1. 包装类
(基本类型中没有多少我们能够使用的方法和属性,为了便捷我们需要自己去写)
针对每一种基本类型提供了对应的类的形式 --- 包装类
byte | short | int | long | float | double | char | boolean | void |
Byte | Short | Integer | Long | Float | Double | Character | Boolean | Void |
void 是一个最终类,不能被实例化。
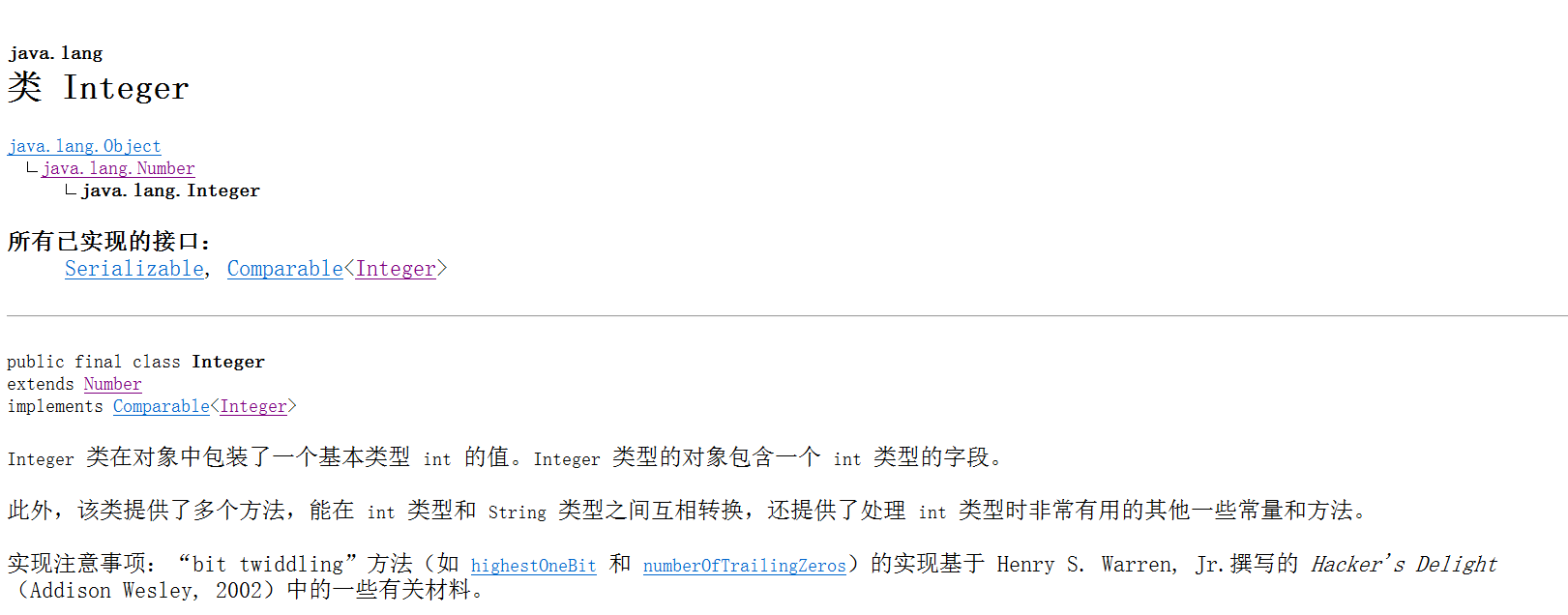
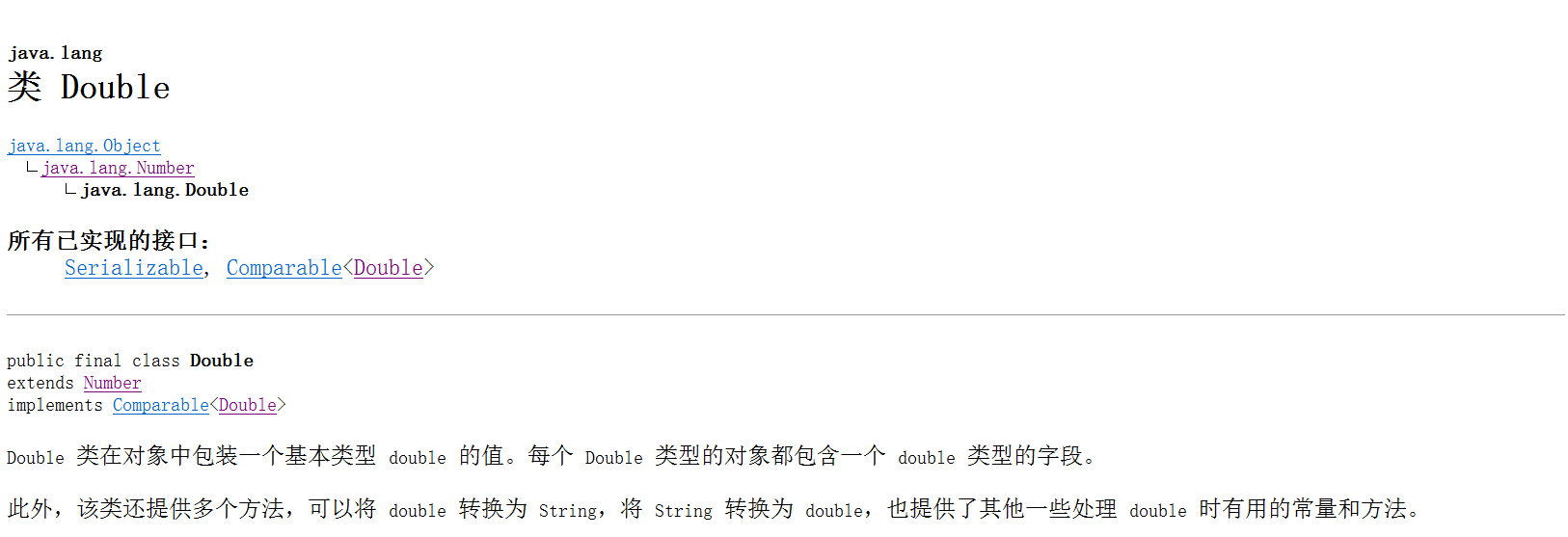
package cn.tedu.baozhuang; public class IntegerDemo1 { public static void main(String[] args) { int i = 7; //封箱 Integer in = new Integer(i); //自动封箱,都是 JDK1.5 的特性 //其实就是在底层默认调用 Interger 类身上的静态方法 valueOf,Double Boolean 这些也是这样的!! //Integer in2 = Integer.valueOf(i); Integer in2 = i; Integer in3 = new Integer(5); //自动拆箱,在底层调用对象身上的 IntegerValue() //in3.IntegerValue(); int i3 = in3; } }
当把基本类型的变量直接赋值给对应的引用类型的对象,--- 自动封箱,底层是调用了对应类身上的 valueOf() 方法
当把引用类型的对象直接赋值给对应的基本类型的变量,---自动拆箱,底层是调用了对应类身上的***Value() 方法,
package cn.tedu.baozhuang; public class IntegerDemo2 { public static void main(String[] args) { //Integer i1 = Integer.valueOf(); //如果这个值不在 -128~127 底层的 valueOf() 调用构造方法 new 一把,返回一个新对象!!! //如果在 -128~127,从 cache 数组中对应下标位置上取值。 Integer i1 = 50; Integer i2 = 50; System.out.println(i1 == i2); Integer in = 400; int i = 400; //包装类和基本类型比较时,会进行自动拆箱成基本类型 System.out.println(in == i); } }
四种整数型都有范围判断(-128~127),float 就没有,直接 new 了!
