package com.utils.img;
import java.awt.Rectangle;
import java.awt.image.BufferedImage;
import java.io.*;
import java.nio.channels.FileChannel;
import java.util.Iterator;
import javax.imageio.ImageIO;
import javax.imageio.ImageReadParam;
import javax.imageio.ImageReader;
import javax.imageio.stream.ImageInputStream;
/**
* 等比例截图
*
* @author leo
*
*/
public class ImageUtils {
// 比例
private static int propX = 16;
private static int propY = 9;
/**
*
* @param inputFile
* @param outFile
* @throws IOException
*/
public static void cutJPG(String inputFile, String outFile) throws IOException {
int[] w_h = getImgW_H(inputFile);
int width = w_h[0];
int height = w_h[1];
// 计算截图的坐标位置
boolean prop = width / propX > height / propY;
int x = 0;
int y = 0;
// 图片过宽
if (prop) {
x = (width - height / propY * propX) / 2;
width = height / propY * propX;
} else {
// 图片过高
y = (height - width / propX * propY) / 2;
height = width / propX * propY;
}
ImageInputStream imageStream = null;
try {
Iterator<ImageReader> readers = ImageIO.getImageReadersByFormatName("jpg");
ImageReader reader = readers.next();
imageStream = ImageIO.createImageInputStream(new FileInputStream(inputFile));
reader.setInput(imageStream, true);
ImageReadParam param = reader.getDefaultReadParam();
System.out.println(reader.getWidth(0));
System.out.println(reader.getHeight(0));
Rectangle rect = new Rectangle(x, y, width, height);
param.setSourceRegion(rect);
BufferedImage bi = reader.read(0, param);
ImageIO.write(bi, "jpg", new FileOutputStream(outFile));
} finally {
imageStream.close();
}
}
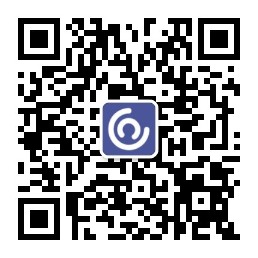
/**
* 获取图片的真实像素
*
* @param imgPath
* @return
*/
public static int[] getImgW_H(String imgPath) {
File file = new File(imgPath);
FileChannel fc = null;
if (file.exists() && file.isFile()) {
try {
@SuppressWarnings("resource")
FileInputStream fs = new FileInputStream(file);
fc = fs.getChannel();
System.out.println(fc.size() + "-----fc.size()");
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
System.out.println(file.length() + "-----file.length B");
System.out.println(file.length() * 1024 + "-----file.length kb");
BufferedImage bi = null;
try {
bi = ImageIO.read(file);
} catch (IOException e) {
e.printStackTrace();
}
int width = bi.getWidth();
int height = bi.getHeight();
System.out.println("宽:像素-----" + width + "高:像素" + height);
int[] result = { width, height };
return result;
}
public static void main(String[] args) throws Exception {
cutJPG("C:/data0/test.jpg", "C:/data0/test1.jpg");
}
}