版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/jj12345jj198999/article/details/82707291
最近项目中需要在linux操作系统下定时监测一些状态信息,如cpu使用率,ddr使用率,网卡带宽,硬盘使用率等。自己写代码时发现很多东西都可以从proc内存文件系统中读到,linux实在太强大了。这里把我写的代码整理在这里,供参考。
common的
/*
* common.h
*
* Created on: 2018��8��16��
* Author: Administrator
*/
#ifndef SRC_COMMON_H_
#define SRC_COMMON_H_
#define CK_TIME 2
void getInfor(char *path, char *name, char infor[]);
void getInforAftSpace(char *path, char *name, char infor[]);
void getInforNotd(char *path, char *name, char infor[]);
void getSysVersion(char *path, char *name, char infor[]);
unsigned char transfer(float temp);
#endif /* SRC_COMMON_H_ */
/*
* common.c
*
* Created on: 2018��8��16��
* Author: Administrator
*/
#include<stdio.h>
#include<string.h>
#include <stdlib.h>
#include <sys/time.h>
#include <fcntl.h>
#include <unistd.h>
#include "common.h"
//get info in the proc file
void getInfor(char *path, char *name, char infor[])
{
int fd = open(path, O_RDONLY);
char store[2000];
int k = 0;
char *p = NULL;
read(fd, store, sizeof(store));
close(fd);
//get store name
p = strstr(store, name);
//skip title
while ((*p < '0') || (*p > '9'))
{
p++;
}
//get number
while ((*p >= '0') && (*p <= '9'))
{
infor[k] = *p;
k++;
p++;
}
infor[k] = '\0';
float fInfor = (float) atoi(infor);
fInfor = fInfor / 1024;
gcvt(fInfor, 5, infor);
}
//get number after space
void getInforAftSpace(char *path, char *name, char infor[])
{
int fd = open(path, O_RDONLY);
char store[2000];
int k = 0;
char *p = NULL;
read(fd, store, sizeof(store));
close(fd);
//get point of the row
p = strstr(store, name);
//skip title
while ((*p < '0') || (*p > '9'))
{
p++;
}
//get numbers
while ((*p >= '0') && (*p <= '9'))
{
infor[k] = *p;
k++;
p++;
}
infor[k] = '\0';
}
//get info node for cpu
void getInforNotd(char *path, char *name, char infor[])
{
int fd = open(path, O_RDONLY);
char store[2000];
int k = 0;
char *p = NULL;
read(fd, store, sizeof(store));
close(fd);
//get point of the row
p = strstr(store, name);
//skip title
do
{
p++;
} while (*p != ':');
p += 2;
//get number
while (*p != '\n')
{
infor[k] = *p;
k++;
p++;
}
infor[k] = '\0';
}
void getSysVersion(char *path, char *name, char infor[])
{
int fd = open(path, O_RDONLY);
char store[2000];
int k = 0;
char *p = NULL;
read(fd, store, sizeof(store));
close(fd);
//get point of the row
p = strstr(store, name);
//get numbers
while (*p != '\n' && *p != ')')
{
infor[k] = *p;
k++;
p++;
}
infor[k++] = ')';
infor[k] = '\0';
}
unsigned char transfer(float temp)
{
unsigned char result;
char buff[100];
int before,end;
memset(buff,0,sizeof(buff));
sprintf(buff,"%.2f",temp);
printf("buff is %s temp is %f\n",buff,temp);
sscanf(buff,"%d.%d",&before,&end);
result=(before<<4)|end;
printf("result is 0x%x before is %d end is %d\n",result,before,end);
return result;
}
cpu的
/*
* cpu.h
*
* Created on: 2018年8月16日
* Author: Administrator
*/
#ifndef SRC_CPU_H_
