链接:https://www.nowcoder.com/acm/contest/141/C
来源:牛客网
题目描述
Eddy likes to play cards game since there are always lots of randomness in the game. For most of the cards game, the very first step in the game is shuffling the cards. And, mostly the randomness in the game is from this step. However, Eddy doubts that if the shuffling is not done well, the order of the cards is predictable!
To prove that, Eddy wants to shuffle cards and tries to predict the final order of the cards. Actually, Eddy knows only one way to shuffle cards that is taking some middle consecutive cards and put them on the top of rest. When shuffling cards, Eddy just keeps repeating this procedure. After several rounds, Eddy has lost the track of the order of cards and believes that the assumption he made is wrong. As Eddy's friend, you are watching him doing such foolish thing and easily memorizes all the moves he done. Now, you are going to tell Eddy the final order of cards as a magic to surprise him.
Eddy has showed you at first that the cards are number from 1 to N from top to bottom.
For example, there are 5 cards and Eddy has done 1 shuffling. He takes out 2-nd card from top to 4-th card from top(indexed from 1) and put them on the top of rest cards. Then, the final order of cards from top will be [2,3,4,1,5].
输入描述:
The first line contains two space-separated integer N, M indicating the number of cards and the number of shuffling Eddy has done. Each of following M lines contains two space-separated integer pi, si indicating that Eddy takes pi-th card from top to (pi+si-1)-th card from top(indexed from 1) and put them on the top of rest cards. 1 ≤ N, M ≤ 105 1 ≤ pi ≤ N 1 ≤ si ≤ N-pi+1
输出描述:
Output one line contains N space-separated integers indicating the final order of the cards from top to bottom.
示例1
输入
5 1 2 3
输出
2 3 4 1 5
示例2
输入
5 2 2 3 2 3
输出
3 4 1 2 5
示例3
输入
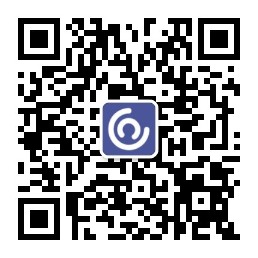
5 3 2 3 1 4 2 4
输出
3 4 1 5 2
题意:有n张扑克牌,初始从1到n顺序排放,m次操作,每次操作把区间[p,p+s-1]的牌取出放在最前面,输出m次操作后的序列
思路:伸展树的模板题,看了一天的伸展树和Treap,似懂非懂,另一种写法是用rope,其实rope的底层实现也是平衡树,rope比较耗时
#include <cstdio>
#include <algorithm>
#include <vector>
using namespace std;
struct Node
{
Node *ch[2];
int s,v;
int cmp(int k)
{
int d = k - ch[0] -> s;
if(d == 1) return -1;
return d <= 0 ? 0 : 1;
}
void maintain()
{
s = 1;
if(ch[0] != NULL) s += ch[0] -> s;
if(ch[1] != NULL) s += ch[1] -> s;
}
};
Node *null = new Node();
void Rotate(Node* &o,int d)
{
Node *k = o -> ch[d ^ 1];
o -> ch[d ^ 1] = k -> ch[d];
k -> ch[d] = o;
o -> maintain();
k -> maintain();
o = k;
}
void Splay(Node* &o,int k)
{
int d = o -> cmp(k);
if(d == 1) k -= o -> ch[0] -> s + 1;
if(d != -1) {
Node *p = o -> ch[d];
int d2 = p -> cmp(k);
int k2 = (d2 == 0 ? k : k - p -> ch[0] -> s - 1);
if(d2 != -1) {
Splay(p -> ch[d2],k2);
if(d == d2) Rotate(o,d ^ 1);
else Rotate(o -> ch[d],d);
}
Rotate(o,d ^ 1);
}
}
Node* Merge(Node* left,Node* right)
{
Splay(left,left -> s);
left -> ch[1] = right;
left -> maintain();
return left;
}
void Split(Node* o,int k,Node* &left,Node* &right)
{
Splay(o,k);
left = o;
right = o -> ch[1];
o -> ch[1] = null;
left -> maintain();
}
const int maxn = 100000 + 100;
struct SplaySequence
{
int n;
Node seq[maxn];
Node *root;
Node *build(int sz)
{
if(sz == 0) return null;
Node* L = build(sz / 2);
Node* o = &seq[++n];
o -> v = n;
o -> ch[0] = L;
o -> ch[1] = build(sz - sz / 2 - 1);
o -> s = 0;
o -> maintain();
return o;
}
void init(int sz)
{
n = 0;
null -> s = 0;
root = build(sz);
}
};
vector<int> ans;
void print(Node *o)
{
if(o != null)
{
print(o -> ch[0]);
ans.push_back(o -> v);
print(o -> ch[1]);
}
}
SplaySequence ss;
int main(void)
{
int n,m;
scanf("%d%d",&n,&m);
ss.init(n + 1);
while(m--) {
int a,b;
scanf("%d%d",&a,&b);
if(a == 1) continue;
b = a + b - 1;
Node *left,*mid,*right,*o,*first,*_left;
Split(ss.root,a,left,o);
Split(o,b - a + 1,mid,right);
Split(left,1,first,_left);
ss.root = Merge(Merge(first,mid),Merge(_left,right));
}
print(ss.root);
for(int i = 1; i < ans.size(); i++) {
if(i == 1) printf("%d",ans[i] - 1);
else printf(" %d",ans[i] - 1);
}
printf("\n");
return 0;
}
rope写法,非常简洁
#include<bits/stdc++.h>
#include<ext/rope>
using namespace std;
using namespace __gnu_cxx;
rope<int> s;
int main()
{
int n,m,l,e,i;
scanf("%d%d",&n,&m);
for(i=1;i<=n;i++){
s.push_back(i);
}
while(m--){
scanf("%d%d",&l,&e);
s=s.substr(l-1,e)+s.substr(0,l-1)+s.substr(l+e-1,n-e-(l-1));
}
for(i=0;i<s.size();i++){
if(i>0) printf(" ");
printf("%d",s[i]);
}
return 0;
}