问题
给定一个由整数组成的非空数组所表示的非负整数,在该数的基础上加一。
最高位数字存放在数组的首位, 数组中每个元素只存储一个数字。
你可以假设除了整数 0 之外,这个整数不会以零开头。
输入: [1,2,3]
输出: [1,2,4]
解释: 输入数组表示数字 123。
输入: [4,3,2,1]
输出: [4,3,2,2]
解释: 输入数组表示数字 4321。
Given a non-empty array of digits representing a non-negative integer, plus one to the integer.
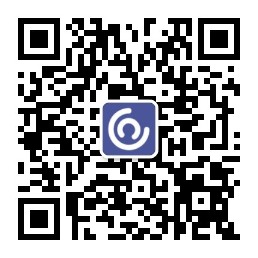
The digits are stored such that the most significant digit is at the head of the list, and each element in the array contain a single digit.
You may assume the integer does not contain any leading zero, except the number 0 itself.
Input: [1,2,3]
Output: [1,2,4]
Explanation: The array represents the integer 123.
Input: [4,3,2,1]
Output: [4,3,2,2]
Explanation: The array represents the integer 4321.
示例
public class Program {
public static void Main(string[] args) {
int[] nums = { 1, 3, 5, 6, 9 };
var res = PlusOne(nums);
ShowArray(res);
Console.ReadKey();
}
private static void ShowArray(int[] array) {
foreach(var num in array) {
Console.Write($"{num} ");
}
Console.WriteLine();
}
private static int[] PlusOne(int[] digits) {
for(int i = digits.Length - 1; i >= 0; i--) {
if(++digits[i] != 10) {
return digits;
} else {
digits[i] = 0;
if(i == 0) {
//增加数组长度,扩容
int[] newDigits = new int[digits.Length + 1];
for(int j = 0; j < digits.Length; j++) {
newDigits[j + 1] = digits[j];
}
newDigits[0] = 1;
return newDigits;
}
}
}
return null;
}
}
以上给出1种算法实现,以下是这个案例的输出结果:
1 3 5 7 0
分析:
在最坏的情况下,我们讨论第29行代码的执行情况,显然需要执行 次,即以上算法的时间复杂度为:
。