#define SRC_CPU_H_
float getCpuUseRatio();
void getcpuVersion();
#endif /* SRC_CPU_H_ */
/*
* cpu.c
*
* Created on: 2018��8��16��
* Author: Administrator
*/
#include<stdio.h>
#include<string.h>
#include <stdlib.h>
#include <sys/time.h>
#include <fcntl.h>
#include <unistd.h>
#include "cpu.h"
#include "common.h"
//cpu info
float zuser = 0, ztotal = 0;
//cpu version
char modeName[50];
extern unsigned char bit_info[32];
//get cpu use ratio
float getCpuUseRatio()
{
FILE *fp;
char buffer[1024];
size_t buf;
float useRatio;
float user = 0, nice = 0, sys = 0, idle = 0, iowait = 0;
float all1,all2,idle1,idle2;
fp = fopen("/proc/stat", "r");
buf = fread(buffer, 1, sizeof(buffer), fp);
if (buf == 0)
{
return 0;
}
buffer[buf] = '\0';
sscanf(buffer, "cpu %f %f %f %f %f", &user, &nice, &sys, &idle, &iowait);
if (idle <= 0)
{
idle = 0;
}
all1=user+nice+sys+idle+iowait;
idle1=idle;
rewind(fp);
sleep(CK_TIME);
memset(buffer,0,sizeof(buffer));
user=0;
nice=0;
sys=0;
idle=0;
iowait=0;
buf = fread(buffer, 1, sizeof(buffer), fp);
if (buf == 0)
{
return 0;
}
buffer[buf] = '\0';
sscanf(buffer, "cpu %f %f %f %f %f", &user, &nice, &sys, &idle, &iowait);
if (idle <= 0)
{
idle = 0;
}
all2=user+nice+sys+idle+iowait;
idle2=idle;
fclose(fp);
useRatio=all2-all1;
useRatio = 100 * (useRatio-(idle2-idle1)) / useRatio;
if (useRatio > 100)
{
useRatio = 100;
}
printf("cpu use ratio is %f\n",useRatio);
bit_info[19]=transfer(useRatio);
return useRatio;
}
//get cpu version
void getcpuVersion()
{
getInforNotd("/proc/cpuinfo", "model name", modeName);
printf("modeName is %s\n",modeName);
}
disk硬盘的
/*
* disk.h
*
* Created on: 2018年8月16日
* Author: Administrator
*/
#ifndef SRC_DISK_H_
#define SRC_DISK_H_
float getDiskCapacity();
float getDiskUsage();
#endif /* SRC_DISK_H_ */
/*
* disk.c
*
* Created on: 2018��8��16��
* Author: Administrator
*/
#include<stdio.h>
#include<string.h>
#include <stdlib.h>
#include <sys/time.h>
#include <fcntl.h>
#include <unistd.h>
#include "disk.h"
#include "common.h"
extern unsigned char bit_info[32];
//disk info
float diskCapacity=0,diskUsage=0;
//get disk info
float getDiskCapacity()
{
FILE *fstream=NULL;
char buff[1024];
int errno=0;
memset(buff,0,sizeof(buff));
if(NULL==(fstream=popen("fdisk -l | grep '/dev/sda' -w","r")))
{
fprintf(stderr,"execute command failed:%s",strerror(errno));
return -1;
}
while(NULL!=fgets(buff,sizeof(buff),fstream))
{
//printf("buff is %s\n",buff);
sscanf(buff, "Disk /dev/sda:%f GB",&diskCapacity);
printf("Disk capacity is %f\n",diskCapacity);
}
return diskCapacity;
}
float getDiskUsage()
{
FILE *fstream=NULL;
char buff[1024];
int errno=0;
int whole,used,avail;
memset(buff,0,sizeof(buff));
if(NULL==(fstream=popen("df -h | grep /dev/sda1","r")))
{
fprintf(stderr,"execute command failed:%s",strerror(errno));
return -1;
}
while(NULL!=fgets(buff,sizeof(buff),fstream))
{
sscanf(buff, "/dev/sda1 %dM %dM %dM %f%%", &whole,&used,&avail,&diskUsage);
