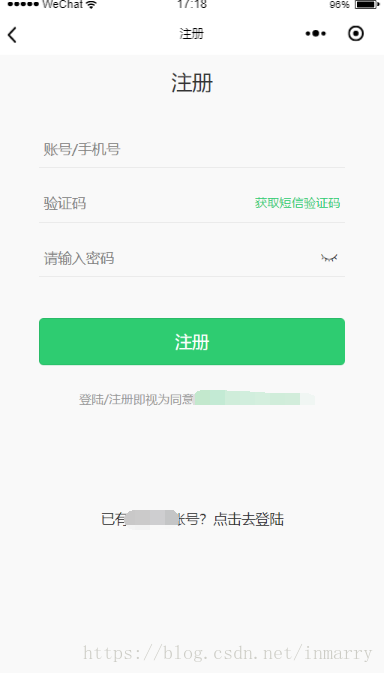
1、在page里面设置默认data:
var util = require('../../utils/MD5.js')
let app = getApp();
let myreg = /^(14[0-9]|13[0-9]|15[0-9]|17[0-9]|18[0-9])\d{8}$$/;
let timeOut = 60;
Page({
/**
* 页面的初始数据
*/
data: {
judgePage: 1,
userAccount: "",
userPassword: "",
codeNum: "",
tipsCode: "获取短信验证码",
timeNum: 60,
clikType: false,
userPhoto: "http://cyparty-project.img-cn-beijing.aliyuncs.com/hb/files/1507686525979_icon_avatar.png",
userName: "莘莘学子",
canIUse: wx.canIUse('button.open-type.getUserInfo'),
// subscriberId:wx.getStorageSync('userDataBase')
},
2、获取手机验证码:
//获取验证码时候显示时间
getTime() {
let that = this;
let timer = setInterval(function() {
that.data.timeNum--;
if (that.data.timeNum <= 0) {
that.setData({
tipsCode: "重新获取验证码",
timeNum: timeOut,
clikType: false
});
clearInterval(timer);
} else {
that.setData({
tipsCode: "重新发送" + that.data.timeNum + "秒",
})
}
}, 1000)
},
//输入手机号获取验证码
gainCode() {
let that = this;
if (this.data.userAccount == '') {
wx.showToast({
title: '手机号不能为空',
icon: 'none',
})
} else if (!myreg.test(this.data.userAccount)) {
wx.showToast({
title: '请输入正确的手机号',
icon: 'none',
})
} else {
that.setData({
clikType: true
})
if (this.data.timeNum < timeOut) {
} else {
wx.request({
url: "https://www.mddyg.com:9040/api/v2/doctor/getCode",
data: {
mobile: this.data.userAccount
},
method: "post",
header: {
'content-type': 'application/x-www-form-urlencoded',
},
success: function(res) {
that.getTime();
}
})
}
}
},
// 点击获取手机验证码
clickGainCode(e) {
if (!this.data.clikType) {
this.gainCode();
}
},
3、验证注册登陆:
// 监听输入账号
accountInput(e) {
// console.log(e)
this.setData({
userAccount: e.detail.value,
})
},
//监听输入密码
passwordInput(e) {
// console.log(e)
this.setData({
userPassword: e.detail.value,
})
},
//监听输入验证码
codeInput(e) {
this.setData({
codeNum: e.detail.value,
})
},
//验证注册登陆
onGotUserInfo(e) {
// console.log(e)
let that = this;
if (that.data.userAccount == "") {
wx.showToast({
title: '账号不能为空',
icon: 'none',
})
} else if (that.data.codeNum == "" && that.data.judgePage == 0) {
wx.showToast({
title: '验证码不能为空',
icon: 'none',
})
} else if (that.data.userPassword == "") {
wx.showToast({
title: '密码不能为空',
icon: 'none',
})
} else if (that.data.judgePage == 0) {
that.setData({
userPassword: util.hexMD5(that.data.userPassword)
})
wx.request({
url: app.globalData.url + "/api/personal/add",
data: {
subscriber_account: that.data.userAccount,
subscriber_password: that.data.userPassword,
subscriber_name: that.data.userName,
subscriber_photo: that.data.userPhoto,
},
method: "post",
header: {
'content-type': 'application/x-www-form-urlencoded'
},
success: function(res) {
that.wxGrant();
}
})
} else if (that.data.judgePage == 1) {
let that = this;
that.setData({
userPassword: util.hexMD5(that.data.userPassword)
})
wx.request({
url: app.globalData.url + "/api/login/check",
data: {
subscriber_account: that.data.userAccount,
subscriber_password: that.data.userPassword,
// subscriber_id: that.data.userDataBase.subscriberId
