Allocating memory on the heap
#include <stdlib.h>
void *calloc(size_t numitems , size_t size );
Returns pointer to allocated memory on success, or NULL on error
Example:
struct { /* Some field definitions */ } myStruct;
struct myStruct *p;
p = calloc(1000, sizeof(struct myStruct));
if (p == NULL)
// error;
#include <stdlib.h>
void *realloc(void * ptr , size_t size );
Returns pointer to allocated memory on success, or NULL on error
The call realloc(ptr, 0) is equivalent to calling free(ptr) followed by malloc(0). If
ptr is specified as NULL , then realloc() is equivalent to calling malloc(size).
Since realloc() may relocate the block of memory, we must use the returned
pointer from realloc() for future references to the memory block. We can employ
realloc() to reallocate a block pointed to by the variable ptr as follows:
nptr = realloc(ptr, newsize);
if (nptr == NULL) {
/* Handle error */
} else {
/* realloc() succeeded */
ptr = nptr;
}
--------------------------------------------------------------------------------------------------------------------------------
Allocating memory on the stack
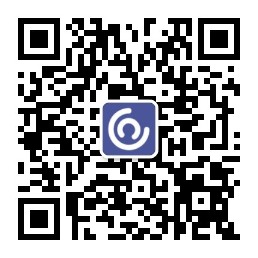
#include <alloca.h>
void *alloca(size_t size );
Returns pointer to allocated block of memory ----- allocates memory dynamically
If the stack overflows as a consequence of calling alloca(), then program behavior is
unpredictable. In particular, we don’t get a NULL return to inform us of the error.
(In fact, in this circumstance, we may receive a SIGSEGV signal.)
Advantage of alloca() is that the memory that it allocates is automati-
cally freed when the stack frame is removed; that is, when the function that called
alloca() returns.