C++
#include< algorithm>
1. nique()
unique()函数是一个去重函数,STL中unique的函数 unique的功能是去除相邻的重复元素(只保留一个),还有一个容易忽视的特性是它并不真正把重复的元素删除。因为unique去除的是相邻的重复元素,所以一般用之前都会要排一下序
#include<iostream>
#include<algorithm>
using namespace std;
int main()
{
int a[]= {1,2,2,3,3,4,4,8,8,5};
int *m=unique(a,a+10);
int k=m-a;
for(int i=0; i<k; i++)
{
cout<<a[i]<<endl;
}
return 0;
}
2. count()
返回某个值出现的次数
#include<iostream>
#include<algorithm>
using namespace std;
int main()
{
int a[]= {1,2,2,3,3,4,4,8,8,5,2};
cout<<count(a, a+11, 2)<<endl;
return 0;
}
3. find()
查找函数
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
int m[]= {35,5,5,9,3,7,10};
int n=7;
if (find(m,m+n,10) == m+n-1)
cout << "yes" << endl;
else
cout << "no" << endl;
cout<<find(m,m+n,10)-m<<endl;//在数组中第一次出现的位置的数组下标
}
4. reverse(s.begin(),s.end())
反转字符串
#include<iostream>
#include<algorithm>
using namespace std;
int main ()
{
string s;
s="123456";
reverse(s.begin(),s.end());
cout<<s;
return 0;
}
6. swap()
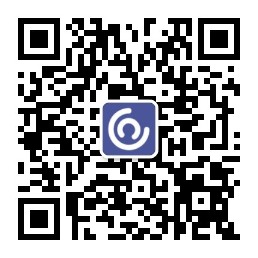
交换两个变量的值
#include<iostream>
#include<algorithm>
using namespace std;
int main ()
{
int x=123;
int y=321;
swap(x,y);
cout<<x<<" "<<y<<endl;
return 0;
}
7. sort()
序列排序(升序、降序),sort(start,end,排序方法),若无排序方法默认升序排列 sort(start,end)
#include<iostream>
#include<algorithm>
using namespace std;
int main()
{
int a[]= {9,6,3,8,5,2,7,4,1,0};
for(int i=0; i<10; i++)
cout<<a[i];
cout<<endl;
sort(a,a+10);//升序
for(int i=0; i<10; i++)
cout<<a[i];
cout<<endl;
return 0;
}
#include<iostream>
#include<algorithm>
using namespace std;
bool cp(int a,int b)
{
return a>b;//升序排列,如果改为return a<b,则为升序
}
int main()
{
int a[]= {9,6,3,8,5,2,7,4,1,0};
for(int i=0; i<10; i++)
cout<<a[i];
cout<<endl;
sort(a,a+10,cp);//降序
for(int i=0; i<10; i++)
cout<<a[i];
cout<<endl;
return 0;
}
// bool类型函数
其调用方式和int 型函数没有太大的区别。bool型变量的值只有 真 (true) 和假 (false)。bool可用于定义函数类型为布尔型,函数里可以有 return true、return false语句。
#include<iostream>
using namespace std;
bool cmp(int a,int b)
{
if(a>b)
return true;
else
return false;
}
int main()
{
int a = 5;
int b = 6;
if(cmp(a,b))
cout << "a>b" << endl;
else
cout << "a<b" << endl;
return 0;
}
8.max() min()
两个值中较大的max()——#include< iostream>
两个值中较小的min()——#include< iostream>
序列中的最小元素min_element()——返回的是地址 ,在#include< algorithm>里
序列中的最大元素max_element()——返回的是地址, 在#include< algorithm>里
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
int m[]= {35,5,5,9,3,7,10};
cout<<max(35,5)<<endl;
cout<<min(35,5)<<endl;
