概要
1.currentThread(); 返回对当前正在执行的线程对象的引用。
2.getId(); 返回该线程的标识符。
3.getName(); 返回该线程的名称。
4.getPriority(); 返回线程的优先级。
5.getState(); 返回该线程的状态。
6.interrupt(); 中断线程。
7.interrupted(); 测试当前线程是否已经中断。
8.isAlive(); 测试线程是否处于活动状态。
9.isDaemon(); 测试该线程是否为守护线程。
10.isInterrupted(); 测试线程是否已经中断。
11.join(); 等待该线程终止。
12.setDaemon(boolean on); 将该线程标记为守护线程或用户线程。
13.setName(String name); 改变线程名称,使之与参数 name 相同。
14.setPriority(int newPriority); 更改线程的优先级。
15.sleep(long millis); 在指定的毫秒数内让当前正在执行的线程休眠(暂停执行),
16.start(); 使该线程开始执行;Java 虚拟机调用该线程的 run 方法。
17.stop(); 已过时。
18.yield(); 暂停当前正在执行的线程对象,并执行其他线程。
1.currentThread();
package mythread;
public class CountOperate extends Thread {
public CountOperate() {
System.out.println("CountOperate---begin");
System.out.println("Thread.currentThread().getName()="
+ Thread.currentThread().getName());
System.out.println("this.getName()=" + this.getName());
System.out.println("CountOperate---end");
}
@Override
public void run() {
System.out.println("run---begin");
System.out.println("Thread.currentThread().getName()="
+ Thread.currentThread().getName());
System.out.println("this.getName()=" + this.getName());
System.out.println("run---end");
}
}
package test;
import mythread.CountOperate;
public class Run {
public static void main(String[] args) {
CountOperate c = new CountOperate();
Thread t1 = new Thread(c);
t1.setName("A");
t1.start();
}
}
运行结果:
CountOperate---begin
Thread.currentThread().getName()=main
this.getName()=Thread-0
CountOperate---end
run---begin
Thread.currentThread().getName()=A
this.getName()=Thread-0
run---end
注意:
Thread.currentThread()和this的区别!!
2.isAlive();
package mythread;
public class MyThread extends Thread {
@Override
public void run() {
System.out.println("run=" + this.isAlive());
}
}
package run;
import mythread.MyThread;
public class Run {
public static void main(String[] args) {
MyThread mythread = new MyThread();
System.out.println("begin ==" + mythread.isAlive());
mythread.start();
Thread.sleep(1000);//让main线程睡眠,是为了让myhtread线程run方法跑完,再运行main方法的print方法
System.out.println("end ==" + mythread.isAlive());
}
}
运行结果:
begin ==false
end ==true
run=false
注意:
isAlive()作用是测试线程是否处于活动状态。
活动状态就是线程已启动且尚未终止。
3.停止线程
三种方式:
使用退出标志,使线程正常退出,也就是当run方法完成后线程终止。
stop(); // 不安全的,且弃用的方法。
interrupt();// 尽管叫停止/终止,实际 不会终止一个正在运行的线程。
3.1 interrupt();
package exthread;
public class MyThread extends Thread {
@Override
public void run() {
super.run();
for (int i = 0; i < 500000; i++) {
System.out.println("i=" + (i + 1));
}
}
}
package test;
import exthread.MyThread;
public class Run {
public static void main(String[] args) {
try {
MyThread thread = new MyThread();
thread.start();
Thread.sleep(2000);//睡眠是阻止main线程立即调用thread的interrupt()方法;
thread.interrupt();
} catch (InterruptedException e) {
System.out.println("main catch");
e.printStackTrace();
}
}
}
运行结果:
i=1
1=2
..
i=500000
说明:
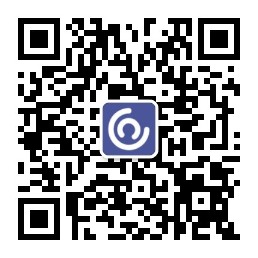
尽管执行了interrupt()方法,但是没有像for-break那样立刻停止,还是会继续执行run()方法。
调用该方法的线程的状态为将被置为”中断”状态。
注意:线程中断仅仅是置线程的中断状态位,不会停止线程。需要用户自己去监视线程的状态为并做处理。支持线程中断的方法(也就是线程中断后会抛出interruptedException的方法)就是在监视线程的中断状态,一旦线程的中断状态被置为“中断状态”,就会抛出中断异常。