FlycoTabLayout简介
FlycoTabLayout是一个Android TabLayout库,目前包含了3个TabLayout,分别是:
1、SlidingTabLayout(依赖于ViewPager一起使用)
主要用于页面顶部导航Tab的实现,参照PagerSlidingTabStrip进行大量修改,新增了部分属性,支持多种风格的指示器显示,支持未读消息数和小红点显示,可以实现的效果如下图所示:
2、CommonTabLayout(不依赖ViewPager可以与其他控件自由搭配使用)
相比于SlidingTabLayout新增了对于Icon图标及其位置的支持,所以我们可以用它来搭建首页底部Tab导航栏,效果如下图:
3、SegmentTabLayout(不依赖ViewPager可以与其他控件自由搭配使用)
仿照QQ消息列表头部tab切换的控件,可以实现的效果如下图:
ViewPager+Fragment+FlycoTabLayout实现的例子
当然了CommonTabLayout,SegmentTabLayout还可以和ViewPager外的其他控件搭配使用,比如FrameLayout。
1,编写布局文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tl="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tablayout"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="?attr/colorPrimary"
tl:tl_divider_color="#1AFFFFFF"
tl:tl_divider_padding="13dp"
tl:tl_divider_width="1dp"
tl:tl_indicator_color="#FFFFFF"
tl:tl_indicator_height="1.5dp"
tl:tl_indicator_width_equal_title="true"
tl:tl_tab_padding="22dp"
tl:tl_tab_space_equal="true"
tl:tl_textSelectColor="#FFFFFF"
tl:tl_textUnselectColor="#66FFFFFF"
tl:tl_underline_color="#1AFFFFFF"
tl:tl_underline_height="1dp" />
<android.support.v4.view.ViewPager
android:id="@+id/view_pager"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
2.MainActivity代码实现
public class MainActivity extends AppCompatActivity {
@InjectView(R.id.tablayout)
SlidingTabLayout tablayout;
@InjectView(R.id.view_pager)
ViewPager viewPager;
private ArrayList<BaseFragment> mFagments = new ArrayList<>();
private String[] mTitles = {"首页", "消息", "联系人", "更多"};
private MyPagerAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
}
private void initView() {
mFagments.add(new Fragment01());
mFagments.add(new Fragment02());
mFagments.add(new Fragment03());
mFagments.add(new Fragment04());
// getChildFragmentManager() 如果是嵌套在fragment中实现就要用这个
// 在activity中FragmentManager通过getSupportFragmentManager()去获取,如果在是在fragment中就需要通过getChildFragmentManager()去说去
adapter = new MyPagerAdapter(getSupportFragmentManager());
viewPager.setAdapter(adapter);
tablayout.setViewPager(viewPager, mTitles);
}
private class MyPagerAdapter extends FragmentPagerAdapter {
public MyPagerAdapter(FragmentManager fm) {
super(fm);
}
@Override
public int getCount() {
return mFagments.size();
}
@Override
public CharSequence getPageTitle(int position) {
return mTitles[position];
}
@Override
public Fragment getItem(int position) {
return mFagments.get(position);
}
}
}
3.1 一般BaseFragment代码如下:
public abstract class BaseFragment extends Fragment {
private View mRootView;
protected Activity mActivity;
private Unbinder bind;
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
this.mActivity = getActivity();
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
mRootView = inflater.inflate(getContentViewId(),container,false);
bind = ButterKnife.bind(this, mRootView);
return mRootView;
}
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
initData();
}
protected void initData(){}
protected abstract int getContentViewId();
@Override
public void onDestroyView() {
super.onDestroyView();
bind.unbind(); // 解绑butterknife
}
}
3.2 具有懒加载功能的BaseFragment代码如下:
public abstract class BaseLazyLoadFragment extends Fragment {
private boolean isFirstLoad = false;
protected View view;
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, Bundle savedInstanceState) {
view = inflater.inflate(setLayoutResourceID(),container,false);//让子类实现初始化视图
initView();//初始化事件
isFirstLoad = true;//视图创建完成,将变量置为true
if (getUserVisibleHint()) {//如果Fragment可见进行数据加载
onLazyLoad();
isFirstLoad = false;
}
return view;
}
@Override
public void onDestroyView() {
super.onDestroyView();
isFirstLoad = false;//视图销毁将变量置为false
}
@Override
public void setUserVisibleHint(boolean isVisibleToUser) {