cout<<max_element(m,m+7)-m<<endl;
cout<<min_element(m,m+7)-m<<endl;//输出数组下标
}
C/C++
- strcmp()——比较字符串s1和s2,区分字母的大小写,#include< string.h>
设这两个字符串为str1,str2,
若str1==str2,则返回零;
若str1 < str2,则返回负数;
若str1 > str2,则返回正数。
- strcat()——连接两个字符串
- strstr()
strstr (str1,str2) ,函数用于判断字符串str2是否是str1的子串。如果是,则该函数返回str2在str1中首次出现的地址;否则,返回NULL
#include <iostream>
#include <string.h>
using namespace std;
int main()
{
char a[]="123abcf45";
char b[]="cf4";
char c[]="cp";
cout<<strstr(a,b)<<endl;
cout<<strstr(a,c)<<endl;
return 0;
}
- memset()——初始化,语法:memset(a,0,sizeof (a) )
- getchar()
从缓冲区读走一个字符,相当于清除缓冲区。前面的scanf()在读取输入时会在缓冲区中留下一个字符’\n’(输入完s[i]的值后按回车键所致),所以如果不在此加一个getchar()把这个回车符取走的话,gets()就不会等待从键盘键入字符,而是会直接取走这个“无用的”回车符,从而导致读取有误
vector容器
begin()——返回一个当前vector容器中起始元素的迭代器。
end()——返回一个当前vector容器中末尾元素的迭代器。
front()——返回当前vector容器中起始元素的引用。
back()——返回当前vector容器中末尾元素的引用。
优先队列**——priority_queue<结构类型> 队列名
头文件:#include< queue>
语法:priority_queue<结构类型> 队列名,默认为降序
————priority_queue< int,vector,less >q; //降序
————priority_queue< int,vector,greater >q; //升序
#include <iostream>
#include <cstdio>
#include <queue>
using namespace std;
priority_queue <int,vector<int>,less<int> > p;
priority_queue <int,vector<int>,greater<int> > q;
int a[5]= {10,12,14,6,8};
int main()
{
for(int i=0; i<5; i++)
p.push(a[i]),q.push(a[i]);
while(!p.empty())
printf("%d ",p.top()),p.pop();
printf("\n");
while(!q.empty())
printf("%d ",q.top()),q.pop();
printf("\n");
return 0;
}
//运行结果:14 12 10 8 6
// 6 8 10 12 14
双端队列——deque<结构类型>队列名
双端队列(deque,全名double-ended queue)是一个限定插入和删除操作的数据结构,具有队列和栈的性质。双端队列中的元素可以从两端弹出,其限定插入和删除操作在表的两端进行。
基本操作:
插入
双端队列不仅提供了push_back成员函数,还提供了push_front成员函数,从而可以在序列的前后两端进行插入操作。
对于双端队列,在序列的两端插入元素的时间复杂度均为常数,在中间插入元素的时间复杂度与插入点到最近序列端点的距离成正比。并且,对于双端队列的插入操作(包括在双端插入和中间插入),将会使所有的迭代器失效,只能采用下标操作
删除
双端队列不仅提供了pop_back成员函数,还提供了pop_front成员函数,从而可以在序列的前后两端进行删除操作。在中间的删除操作将会使所有迭代器失效;而在两端的删除操作,仅当删除位置就是迭代器所指向位置时会使迭代器失效
SDUT 1466
#include <bits/stdc++.h>
using namespace std;
int main()
{
int n,x,i,m=0,a[10001];
string s;
deque<int>q;
cin>>n;
for(i=1; i<=n; i++)
{
cin>>s;
if(s=="LIN")
{
cin>>x;
q.push_front(x);
}
else if(s=="RIN")
{
cin>>x;
q.push_back(x);
}
else if(s=="LOUT")
{
if(q.empty()) a[m++]=i;
else q.pop_front();
}
else if(s=="ROUT")
{
if(q.empty()) a[m++]=i;
else q.pop_back();
}
else a[m++]=i;
}
while(!q.empty())
{
cout<<q.front()<<" ";
q.pop_front();
}
cout<<endl;
for(i=0; i<m; i++)
cout<<a[i]<<" "<<"ERROR"<<endl;
return 0;
}
余生还请多多指教!