字符串操作
s为字符串
s.isalnum() 所有字符都是数字或者字母
s.isalpha() 所有字符都是字母
s.isdigit() 所有字符都是数字
s.islower() 所有字符都是小写
s.isupper() 所有字符都是大写
s.istitle() 所有单词都是首字母大写,像标题
s.isspace() 所有字符都是空白字符
s.index(x) 返回x所在索引,如:s.index(max(s))返回第一个最大值索引
判断是整数还是浮点数
a=123
b=123.123
>>>isinstance(a,int)
True
>>>isinstance(b,float)
True
>>>isinstance(b,int)
False
注意:在Python中,字符串是不可变的,因此无法就地更改字符。
L
Out[20]: 'hgfdsa'
L[1]
Out[21]: 'g'
L[1]="k"
Traceback (most recent call last):
File "<ipython-input-22-dedefc94809c>", line 1, in <module>
L[1]="k"
TypeError: 'str' object does not support item assignment
反转字符串
L="asdfgh"
LL = list(L)
LL.reverse()
L = "".join(LL)
L
Out[19]: 'hgfdsa'
string中的index和find
find和C中的strstr函数一样,s1.find(s2)返回s2作为s1的子字符串的起始位置,若干不存在返回-1,如果s2为空返回0。
index除了不能处理不存在的情况,其他和find一样
List操作
将两个list合并为一个list:
list3 = list1+list2 #其中元素不能含有Notetype类型
求list、ndarray众数
from scipy import stats
mode(a, axis=0, nan_policy='propagate')
Return an array of the modal (most common) value in the passed array.
If there is more than one such value, only the smallest is returned.
The bin-count for the modal bins is also returned.
Parameters
----------
a : array_like
n-dimensional array of which to find mode(s).
axis : int or None, optional
Axis along which to operate. Default is 0. If None, compute over
the whole array `a`.
nan_policy : {'propagate', 'raise', 'omit'}, optional
Defines how to handle when input contains nan. 'propagate' returns nan,
'raise' throws an error, 'omit' performs the calculations ignoring nan
values. Default is 'propagate'.
Returns
-------
mode : ndarray
Array of modal values.
count : ndarray
Array of counts for each mode.
Examples
--------
a = np.array([[6, 8, 3, 0],
[3, 2, 1, 7],
[8, 1, 8, 4],
[5, 3, 0, 5],
[4, 7, 5, 9]])
from scipy import stats
stats.mode(a) #默认是axis=0,即求出各列的众数,若有出现次数相同的数字,则输出值小的那个
(array([[3, 1, 0, 0]]), array([[1, 1, 1, 1]]))#前一个array是众数,后一个array是对应众数出现的次数
To get mode of whole array, specify ``axis=None``:
stats.mode(a, axis=None) #axis=None为求包含矩阵中所有项的众数
(array([3]), array([3]))
List排序
第一种:内建方法sort()
可以直接对列表进行排序
用法:
list.sort(func=None, key=None, reverse=False(or True))
对于reverse这个bool类型参数,当reverse=False时:为正向排序;当reverse=True时:为反向排序。默认为False。
执行完后会改变原来的list,如果你不需要原来的list,这种效率稍微高点
为了避免混乱,其会返回none
例如:
>>> list = [2,8,4,6,9,1,3]
>>> list.sort()
>>> list
[1, 2, 3, 4, 6, 8, 9]
第二种:内建函数sorted()
sorted()也可以对字符串进行排序,字符串默认按照ASCII大小来比较。
这个和第一种的差别之处在于:
sorted()不会改变原来的list,而是会返回一个新的已经排序好的list
list.sort()方法仅仅被list所定义,sorted()可用于任何一个可迭代对象
用法:
sorted(list)
该函数也含有reverse这个bool类型的参数,当reverse=False时:为正向排序(从小到大);当reverse=True时:为反向排序(从大到小)。当然默认为False。
执行完后会有返回一个新排序好的list
例如:
>>> list = [2,8,4,1,5,7,3]
>>> other = sorted(list)
>>> other
[1, 2, 3, 4, 5, 7, 8]
>>> other = sorted(list,reverse=True) #sort也是如此
>>> other
[8, 7, 5, 4, 3, 2, 1]
自定义排序
import functools
L = [2,1,4,9,3,3]
def reversed_cmp(x,y):
if x>y:
return -1
if x<y:
return 1
return 0
print(sorted(L,key=functools.cmp_to_key(reversed_cmp)))
out[1]:[9, 4, 3, 3, 2, 1]
compare(x,y)传入两个待比较的元素 x, y,如果 x 应该排在 y 的前面,返回 -1,如果 x 应该排在 y 的后面,返回 1。如果 x 和 y 相等,返回 0。python3中需要用functools.cmp_to_key,不像python2中直接甩一个cmp函数在后面就行了。
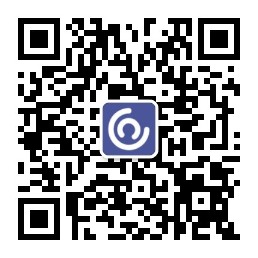
赋值时子List中用L[:]和L的区别
将多维List中的List赋值给另一个变量的时候,如果用append、pop方法改变这个变量,那么原来的List也会改变,如果赋值时用[:]形式则不会改变。但是直接对变量赋值运算不会改变原来List。
##没有用[:]形式
L = [[1],[2]]
for i in L:
x = i
x.append(0)
print(L)
out[1]:
[[1, 0], [2]]
[[1, 0], [2, 0]]
##用的[:]形式
L = [[1],[2]]
for i in L:
x = i[:]
x.append(0)
print(L)
out[2]:
[[1], [2]]
[[1], [2]]
##直接对x等号赋值
L = [[1],[2]]
for i in L:
x = i
x = [6]
print(L)
out[3]:
[[1], [2]]
[[1], [2]]
在for循环中L和L[:]的区别
##in L的话,会不断用更新后的L再次进行for循环
L = [1]
for i in L:
print(L)
L.append(1)
out[1]:
[1]
[1, 1]
[1, 1, 1]
[1, 1, 1, 1]
[1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
……………………
##用了L[:]
L = [1]
for i in L[:]:
print(L)
L.append(1)
out[2]:
[1]
数值操作
对数值取整的几种方法
四舍五入:
这里round并不总是四舍五入,在4.5的时候会得到去尾法的结果;另外round有两个参数,第二个参数用来控制精度,默认是0(即取整);
num = 4.6
round(num)
Out[40]: 5
去尾法:
num = 4.9
int(num)
Out[36]: 4
进一法:
import math
num = 4.1
math.ceil(num)
Out[35]: 5