代码要求:
用户登录后,创建cookie保存用户信息
设置cookie的有效期为5分钟
在登录页循环遍历cookie数组,判断是否存在指定名称的cookie,若存在则直接跳转至欢迎页面
代码实现过程:登录页面输入用户名和密码,点击登陆后,连接数据库,并查找,如果有用户名和密码相同,则设置cookie,进入欢迎页面,并且,设置有效期5分钟的cookie,5分钟内进入登录页面直接跳转至欢迎页面!
登录页面代码:
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'userLogin.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<% Cookie[] cookies=request.getCookies();
if(cookies!=null){
for(int i=0;i<cookies.length;i++){
//cookie名与用户名设置cookie时的键比较
if(cookies[i].getName().equals("username")){
response.sendRedirect("welcome.jsp");
}
}
}
%>
<form action="<%=request.getContextPath()%>/jsp/Login/douser.jsp" method="post" >
<div>用户名:</div> <input type="text" name="username" value=""> <div id="namediv"></div>
<div>密码:</div> <input type="password" name="password" value=""> <div id="pwddiv"></div>
<input type="submit" value="登录">
</form>
</body>
</html>
页面如图:
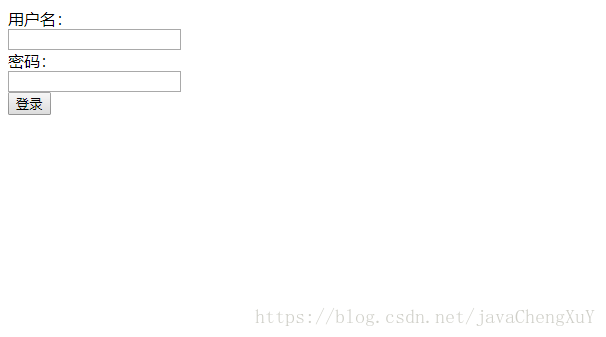
跳转页面代码:
<%@page import="java.net.URLEncoder"%>
<%@page import="cn.user.userEntity.User"%>
<%@page import="java.util.List"%>
<%@page import="cn.user.userServiceImpl.UserServiceImpl"%>
<%@page import="cn.user.userService.UserService"%>
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<%
request.setCharacterEncoding("UTF-8");
//获取客户端输入的用户名和密码
String username=request.getParameter("username");
//用户名转为utf-8格式,不转response.addCookie(nameCookie)处可能报错
String uName=URLEncoder.encode(username, "UTF-8");
String password=request.getParameter("password");
String str="欢迎光临:"+username;
//设置session,有效时间15分钟,将信息传递至欢迎页面
session.setAttribute("mess", str);
session.setMaxInactiveInterval(15*60);
//数据库调取的用户名和密码
String uname=null;
String pwd=null;
//调用java代码获取数据库中的用户名和密码
UserService service=new UserServiceImpl();
List<User> list =service.selectUser(username);
for(User user:list){
uname=user.getUsername();
pwd=user.getPassword();
}
if(username.trim().equals(uname) && password.trim().equals(pwd)){
//创建cookie
out.print(username);
Cookie nameCookie=new Cookie("username",uName.trim());
//cookie设置有效时间5分钟
nameCookie.setMaxAge(5*60);
response.addCookie(nameCookie);
Cookie pwdCookie =new Cookie("pwd",password.trim());
pwdCookie.setMaxAge(5*60);
response.addCookie(pwdCookie);
response.sendRedirect("welcome.jsp");
}
%>
连接数据库处java代码:
BaseDao 类:
package cn.user.userdao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class BaseDao {
Connection connection=null;
PreparedStatement pstmt=null;
ResultSet rs=null;
//连接数据库的方法
public boolean getConnection(){
try {
Class.forName("com.mysql.jdbc.Driver");
String url="jdbc:mysql://localhost:3306/users";
String user="root";
String password="abc12345";
connection=DriverManager.getConnection(url, user, password);
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false;
}
return true;
}
//数据库的增删改
public int executeSql(String sql,Object...params){
int updateRows=0;
if( this.getConnection()){
try {
pstmt=connection.prepareStatement(sql);
for(int i=0;i<params.length;i++){
pstmt.setObject(i+1, params[i]);
}
updateRows= pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
return updateRows;
}
//遍历数据库
public ResultSet selectsql(String sql,Object[] params){
if(this.getConnection()){
try {
pstmt=connection.prepareStatement(sql);
for(int j=0;j<params.length;j++){
pstmt.setObject(j+1, params[j]);
}
rs=pstmt.executeQuery();
} catch (SQLException e) {
e.printStackTrace();
}
}
return rs;
}
//关闭资源
public boolean closeSource(){
if(rs!=null){
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
return false;
}
}
if(pstmt!=null){
try {
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
return false;
}
}
if(connection!=null){
try {
connection.close();
} catch (SQLException e) {
e.printStackTrace();
return false;
}
}
return true;
}
}
// UserDao接口
package cn.user.userdao;
import java.util.List;
import cn.user.userEntity.User;
public interface UserDao {
public boolean addInfo(User user);
public boolean selectName(String name);
public boolean selectEmail(String email);
//此处为上述jsp页面连接数据库,调用数据库搜索用户信息的方法
public List<User> selectUser(String username);
}
// 实用类继承BaseDao,并实现UserDao