printf("Disk Usage is %f\n",diskUsage);
}
pclose(fstream);
bit_info[21]=0x00;
bit_info[22]=0x00;
bit_info[23]=0x00;
bit_info[24]=transfer(diskUsage);
return diskUsage;
}
mem内存的
/*
* mem.h
*
* Created on: 2018年8月16日
* Author: Administrator
*/
#ifndef SRC_MEM_H_
#define SRC_MEM_H_
float getmemInfo();
#endif /* SRC_MEM_H_ */
/*
* mem.c
*
* Created on: 2018��8��16��
* Author: Administrator
*/
#include<stdio.h>
#include<string.h>
#include <stdlib.h>
#include <sys/time.h>
#include <fcntl.h>
#include <unistd.h>
#include "mem.h"
#include "common.h"
extern unsigned char bit_info[32];
//mem info
float memUseRatio;
float total_mem;
char totalMem[20], freeMem[20];
float getmemInfo()
{
getInfor("/proc/meminfo", "MemTotal", totalMem);
getInfor("/proc/meminfo", "MemFree", freeMem);
total_mem = (float) atoi(totalMem);
float free_mem = (float) atoi(freeMem);
memUseRatio = 1 - free_mem / total_mem;
bit_info[20]=transfer(memUseRatio);
printf("memUseRatio is %f\n",memUseRatio);
return memUseRatio;
}
net网络的
/*
* net.h
*
* Created on: 2018年8月16日
* Author: Administrator
*/
#ifndef SRC_NET_H_
#define SRC_NET_H_
float getNetInfo(char *name,int flag);
float netStatus();
#endif /* SRC_NET_H_ */
/*
* net.c
*
* Created on: 2018��8��16��
* Author: Administrator
*/
#include<stdio.h>
#include<string.h>
#include <stdlib.h>
#include <sys/time.h>
#include <fcntl.h>
#include <unistd.h>
#include "net.h"
#include "common.h"
//net info
static float cx5BandWidth=40960; //CX5 band width 40Gbps
static float intelBandWidth=1000; //Intel i350 band width 1000Mbps
float cx5netUsage0=0,cx5netUsage1=0;
float netUsage0=0,netUsage1=0,netUsage2=0,netUsage3=0;
extern unsigned char bit_info[32];
//get net info
float getNetInfo(char *name,int flag)
{
char shell_buffer[100];
char result_buffer[1000];
float curRatio;
float netUsage=0;
float rxBytes1=0,txBytes1=0,rxBytes2=0,txBytes2=0;
float temp1=0,temp2=0,temp3=0,temp4=0,temp5=0,temp6=0,temp7=0;
FILE *fstream=NULL;
char buff[1024];
int errno=0;
memset(buff,0,sizeof(buff));
sprintf(shell_buffer,"cat /proc/net/dev| grep %s",name);
printf("%s\n",shell_buffer);
sprintf(result_buffer," %s: %%f %%f %%f %%f %%f %%f %%f %%f %%f",name);
printf("%s\n",result_buffer);
if(NULL==(fstream=popen(shell_buffer,"r")))
{
fprintf(stderr,"execute command failed:%s",strerror(errno));
return -1;
}
while(NULL!=fgets(buff,sizeof(buff),fstream))
{
sscanf(buff, result_buffer, &rxBytes1,&temp1,&temp2,&temp3,&temp4,&temp5,&temp6,&temp7,&txBytes1);
}
//printf("%f %f\n",rxBytes1,txBytes1);
rewind(fstream);
sleep(CK_TIME);
memset(buff,0,sizeof(buff));
if(NULL==(fstream=popen(shell_buffer,"r")))
{
fprintf(stderr,"execute command failed:%s",strerror(errno));
return -1;
}
while(NULL!=fgets(buff,sizeof(buff),fstream))
{
sscanf(buff, result_buffer, &rxBytes2,&temp1,&temp2,&temp3,&temp4,&temp5,&temp6,&temp7,&txBytes2);
}
pclose(fstream);