},
method: "post",
header: {
'content-type': 'application/x-www-form-urlencoded'
},
success: function(res) {
// console.log(res);
// console.log(1111)
if (res.data.status == false) {
wx.showToast({
title: '账号不存在',
icon: 'none'
})
} else {
wx.setStorageSync('userDataBase', res.data.result.data[0])
that.wxGrant();
}
}
})
}
},
4、register.wxml 布局文件:
<!--pages/registerPage/register.wxml-->
<view class="registe_container">
<view class="registe_box">
<view class="registe_name">{{judgePage==0?"注册":"登录"}}</view>
</view>
<view class='message_box'>
<view class='message_account registe_message_box'>
<input class='message_account_num' type='text' placeholder="账号/手机号" name="userName" bindinput="accountInput"></input>
</view>
<view class="message_code registe_message_box {{judgePage==0?'show':'hide'}}">
<input class='message_code_num' type='text' password="false" placeholder="验证码" name="codeNum" bindinput="codeInput"></input>
<view class="message_code_gain" bindtap="clickGainCode">{{tipsCode}}</view>
<view class='clear'></view>
</view>
<view class='message_password registe_message_box'>
<input class='message_password_num' type='text' password="true" name="userPassword" placeholder="请输入密码" bindinput="passwordInput"></input>
<icon class="iconfont icon-biyan"></icon>
<view class='clear'></view>
</view>
</view>
<view class="button_box">
<button class="button_registe" wx:if="{{canIUse}}" open-type="getUserInfo" bindgetuserinfo="onGotUserInfo">{{judgePage==0?"注册":"登录"}}
<!-- <view class="button_name" bindtap="jumpMy" wx.if="{{canIUse}}" bindgetuserinfo="onGotUserInfo"></view> -->
</button>
</view>
<view class="tips_box">
<view class='agreement_box'>
<view class="agreement_name">登陆/注册即视为同意</view>
<view class="agreement_tips" bindtap="jumpAgreement">注册协议</view>
<view class='clear'></view>
</view>
<view class="registe_account {{judgePage==1?'show':'hide'}}" bindtap="junpRegiste">未注册?点击去注册</view>
<view class="already_account {{judgePage==0?'show':'hide'}}" bindtap="junpLogin">已有账号?点击去登陆</view>
</view>
</view>
确定 取消
5、register.wxss 样式文件:
/* pages/registerPage/register.wxss */
.registe_name {
font-size: 40rpx;
line-height: 110rpx;
text-align: center;
}
.message_box {
margin: 40rpx 0;
}
.registe_message_box {
margin: 34rpx 80rpx;
border-bottom: 2rpx solid #e8e8e8;
padding: 10rpx;
}
.message_code_num {
float: left;
}
.message_code_gain {
color: #2ecc71;
float: right;
font-size: 24rpx;
line-height: 50rpx;
}
.message_password_num {
float: left;
}
.icon-biyan {
float: right;
font-size: 44rpx;
line-height: 54rpx;
}
.button_box {
margin-top: 80rpx;
}
.button_registe {
width: 594rpx;
height: 92rpx;
line-height: 92rpx;
background: #2ecc71;
color: #fff;
}
.agreement_box {
font-size: 24rpx;
margin: 40rpx 0;
text-align: center;
}
.agreement_name{
float:left;
color: #999;
}
.agreement_tips{
float:left;
color: #2ecc71;
}
.tips_box{
text-align: center;
padding:10rpx 158rpx;
}
.already_account{
margin-top:200rpx;
}
.registe_account{
margin-top:100rpx;
}