super.setUserVisibleHint(isVisibleToUser);
if (isFirstLoad && isVisibleToUser) {//视图变为可见并且是第一次加载
onLazyLoad();
isFirstLoad = false;
}
}
//数据加载接口,留给子类实现
public abstract void onLazyLoad();
//初始化视图接口,子类必须实现
public abstract int setLayoutResourceID();
//初始化事件接口,留给子类实现
public void initView(){ }
}
4.分别继承以上任意一种BaseFragment,实现Fragment01.java,Fragment02.java,Fragment03.java,Fragment04.java即可。
官方Demo中的案例效果布局文件:
分别对应简介中的三张图示效果
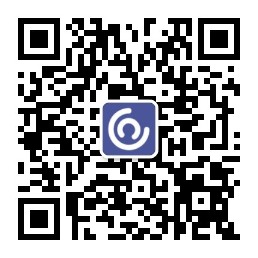
图三:
<ScrollView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tl="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#eeeeee"
android:scrollbars="none">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<com.flyco.tablayout.SegmentTabLayout
android:id="@+id/tl_1"
android:layout_width="wrap_content"
android:layout_height="32dp"
android:layout_gravity="center_horizontal"
android:layout_marginTop="10dp"
tl:tl_bar_color="#ffffff"
tl:tl_indicator_color="#2C97DE"
tl:tl_indicator_corner_radius="8dp"
tl:tl_tab_padding="20dp"/>
<com.flyco.tablayout.SegmentTabLayout
android:id="@+id/tl_2"
android:layout_width="wrap_content"
android:layout_height="36dp"
android:layout_gravity="center_horizontal"
android:layout_marginTop="10dp"
android:paddingLeft="10dp"
android:paddingRight="10dp"
tl:tl_indicator_anim_enable="true"
tl:tl_indicator_bounce_enable="false"
tl:tl_indicator_color="#009688"
tl:tl_tab_padding="20dp"/>
<com.flyco.tablayout.SegmentTabLayout
android:id="@+id/tl_3"
android:layout_width="match_parent"
android:layout_height="36dp"
android:layout_marginTop="10dp"
android:paddingLeft="25dp"
android:paddingRight="25dp"
tl:tl_bar_color="#ffffff"
tl:tl_indicator_anim_enable="true"
tl:tl_indicator_color="#F6CE59"
tl:tl_indicator_margin_bottom="2dp"
tl:tl_indicator_margin_left="2dp"
tl:tl_indicator_margin_right="2dp"
tl:tl_indicator_margin_top="2dp"
tl:tl_textBold="SELECT"/>
<com.flyco.tablayout.SegmentTabLayout
android:id="@+id/tl_4"
android:layout_width="wrap_content"
android:layout_height="36dp"
android:layout_gravity="center_horizontal"
android:paddingLeft="10dp"
android:paddingRight="10dp"
tl:tl_bar_color="#ffffff"
tl:tl_indicator_color="#EC7263"
tl:tl_indicator_margin_bottom="2dp"
tl:tl_indicator_margin_left="2dp"
tl:tl_indicator_margin_right="2dp"
tl:tl_indicator_margin_top="2dp"
tl:tl_tab_width="80dp"
tl:tl_textBold="BOTH"/>
<com.flyco.tablayout.SegmentTabLayout
android:id="@+id/tl_5"
android:layout_width="match_parent"
android:layout_height="36dp"
android:layout_gravity="center_horizontal"
android:paddingLeft="30dp"
android:paddingRight="30dp"
tl:tl_indicator_anim_enable="true"
tl:tl_indicator_bounce_enable="false"
tl:tl_indicator_margin_bottom="2dp"
tl:tl_indicator_margin_left="2dp"
tl:tl_indicator_margin_right="2dp"
tl:tl_indicator_margin_top="2dp"
tl:tl_tab_space_equal="true"/>
</LinearLayout>
</ScrollView>
图一:
<ScrollView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tl="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#eeeeee"
android:scrollbars="none">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tl_1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#666666"
android:paddingBottom="15dp"
android:paddingTop="15dp"
tl:tl_indicator_gravity="TOP"
tl:tl_textBold="SELECT"
tl:tl_underline_color="#1A000000"
tl:tl_underline_gravity="TOP"
tl:tl_underline_height="1dp"/>
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tl_2"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#EEEEEE"
tl:tl_divider_color="#1A000000"
tl:tl_divider_padding="13dp"
tl:tl_divider_width="1dp"
tl:tl_indicator_color="#000000"
tl:tl_indicator_height="1.5dp"
tl:tl_indicator_width_equal_title="true"
tl:tl_tab_padding="22dp"
tl:tl_tab_space_equal="true"
tl:tl_textSelectColor="#000000"
