Today , I will study the state and lifecycle hooks of React.
class Clock extends React.Component {
super(props);
this.state = {date: new Date()};
}
componentDidMount() {
this.timerID = setInterval(
() => this.tick(),
1000
);
}
componentWillUnmount() {
clearInterval(this.timerID);
}
tick() {
this.setState({
date: new Date()
});
}
render() {
return (
<div>
<h1>Hello, world!</h1>
<h2>It is {this.state.date.toLocaleTimeString()}.</h2>
</div>
);
}
}
ReactDOM.render(
<Clock />,
document.getElementById('root')
);
Let’s quickly recap what’s going on and the order in which the methods are called:
When
<Clock />
is passed toReactDOM.render()
, React calls the constructor of theClock
component. SinceClock
needs to display the current time, it initializesthis.state
with an object including the current time. We will later update this state.React then calls the
Clock
component’srender()
method. This is how React learns what should be displayed on the screen. React then updates the DOM to match theClock
’s render output.When the
Clock
output is inserted in the DOM, React calls thecomponentDidMount()
lifecycle hook. Inside it, theClock
component asks the browser to set up a timer to call the component’stick()
method once a second.Every second the browser calls the
tick()
method. Inside it, theClock
component schedules a UI update by callingsetState()
with an object containing the current time. Thanks to thesetState()
call, React knows the state has changed, and calls therender()
method again to learn what should be on the screen. This time,this.state.date
in therender()
method will be different, and so the render output will include the updated time. React updates the DOM accordingly.If the
Clock
component is ever removed from the DOM, React calls thecomponentWillUnmount()
lifecycle hook so the timer is stopped.
this.setState((prevState, props) => ({
counter: prevState.counter + props.increment
}));
====
// Correct
this.setState(function(prevState, props) {
return {
counter: prevState.counter + props.increment
};
});
You have to be careful about the meaning of this
in JSX callbacks. In JavaScript, class methods are not bound by default. If you forget to bind this.handleClick
and pass it to onClick
, this
will be undefined
when the function is actually called.
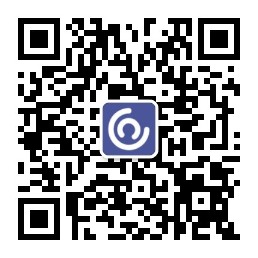
This is not React-specific behavior; it is a part of how functions work in JavaScript. Generally, if you refer to a method without ()
after it, such as onClick={this.handleClick}
, you should bind that method.