01.第一章:List集合_List接口介绍:
1).回顾Java的集合体系结构:
A).Collection(单列集合):
|--List:1).有序的;2).可以存储重复元素;
|--ArrayList(子类):无特有方法
|--LinkedList(子类):有一些特有方法,用于模拟栈、队列:
1).push():模拟压栈
2).poll():模拟弹栈
3).addFirst():添加到列头;
addLast():添加到列尾;
4).removeFirst():移除列头;
removeLast():移除列尾;
|--Set:1).无序的;2).不能存储重复元素;
|--HashSet(子类):哈希表实现:
|--LinkedHashSet(子类):Set的特例,有序的;
链表 + 哈希表实现
链表:保证存储元素的顺序;
哈希表:保证存储元素的唯一性;
B).Map(双列集合):
02.第一章:List集合_List接口中的常用方法:
1).增:
public void add(int index, E ele) : 将元素ele添加到index位置。
2).删:
public E remove(int index) : 移除列表中指定位置的元素, 返回的是被移除的元素。
3).改:
public E set(int index, E ele) :用指定元素替换集合中指定位置的元素,返回值的更新前的元素。
4).查:
public E get(int index) :返回集合中指定位置的元素。
注意:上面所有方法都有一个"index(索引)"参数;这个索引在使用时要保证在正确的范围,否则抛异常;
示例代码:
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("黄磊");
list.add("黄健翔");
list.add("黄渤");
System.out.println(list);
//在黄健翔的前面添加一个:孙红雷
list.add(3,"孙红雷");
System.out.println(list);
//移除“黄磊”
System.out.println("移除黄磊:" + list.remove(0));
System.out.println(list);
//将索引为:1的元素替换为:杨颖
System.out.println("索引为:1的元素替换为:杨颖:返回值 : " + list.set(1,"杨颖"));
System.out.println(list);
//获取索引为2的元素
System.out.println(list.get(2));
}
03.第二章:数据结构_概念及作用:
1).什么是“数据结构”:就是指“存储和管理”大量数据的一种方式;
2).“数据结构”的作用:每种数据结构都会影响到数据的排列方式,
从而影响增、删、改、查的执行效率;
04.第二章:数据结构_数组:
1).ArrayList集合的内部就是使用“数组”结构;
2).存储方式:
3).数组结构的特点:
1).查询快;增删慢;
2).内部存储是连续的;
3).不属于“先进先出”(属于根据索引随意出)
05.第二章:数据结构_链表:
1).LinkedList内部使用的是“链表结构”:
2).存储方式:
3).特点:
1).查询慢;增删快;
2).各个“节点”之间通过“地址”来连接;
06.第二章:数据结构_栈和队列:
1).“栈”结构:关注“存、取”的方式:
特点:
1).先进后出;
2).运算受限的线性表(只能在一端进行插入、删除)
3).只能在“栈顶”进行插入和删除;
2)."队列”结构:关注“存、取”的方式:
特点:
1).先进先出;(必须按顺序一个一个出,不能随意出)
2).运算受限的线性表(在一端插入,在另一端删除)
07.第二章:数据结构_红黑树:
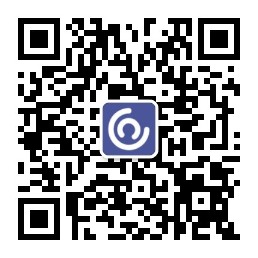
1).“树”结构:关注“存、取”的方式:
2).存储方式图示:
08.第三章:List的子类_ArrayList集合:
1).无特有方法:
2).内部数组实现(查询快;增删慢)
3).代码:
ArrayList<String> list = new ArrayList<>();
list.add("孙悟空");
list.add("曹操");
list.add("贾宝玉");
//遍历:方式一:迭代器(foreach)Collection接口
Iterator<String> it = list.iterator();
while(it.hasNext()){
System.out.println(it.next());
}
//遍历:方式二:get(index)List接口
for(int i = 0;i < list.size(); i++){
System.out.println(list.get(i));
}
09.第三章:List的子类_LinkedList集合:
1).有一些特有方法,可以模拟栈、队列;
1).addFirst():可以模拟压栈
2).addLast():可以模拟排队;
3).push():可以模拟压栈
4).pop():从列表的开头取出,并删除一个元素;可以模拟弹栈;
poll():从列表的开头取出,并删除一个元素;可以模拟弹栈;(如果列表为空,不抛异常,比较常用);
2).内部是“链表”实现;查询慢;增删快;
3).使用LinkedList模拟栈:
public class Demo {
public static void main(String[] args) {
LinkedList<String> list = new LinkedList<>();
//压栈
list.push("孙悟空");
list.push("猪八戒");
list.push("白骨精");
list.push("白龙马");
//弹栈
while (list.size() > 0) {
System.out.println("弹栈:" + list.poll());
}
}
}
10.第四章:Set接口_HashSet存储字符串:
public class Demo {
public static void main(String[] args) {
Set<String> set = new HashSet<>();
set.add("孙悟空");
set.add("猪八戒");
set.add("白龙马");
set.add("曹操");
String s = new String("白龙马");
set.add(s);//不存储重复元素
set.add("白龙马");//不存储重复元素
set.add("曹操");//不存储重复元素
for(String ss : set){
System.out.println(ss);//无序
}
}
}
11.第四章:Set接口_HashSet存储自定义对象:
public class Demo {
public static void main(String[] args) {
Set<Student> stuSet = new HashSet<>();
//Student类要重写hashCode()和equals()方法
stuSet.add(new Student("黄渤",18));
stuSet.add(new Student("黄晓明", 19));
stuSet.add(new Student("黄晓明", 19));
for (Student stu : stuSet) {
System.out.println(stu);
}
}
}
12.第四章:Set接口数据结构哈希表:
1).哈希表存储结构:
13.第四章:Set接口_LinkedHashSet的特点及使用:
1).LinkedHashSet是Set的特例:有序的
由链表 + 哈希表实现;
链表:保证元素的顺序;
哈希表:保证元素的唯一;
2).示例代码:
public class Demo {
public static void main(String[] args) {
Set<String> set = new LinkedHashSet<>();
set.add("孙悟空");
set.add("猪八戒");
set.add("白龙马");
set.add("曹操");
for (String s : set) {
System.out.println(s);//有序的
}
}
}
14.第四章:Set接口_可变参数
public class Demo {
public static void main(String[] args) {
sum(10, 20);
sum(10, 20, 3, 40, 532, 43, 24, 324, 324, 324, 324, 43);
p1();//不传实参
p1("43l","fdsaf","fsjklsdjfs","fsdf");//可以传任意多的实参
}
public static int sum(int... arr) {//语法糖:编译后:就是"数组"
int sum = 0;
for(int i = 0;i < arr.length; i++){
sum += arr[i];
}
return sum;
}
public static void p1(String ... arr){
}
}
说明:
1).可变参数可以是任何类型(基本类型、引用类型);
2).调用时,对于“可变的形参”,可以不传实参,也可以传任意多的实参;
3).在一个方法的形参列表中,最多只能有一个“可变参数”。
4).在一个方法的形参列表中,可以同时出现“可变参数”和“普通参数”,但“可变参数”必须位于参数列表的末尾。
5).“可变参数”编译后就是“数组”,在方法内部,按照数组处理即可。
15.第五章:Collections工具类_常用功能:
1).java.util.Collections(工具类):里面包含了一些对Collection集合操作的工具类
2).常用方法:
1).public static <T> boolean addAll(Collection<T> c, T. . . elements) :往集合中添加一些元素。
示例代码:
ArrayList<Integer> intList = new ArrayList<>();
Collections.addAll(intList,10,24,4324,2,432,4,24,324,32432,4);
System.out.println(intList);
2).public static void shuffle(List<?> list) 打乱顺序 :打乱集合顺序。
示例代码:
System.out.println("打乱顺序:");
Collections.shuffle(intList);
System.out.println(intList);
3).public static <T> void sort(List<T> list) :将集合中元素按照默认规则排序(自然排序,升序)。
基于被排序对象的:compareTo()方法(需要实现Comparable接口)
示例代码:
System.out.println("排序:");
Collections.sort(intList);
System.out.println(intList);
4).public static <T> void sort(List<T> list,Comparator<? super T>):将集合中元素按照指定规则排序。
使用比较器(Comparator)可以允许被排序对象不实现Comparable接口。但是要制作一个比较器(Comparator
的子类),具体例子见:17
16.第五章:Collections工具类实现比较方式一为类添加比较功能_Comparable接口:
1).java.util.Comparable接口:作用:添加“比较的方法”--compareTo()
2).Java类库中的一些常用类:String、Integer(各种包装类)都实现了这个接口,所以可以使用Collections工具类的sort()
方法进行排序。
3).要对“自定义类”进行排序,就需要实现Comparable接口,并实现compareTo()方法。
4).示例代码:
自定义对象:
public class Student implements Comparable {
String name;
int age;
......
@Override
public int compareTo(Object o) {
Student stu = (Student)o;
return this.age - stu.age;
}
}
测试类:
public static void main(String[] args) {
List<Student> stuList = new ArrayList<>();
stuList.add(new Student("张三",20));
stuList.add(new Student("李四",18));
stuList.add(new Student("李四2",17));
stuList.add(new Student("李四",19));
stuList.add(new Student("李四",13));
Collections.sort(stuList);//排序--内部调用Student的compareTo()方法。
System.out.println(stuList);
}
17.第五章:Collections工具类实现比较方式二比较器_Comparator接口:
public class Demo {
public static void main(String[] args) {
List<Student> stuList = new ArrayList<>();
stuList.add(new Student("jack",20));
stuList.add(new Student("rose",17));
stuList.add(new Student("anna",18));
stuList.add(new Student("anna",19));
stuList.add(new Student("tom",13));
Collections.sort(stuList,new Comparator(){//比较器
@Override
public int compare(Object o1, Object o2) {
Student stu1 = (Student)o1;
Student stu2 = (Student)o2;
//1.先按姓名排升序
int num = stu1.name.compareTo(stu2.name);
//2.如果姓名相同,按年龄降序排
int num2 = (num == 0 ? stu2.age - stu1.age : num);
return num2;
}
});
System.out.println(stuList);
}
}
学习目标总结:
01.能够说出List集合特点
1).有序的;
2).可以存储重复元素;
3).可以通过“索引”操作元素;
02.能够说出常见的数据结构
1).数组;
2).链表:
3).栈
4).队列;
5).树
6).哈希表;
03.能够说出数组结构特点
1).在内存中存储是“连续的”;
2).查询快;增删慢;
04.能够说出单向链表结构特点
1).查询慢;增删快;
2).通过相邻元素记录对方的地址实现;
05.能够说出栈结构特点
1).运算受限的线性表(只能在同一端操作插入、删除)
2).先进后出;
3).插入、删除都是在“栈顶”;
06.能够说出队列结构特点
1).运算受限的线性表;
2).先进先出;
3).插入、删除分别在两端;
07.能够说出Set集合的特点
1).无序的;
2).不能存储重复元素;
08.能够说出哈希表的特点
1).JDK8的哈希表实现:
数组 + 链表 + 红黑树
2).不存储重复元素
3).验证元素的唯一性:
先判断元素的hashCode():
不同:直接存;
相同:判断元素的equals()
不同:存
相同:不存;
09.使用HashSet集合存储自定义元素
Set stu = new HashSet<>();
注意:需要Student类重写hashCode()和equals()
10.能够说出可变参数的格式
public static void p1(int … 变量名){
1).可变参数编译后就是“数组”,所以可以按照数组进行操作;
2).方法形参列表中只能有一个“可变参数”,可以有普通参数,但可变参数必须位于参数列表的末尾;
}
11.能够使用集合工具类
Collections
1).addAll(集合,T….):
2).shuffer():打乱顺序:
3).sort(List):对集合内的元素排序。基于元素的compareTo()
4).sort(List,Comparator):对list元素进行排序。基于比较器Comparator的。
12.能够使用Comparator比较器进行排序
public class Demo {
public static void main(String[] args) {
List<Student> stuList = new ArrayList<>();
stuList.add(new Student("jack",20));
stuList.add(new Student("rose",17));
stuList.add(new Student("anna",18));
stuList.add(new Student("anna",19));
stuList.add(new Student("tom",13));
Collections.sort(stuList,new Comparator(){//比较器
@Override
public int compare(Object o1, Object o2) {
Student stu1 = (Student)o1;
Student stu2 = (Student)o2;
//1.先按姓名排升序
int num = stu1.name.compareTo(stu2.name);
//2.如果姓名相同,按年龄降序排
int num2 = (num == 0 ? stu2.age - stu1.age : num);
return num2;
}
});
System.out.println(stuList);
}
}