tl:tl_textUnselectColor="#66000000"
tl:tl_underline_color="#1A000000"
tl:tl_underline_height="1dp"/>
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tl_3"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#3F9FE0"
tl:tl_textAllCaps="true"
tl:tl_textBold="BOTH"
tl:tl_textsize="14sp"/>
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tl_4"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#009688"
tl:tl_tab_padding="0dp"
tl:tl_tab_width="95dp"
tl:tl_textsize="15sp"/>
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tl_5"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#F6CE59"
tl:tl_indicator_width="10dp"/>
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tl_6"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#EC7263"
tl:tl_indicator_corner_radius="2dp"
tl:tl_indicator_height="4dp"
tl:tl_indicator_width="4dp"/>
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tl_7"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#57385C"
tl:tl_indicator_corner_radius="1.5dp"
tl:tl_indicator_height="3dp"
tl:tl_indicator_width="10dp"/>
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tl_8"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#E45171"
tl:tl_indicator_color="#eeeeee"
tl:tl_indicator_style="TRIANGLE"/>
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tl_9"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#6D8FB0"
android:paddingLeft="5dp"
android:paddingRight="5dp"
tl:tl_indicator_margin_left="2dp"
tl:tl_indicator_margin_right="2dp"
tl:tl_indicator_style="BLOCK"/>
<com.flyco.tablayout.SlidingTabLayout
android:id="@+id/tl_10"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#222831"
android:paddingLeft="5dp"
android:paddingRight="5dp"
tl:tl_indicator_color="#393E46"
tl:tl_indicator_corner_radius="5dp"
tl:tl_indicator_margin_left="2dp"
tl:tl_indicator_margin_right="2dp"
tl:tl_indicator_style="BLOCK"/>
</LinearLayout>
</ScrollView>
图二:
<ScrollView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tl="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#eeeeee"
android:scrollbars="none">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<com.flyco.tablayout.CommonTabLayout
android:id="@+id/tl_1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#ffffff"
android:paddingBottom="5dp"
android:paddingTop="5dp"
tl:tl_indicator_color="#2C97DE"
tl:tl_textSelectColor="#2C97DE"
tl:tl_textUnselectColor="#66000000"
tl:tl_underline_color="#DDDDDD"
tl:tl_underline_height="1dp"/>
<com.flyco.tablayout.CommonTabLayout
android:id="@+id/tl_2"
android:layout_width="match_parent"
android:layout_height="54dp"
android:background="#ffffff"
tl:tl_iconHeight="23dp"
tl:tl_iconWidth="23dp"
tl:tl_indicator_color="#2C97DE"
tl:tl_indicator_height="0dp"
tl:tl_textSelectColor="#2C97DE"
tl:tl_textUnselectColor="#66000000"
tl:tl_textsize="12sp"
tl:tl_underline_color="#DDDDDD"
tl:tl_underline_height="1dp"/>
<com.flyco.tablayout.CommonTabLayout
android:id="@+id/tl_3"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#ffffff"
tl:tl_iconGravity="LEFT"
tl:tl_iconHeight="18dp"
tl:tl_iconMargin="5dp"
tl:tl_iconWidth="18dp"
tl:tl_indicator_bounce_enable="false"
tl:tl_indicator_color="#2C97DE"
tl:tl_indicator_gravity="TOP"
tl:tl_textSelectColor="#2C97DE"
tl:tl_textUnselectColor="#66000000"
tl:tl_textsize="15sp"
tl:tl_underline_color="#DDDDDD"
tl:tl_underline_gravity="TOP"
tl:tl_underline_height="1dp"/>
<com.flyco.tablayout.CommonTabLayout
android:id="@+id/tl_4"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#F6CE59"
tl:tl_iconVisible="false"
tl:tl_textBold="SELECT"
tl:tl_indicator_width="10dp"
tl:tl_textsize="14sp"/>
<com.flyco.tablayout.CommonTabLayout
tl:tl_textBold="BOTH"
android:id="@+id/tl_5"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#EC7263"
