1. 保留小数点后两位
/** * 返回含有精确到百分位的小数 * @param str * @return */ public static String getDecimalFormat2(String str) { DecimalFormat decimalFormat = new DecimalFormat("0.00"); String decimals = decimalFormat.format(Float.parseFloat(str)); decimalFormat = null; return decimals; } /** * 返回含有精确到百分位的小数 * @param nf * @return */ public static String getFormat2(float nf){ return String.format("%.2f", nf); }
2. 手机号验证
/** * 验证手机号码 * @param pho 手机号 * @return */ public static boolean checkPhone(String pho) { Pattern p = Pattern.compile("^(13[0-9]|14[579]|15[0-3,5-9]|16[6]|17[0135678]|18[0-9]|19[89])\\d{8}$");
return p.matcher(pho).matches(); }
3. 用户名验证
3.1 支持字母、数字、下划线、横线、[6-12]字符
/** * 验证用户名 * * @param password 由数字、26个英文字母或下划线组成的字符串 * @return boolean */ public static boolean isOE(String password) { Pattern pattern = Pattern.compile("^[0-9a-zA-Z_-]{6,12}$"); return pattern.matcher(password).matches(); }
3.2 支持字母、数字、[6-12]字符
4. 验证邮箱
/** * 验证邮箱 * * @param email * @return boolean */ public static boolean isEmail(String email) { Pattern pattern = Pattern.compile("[\\w!#$%&'*+/=?^_`{|}~-]+(?:\\.[\\w!#$%&'*+/=?^_`{|}~-]+)*@(?:[\\w](?:[\\w-]*[\\w])?\\.)+[\\w](?:[\\w-]*[\\w])?"); return pattern.matcher(email).matches(); }
扫描二维码关注公众号,回复:
3278132 查看本文章
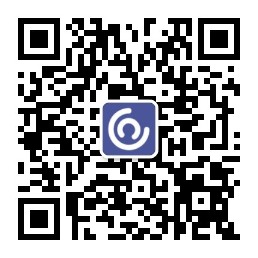
5. 验证日期
/** * 验证日期 * * @param date yyyy-mm-dd (考虑平闰年) * @return boolean */ public static boolean isDate(String date) { Pattern pattern = Pattern.compile("^(?:(?!0000)[0-9]{4}-(?:(?:0[1-9]|1[0-2])-(?:0[1-9]|1[0-9]|2[0-8])"
+ "|(?:0[13-9]|1[0-2])-(?:29|30)|(?:0[13578]|1[02])-31)|(?:[0-9]{2}(?:0[48]|[2468][048]|[13579][26])"
+ "|(?:0[48]|[2468][048]|[13579][26])00)-02-29)$"); Matcher matcher = pattern.matcher(date); return matcher.matches(); }
6. 身份证验证
/** * 身份证号码验证 * @param idCard 身份证 * @return */ public static boolean IDCardValidate(String idCard) { String[] ValCodeArr = {"1", "0", "x", "9", "8", "7", "6", "5", "4", "3", "2"}; String[] Wi = {"7", "9", "10", "5", "8", "4", "2", "1", "6", "3", "7", "9", "10", "5", "8", "4", "2"}; String si = ""; try { // 号码的长度为15位或18位 if (idCard.length() != 15 && idCard.length() != 18) { return false; } // 除最后一位都是数字 if (idCard.length() == 18) { si = idCard.substring(0, 17); } // 判断[0-16]否是数字 Pattern pattern = Pattern.compile("[0-9]*"); Matcher isNum = pattern.matcher(si); if (!isNum.matches()) return false; // 出生年月是否有效 String strYear = si.substring(6, 10);// 年份 String strMonth = si.substring(10, 12);// 月份 String strDay = si.substring(12, 14);// 月份 String regxStr = "^((\\d{2}(([02468][048])|([13579][26]))[\\-\\/\\s]?((((0?[13578])|(1[02]))"
+ "[\\-\\/\\s]?((0?[1-9])|([1-2][0-9])|(3[01])))|(((0?[469])|(11))[\\-\\/\\s]?((0?[1-9])"
+ "|([1-2][0-9])|(30)))|(0?2[\\-\\/\\s]?((0?[1-9])|([1-2][0-9])))))|"(\\d{2}(([02468]"
+ "[1235679])|([13579][01345789]))[\\-\\/\\s]?((((0?[13578])|(1[02]))[\\-\\/\\s]?((0?"
+ "[1-9])|([1-2][0-9])"|(3[01])))|(((0?[469])|(11))[\\-\\/\\s]?((0?[1-9])|([1-2][0-9])"
+ "|(30)))|(0?2[\\-\\/\\s]?((0?[1-9])|(1[0-9])|(2[0-8]))))))(\\s(((0?[0-9])|([1-2][0-3]))"
+ "\\:([0-5]?[0-9])((\\s)|(\\:([0-5]?[0-9])))))?$"; Pattern pattern1 = Pattern.compile(regxStr); Matcher isNo = pattern1.matcher(strYear + "-" + strMonth + "-" + strDay); if (!isNo.matches()) { return false; } GregorianCalendar gc = new GregorianCalendar(); SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); if ((gc.get(Calendar.YEAR) - Integer.parseInt(strYear)) > 150 || (gc.getTime().getTime() - sdf.parse(strYear + "-" + strMonth + "-" + strDay).getTime()) < 0) { return false; } if (Integer.parseInt(strMonth) > 12 || Integer.parseInt(strMonth) == 0) { return false; } if (Integer.parseInt(strDay) > 31 || Integer.parseInt(strDay) == 0) { return false; } // 判断地区编码 String areaCode = "11,12,13,14,15,21,22,23,31,32,33,34,35,36,37,41,42" + "43,44,45,46,50,51,52,53,54,61,62,63,64,65,71,81,82,91"; if (!areaCode.contains(si.substring(0, 2))) return false; // 判断最后一位的值 int TotalmulAiWi = 0; for (int i = 0; i < 17; i++) { char ch = si.charAt(i); TotalmulAiWi += (ch - '0') * Integer.parseInt(Wi[i]); } int modValue = TotalmulAiWi % 11; String strVerifyCode = ValCodeArr[modValue]; si = si + strVerifyCode; if (idCard.length() == 18) { if (!si.equalsIgnoreCase(idCard)) { return false; } } } catch (Exception e) { return false; } return true; }
7. 统一社会信用代码
/** * 统一社会信用代码 * * @param businessCode 信用代码 * @return */ public static boolean isValidCode(String businessCode) { if (TextUtils.isEmpty(businessCode) || businessCode.length() != 18) { return false; } String baseCode = "0123456789ABCDEFGHJKLMNPQRTUWXY"; char[] baseCodeArray = baseCode.toCharArray(); Map<Character, Integer> codes = new HashMap<>(); for (int i = 0; i < baseCode.length(); i++) { codes.put(baseCodeArray[i], i); } char[] businessCodeArray = businessCode.toCharArray(); Character check = businessCodeArray[17]; if (baseCode.indexOf(check) == -1) { return false; } int[] wi = {1, 3, 9, 27, 19, 26, 16, 17, 20, 29, 25, 13, 8, 24, 10, 30, 28}; int sum = 0; for (int i = 0; i < 17; i++) { Character key = businessCodeArray[i]; if (baseCode.indexOf(key) == -1) { return false; } sum += (codes.get(key) * wi[i]); } int value = 31 - sum % 31; return value == codes.get(check); }
8. MD5加密
/** * 利用MD5进行加密 * * @param str 待加密的字符串 * @return 加密后的字符串 */ public static String encoderByMd5(String str) { try { // 生成一个MD5加密计算摘要 MessageDigest md = MessageDigest.getInstance("MD5"); // 计算md5函数 md.update(str.getBytes()); // digest()最后确定返回md5 hash值,返回值为8为字符串。因为md5 hash值是16位的hex值,实际上就是8位的字符 // BigInteger函数则将8位的字符串转换成16位hex值,用字符串来表示;得到字符串形式的hash值 return new BigInteger(1, md.digest()).toString(16); } catch (Exception e) { e.printStackTrace(); return null; } }
9. 验证中文
/** * 验证中文 * * @param textString 字符串 * @return boolean */ public static boolean isChinese(String textString) { Pattern pattern = Pattern.compile("[\u4e00-\u9fa5]"); return pattern.matcher(textString).matches(); }