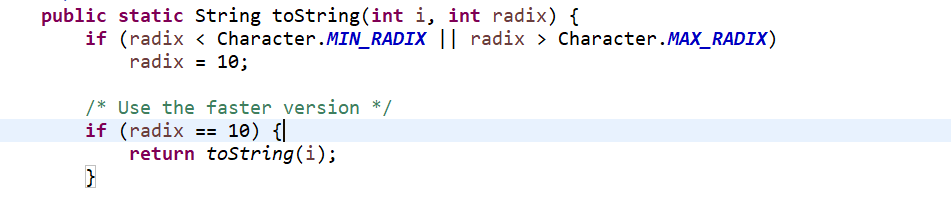
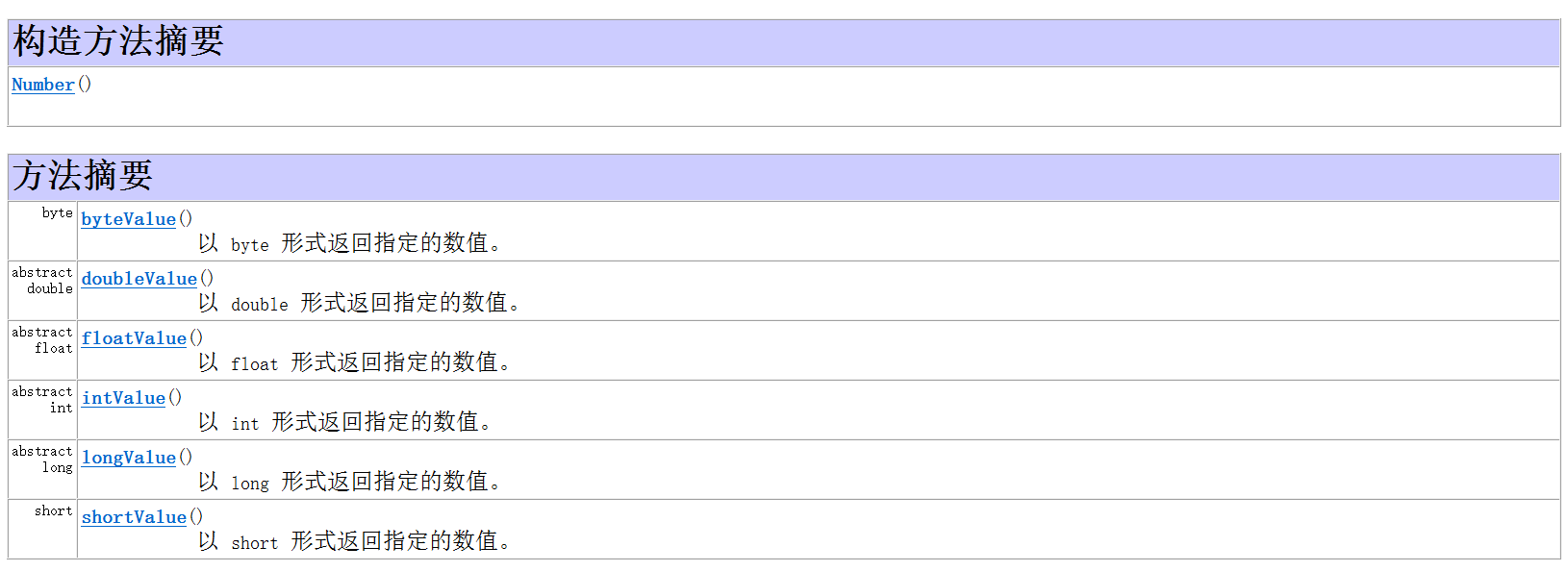
注意:凡是 Number 的子类都会提供将字符串转化为对应类型的构造方法


包装类产生的对象的哈希码是固定的,不随环境改变。
注意:字面量的哈希码不随环境不随系统而改变
2. 数学类
(是在代码中调用 Java 中写好的数学类)
Math --- 最终类,针对基本类型提供了基本的数学运算
BigDecimal --- 能够精确运算,要求参数以字符串形式传递
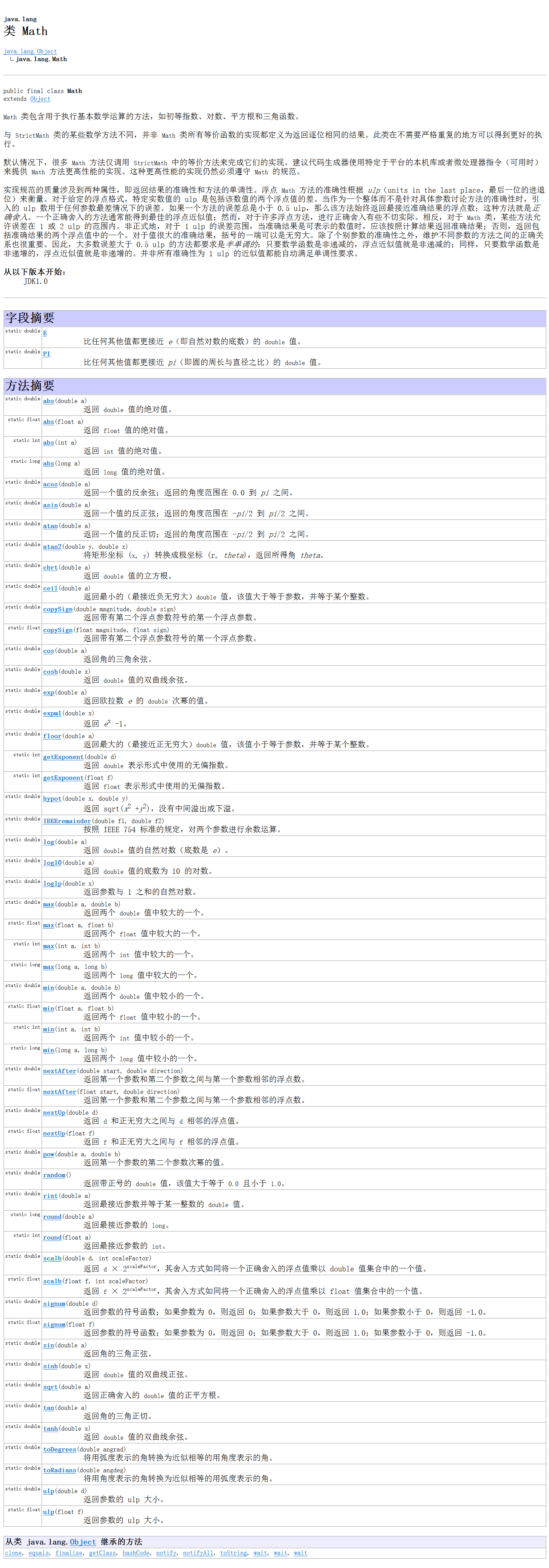
几个比较重要的方法对应如下代码:
package cn.tedu.math; import java.util.Random; public class MathDemo1 { public static void main(String[] args) { System.out.println(Math.abs(-5.86)); //求立方根 System.out.println(Math.cbrt(8)); //向上取整,返回一个double 类型的数据 System.out.println(Math.ceil(4.06)); //向下取整,都是返回double 类型的 System.out.println(Math.floor(4.06)); //四舍五入,返回一个 long 类型的 System.out.println(Math.round(3.68)); //返回一个[0,1)的一个随机小数 System.out.println(Math.random()); //获取 30-90 一个随机整数 System.out.println((int)(Math.random()*61+30)); //获取 0-30的数,只能是整数 Random r = new Random(); System.out.println(r.nextInt(30)); //抽奖系统,实际上要考虑权重问题(就是让那种经常到这里消费的人的概率高一些) if(Math.random() * Math.random() > 0.95){ System.out.println("亲,恭喜你中奖啦!"); } } }
两个很大的数相乘,用一个数组存放。
int[ ] arr1 = new int[ n ];
int[ ] arr2 = new int[ n ];
int[ ] result = new int[ 2*n ];
for(int i = 0; i < n; i++){
for(int j = 0; j < n; j++){
int r = arr1[i] * arr2[j] + result[ i+j ];//是一个暂时的数,用于后面分别存放,是i+j位的数(其实就是上一位的 i + j +1 位)
result[ i+j ] = r % 10; //表示第 i + j 位的结果
result[ i+j+1 ] = r / 10; //表示第 i + j + 1 位结果,用于表示进位
}
}