tl:tl_iconVisible="false"
tl:tl_indicator_corner_radius="2dp"
tl:tl_indicator_height="4dp"
tl:tl_indicator_width="4dp"
tl:tl_textsize="14sp"/>
<com.flyco.tablayout.CommonTabLayout
android:id="@+id/tl_6"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#57385C"
tl:tl_iconVisible="false"
tl:tl_indicator_corner_radius="1.5dp"
tl:tl_indicator_height="3dp"
tl:tl_indicator_width="10dp"
tl:tl_textsize="14sp"/>
<com.flyco.tablayout.CommonTabLayout
android:id="@+id/tl_7"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#E45171"
tl:tl_iconVisible="false"
tl:tl_indicator_color="#eeeeee"
tl:tl_indicator_corner_radius="1.5dp"
tl:tl_indicator_height="3dp"
tl:tl_indicator_style="TRIANGLE"
tl:tl_indicator_width="10dp"
tl:tl_textsize="14sp"/>
<com.flyco.tablayout.CommonTabLayout
android:id="@+id/tl_8"
android:layout_width="match_parent"
android:layout_height="48dp"
android:background="#6D8FB0"
android:paddingLeft="5dp"
android:paddingRight="5dp"
tl:tl_iconVisible="false"
tl:tl_indicator_margin_left="5dp"
tl:tl_indicator_margin_right="5dp"
tl:tl_indicator_style="BLOCK"
tl:tl_textsize="14sp"/>
</LinearLayout>
</ScrollView>
属性列表:
tl_indicator_color color 设置显示器颜色
tl_indicator_height dimension 设置显示器高度
tl_indicator_width dimension 设置显示器固定宽度
tl_indicator_margin_left dimension 设置显示器margin,当indicator_width大于0,无效
tl_indicator_margin_top dimension 设置显示器margin,当indicator_width大于0,无效
tl_indicator_margin_right dimension 设置显示器margin,当indicator_width大于0,无效
tl_indicator_margin_bottom dimension 设置显示器margin,当indicator_width大于0,无效
tl_indicator_corner_radius dimension 设置显示器圆角弧度
tl_indicator_gravity enum 设置显示器上方(TOP)还是下方(BOTTOM),只对常规显示器有用
tl_indicator_style enum 设置显示器为常规(NORMAL)或三角形(TRIANGLE)或背景色块(BLOCK)
tl_underline_color color 设置下划线颜色
tl_underline_height dimension 设置下划线高度
tl_underline_gravity enum 设置下划线上方(TOP)还是下方(BOTTOM)
tl_divider_color color 设置分割线颜色
tl_divider_width dimension 设置分割线宽度
tl_divider_padding dimension 设置分割线的paddingTop和paddingBottom
tl_tab_padding dimension 设置tab的paddingLeft和paddingRight
tl_tab_space_equal boolean 设置tab大小等分
tl_tab_width dimension 设置tab固定大小
tl_textsize dimension 设置字体大小
tl_textSelectColor color 设置字体选中颜色
tl_textUnselectColor color 设置字体未选中颜色
tl_textBold boolean 设置字体加粗
tl_iconWidth dimension 设置icon宽度(仅支持CommonTabLayout)
tl_iconHeight dimension 设置icon高度(仅支持CommonTabLayout)
tl_iconVisible boolean 设置icon是否可见(仅支持CommonTabLayout)
tl_iconGravity enum 设置icon显示位置,对应Gravity中常量值,左上右下(仅支持CommonTabLayout)
tl_iconMargin dimension 设置icon与文字间距(仅支持CommonTabLayout)
tl_indicator_anim_enable boolean 设置显示器支持动画(only for CommonTabLayout)
tl_indicator_anim_duration integer 设置显示器动画时间(only for CommonTabLayout)
tl_indicator_bounce_enable boolean 设置显示器支持动画回弹效果(only for CommonTabLayout)
tl_indicator_width_equal_title boolean 设置显示器与标题一样长(only for SlidingTabLayout)
FlycoRoundView库的使用
一个扩展原生控件支持圆角矩形框背景的库,可以减少相关shape资源文件使用,支持TextView,FrameLayout,LinearLayout,RelativeLayout,更多使用也可以自己扩展。
通过观察源码,主要有如下几个类
RoundFrameLayout
RoundLinearLayout
RoundRelativeLayout
RoundTextView
并且FlycoTabLayout库中已经依赖了该库,如果使用了FlycoTabLayout,就可以直接使用该库,如果是单独使用则要先添加依赖:
Gradle
dependencies{
compile 'com.flyco.roundview:FlycoRoundView_Lib:1.1.4@aar'
}
效果如下:
实现以上的效果只需要如下,几个简单的自定义属性:
<com.flyco.roundview.RoundTextView
android:id="@+id/rtv_3"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:layout_marginBottom="10dp"
android:ellipsize="end"
android:gravity="center"
android:paddingBottom="10dp"
android:paddingLeft="18dp"
android:paddingRight="18dp"
android:paddingTop="10dp"
android:singleLine="true"
android:text="TextView Radius 10dpTextView Radius 10dp"
android:textColor="#383838"
rv:rv_backgroundColor="#F6CE59"
rv:rv_cornerRadius="10dp" />
支持属性 Attributes: