1.在了解顺序表之前,首先知道结构体,通过结构体来定义一个顺序表
1)静态顺序表代码如下:
1 typedef int DataType; 2 3 #define MAX_SIZE (100) 4 5 typedef struct SeqList { 6 DataType array[MAX_SIZE]; 7 int size; // 1. 保存顺序表里已经存了的数据个数 8 // 2. 当前可用下标 9 } SeqList;
2)动态顺序表定义如下:
1 typedef int DataType; 2 3 #define INIT_CAPACITY (3) 4 5 typedef struct SeqListD { 6 DataType * parray; 7 int capacity; // 当前容量 等于 静态顺序表 MAX_SIZE 8 int size; // 同静态顺序表 9 } SeqListD;
2.动态顺序表的插入删除操作和静态顺序表的基本一样,无非就是多了一个扩容机制
a.插入数据
1)头插法: 每一次在顺序表的最前方插入数据,其他的数据后移
1 void SeqListPushFront(SeqList *pSeq, DataType data) 2 { 3 assert(pSeq); 4 5 // 特殊情况(满了) 6 if (pSeq->size >= MAX_SIZE) { 7 printf("满了\n"); 8 assert(0); 9 return; 10 } 11 12 // 现有数据整体往后搬移一格 13 // i 代表的是位置 14 for (int i = pSeq->size; i > 0; i--) { 15 // 前面是位置 后面是数据 16 pSeq->array[i] = pSeq->array[i - 1]; 17 } 18 19 // i 代表的是数据 20 /* 21 for (int i = pSeq->size - 1; i >= 0; i--) { 22 pSeq->array[i + 1] = pSeq->array[i]; 23 } 24 */ 25 26 // 插入 27 pSeq->array[0] = data; 28 pSeq->size++; 29 }
2)尾插法:顺序表的末尾增加数据,其他元素不用改变
1 void SeqListPushBack(SeqList *pSeq, DataType data) 2 { 3 assert(pSeq); 4 5 // 特殊情况(满了) 6 if (pSeq->size >= MAX_SIZE) { 7 printf("满了\n"); 8 assert(0); 9 return; 10 } 11 12 // 通常情况 13 pSeq->array[pSeq->size] = data; 14 pSeq->size++; 15 }
3)中间插入:最为普通的插入
1 void SeqListInsert(SeqList *pSeq, int pos, DataType data) 2 { 3 assert(pSeq); 4 assert(pos >= 0 && pos <= pSeq->size); 5 6 // 特殊情况(满了) 7 if (pSeq->size >= MAX_SIZE) { 8 printf("满了\n"); 9 assert(0); 10 return; 11 } 12 13 // 1. 数据搬移 14 // 1) 从后往前 2) i 取 数据 [size - 1, pos] 15 for (int i = pSeq->size - 1; i >= pos; i--) { 16 // 位置 数据 17 pSeq->array[i + 1] = pSeq->array[i]; 18 } 19 20 21 // 2. 插入 22 pSeq->array[pos] = data; 23 pSeq->size++; 24 }
以上所有插入操作只是以静态为例,动态操作只是在判断顺序表满了的时候自动扩容和静态不一样,以下将展示动态扩容的代码:
1 void ExpandIfRequired(SeqListD *pSeq) 2 { 3 // 扩容条件 4 if (pSeq->size < pSeq->capacity) { 5 // 没满 6 return; 7 } 8 9 // 扩容 10 pSeq->capacity *= 2; 11 12 // 1. 申请新空间 13 DataType *newArray = (DataType *)malloc(sizeof(DataType)* pSeq->capacity); 14 assert(newArray); 15 // 2. 数据搬移 16 for (int i = 0; i < pSeq->size; i++) { 17 newArray[i] = pSeq->parray[i]; 18 } 19 // 3. 释放老空间,关联新空间 20 free(pSeq->parray); 21 pSeq->parray = newArray; 22 }
扫描二维码关注公众号,回复:
2795396 查看本文章
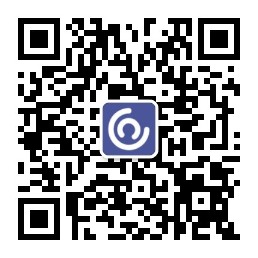