package cn.user.userdaoImpl;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import cn.user.userEntity.User;
import cn.user.userdao.BaseDao;
import cn.user.userdao.UserDao;
//UserDao实现类
public class UserDaoImpl extends BaseDao implements UserDao {
//插入数据路方法
public boolean addInfo(User user) {
boolean flag=false;
String sql="INSERT INTO users(id,username,PASSWORD,email,phone) VALUES (NULL,?,?,?,?)";
Object[] params={user.getUsername(),user.getPassword(),user.getEmail(),user.getPhone()};
//调用增删改方法
int i= this.executeSql(sql, params);
if(i>0){
flag=true;
}
return flag;
}
public boolean selectName(String name){
boolean flag=false;
String sql="SELECT username FROM users WHERE username=?";
Object[]params={name};
ResultSet rs=this.selectsql(sql, params);
try {
if(rs.next()){
flag=true;
}
} catch (SQLException e) {
e.printStackTrace();
}
this.closeSource();
return flag;
}
public boolean selectEmail(String email) {
boolean flag=false;
String sql="SELECT email FROM users WHERE email=?";
Object[]params={email};
ResultSet rs=this.selectsql(sql, params);
try {
if(rs.next()){
flag=true;
}
} catch (SQLException e) {
e.printStackTrace();
}
this.closeSource();
return flag;
}
//此处为上述jsp页面连接数据库,调用数据库搜索用户信息的方法
public List<User> selectUser(String username){
User user=null;
List<User> list=new ArrayList<User>();
String sql="SELECT username,password FROM users WHERE username=?";
Object[]params={username};
ResultSet rs=this.selectsql(sql, params);
System.out.println("1");
try {
if(rs.next()){
user=new User();
String userName=rs.getString("username");
String password=rs.getString("password");
user.setUsername(userName);
user.setPassword(password);
list.add(user);
}
} catch (SQLException e) {
e.printStackTrace();
}
this.closeSource();
return list;
}
}
// UserService接口
package cn.user.userService;
import java.util.List;
//服务类接口
import cn.user.userEntity.User;
public interface UserService {
public boolean addinfo(User user);
public boolean selectName(String name);
public boolean selectEmail(String email);
//此处为上述jsp页面连接数据库,调用数据库搜索用户信息的方法
public List<User> selectUser(String username);
}
// UserService接口的实现类
package cn.user.userServiceImpl;
//服务实现类
import java.util.List;
import cn.user.userEntity.User;
import cn.user.userService.UserService;
import cn.user.userdao.UserDao;
import cn.user.userdaoImpl.UserDaoImpl;
public class UserServiceImpl implements UserService{
private UserDao userDao;
public UserServiceImpl(){
userDao=new UserDaoImpl();
}
public boolean addinfo(User user) {
boolean flag=userDao.addInfo(user);
return flag;
}
public boolean selectName(String name) {
return userDao.selectName(name);
}
public boolean selectEmail(String email) {
return userDao.selectEmail(email);
}
//此处为上述jsp页面连接数据库,调用数据库搜索用户信息的方法
public List<User> selectUser(String username){
return userDao.selectUser(username);
}
}
//实体类
package cn.user.userEntity;
public class User {
private int id;
private String username;
private String password;
private String email;
private String phone;
public User(String username, String password, String email,
String phone) {
this.username = username;
this.password = password;
this.email = email;
this.phone = phone;
}
public User(int id, String username, String password, String email,
String phone) {
super();
this.id = id;
this.username = username;
this.password = password;
this.email = email;
this.phone = phone;
}
public User() {
super();
// TODO Auto-generated constructor stub
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
}
调用数据库代码结束
欢迎页面代码:
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'MyJsp.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
<script >
function exit(){
window.location="jsp/Login/userLogin.jsp";
}
</script>
</head>
<body>
<%=session.getAttribute("mess").toString() %>
<% //设置cookie为5分钟,所以单击退出之后,立马还进入欢迎页面
%>
<button type="button" onclick="exit();" >退出</button>
</body>
</html>
欢迎页面如图:
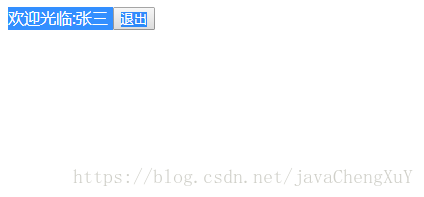
欢迎光临:张三是用session传递过来,有效时间15分钟,cookie5分钟时间过期后,点击退出,跳转到登录页面。
登录页面设置cookie,用户登录后,创建cookie保存用户信息
猜你喜欢
转载自blog.csdn.net/javaChengXuY/article/details/81783124
今日推荐
周排行