");
让文件默认直接下载,并且下载的文件名为文件原名。
文件上传的方式多种多样,已有多种框架集成文件上传功能,该篇只是最基本的一种,后续再看看其它的实现方式吧。