curRatio=(rxBytes2-rxBytes1+txBytes2-txBytes1)*8/2;
//printf("curRatio is %f\n",curRatio);
if(flag==1)
{
netUsage = curRatio/cx5BandWidth;
}
else
{
netUsage = curRatio/intelBandWidth;
}
if (netUsage > 100)
{
netUsage = 100;
}
printf("%s netUsage is %f\n",name,netUsage);
return netUsage;
}
float netStatus()
{
//get cx5 net usage
cx5netUsage0=getNetInfo("enp2s0f0",1);
bit_info[25]=transfer(cx5netUsage0);
cx5netUsage1=getNetInfo("enp2s0f1",1);
bit_info[26]=transfer(cx5netUsage1);
//get intel i350 net usage
netUsage0=getNetInfo("enp9s0f0",0);
netUsage1=getNetInfo("enp9s0f1",0);
netUsage2=getNetInfo("enp9s0f2",0);
netUsage3=getNetInfo("enp9s0f3",0);
bit_info[27]=transfer(netUsage0);
bit_info[28]=transfer(netUsage1);
bit_info[29]=transfer(netUsage2);
bit_info[30]=transfer(netUsage3);
return 1;
}
temp温度的,前提安装lm_sensor这个工具
/*
* temp.h
*
* Created on: 2018年8月22日
* Author: Administrator
*/
#ifndef SRC_TEMP_H_
#define SRC_TEMP_H_
float getCpuTemp();
#endif /* SRC_TEMP_H_ */
/*
* temp.c
*
* Created on: 2018��8��22��
* Author: Administrator
*/
#include<stdio.h>
#include<string.h>
#include <stdlib.h>
#include <sys/time.h>
#include <fcntl.h>
#include <unistd.h>
#include "common.h"
#include "temp.h"
extern unsigned char bit_info[32];
float getCpuTemp()
{
FILE *fstream=NULL;
char buff[1024];
int errno=0;
float temp11=0;
memset(buff,0,sizeof(buff));
if(NULL==(fstream=popen("sensors | grep Physical","r")))
{
fprintf(stderr,"execute command failed:%s",strerror(errno));
return -1;
}
while(NULL!=fgets(buff,sizeof(buff),fstream))
{
sscanf(buff, "Physical id 0: +%f", &temp11);
printf("CPU temp is %f\n",temp11);
}
bit_info[31]=transfer(temp11);
return temp11;
}
version版本号的
/*
* version.h
*
* Created on: 2018��8��16��
* Author: Administrator
*/
#ifndef SRC_VERSION_H_
#define SRC_VERSION_H_
float getbiosVersion();
float getosVersion();
float getdriverVersion();
#endif /* SRC_VERSION_H_ */
/*
* version.c
*
* Created on: 2018��8��16��
* Author: Administrator
*/
#include<stdio.h>
#include<string.h>
#include <stdlib.h>
#include <sys/time.h>
#include <fcntl.h>
#include <unistd.h>
#include "version.h"
#include "common.h"
extern unsigned char bit_info[32];
//get bios version
float getbiosVersion()
{
FILE *fstream=NULL;
char buff[1024];
int errno=0;
float biosVersion=0;
memset(buff,0,sizeof(buff));
if(NULL==(fstream=popen("dmidecode -t bios | grep 'BIOS Revision' ","r")))
{
fprintf(stderr,"execute command failed:%s",strerror(errno));
return -1;
}
while(NULL!=fgets(buff,sizeof(buff),fstream))
{
sscanf(buff, " BIOS Revision: %f", &biosVersion);
printf("BIOS version is %f\n",biosVersion);
}
bit_info[0]=transfer(biosVersion);
pclose(fstream);
return biosVersion;
}
//get system version
float getosVersion()
{
FILE *fstream=NULL;
char buff[1024];
int errno=0;
int i,flag=0;
memset(buff,0,sizeof(buff));
if(NULL==(fstream=popen("uname -r","r")))
{
fprintf(stderr,"execute command failed:%s",strerror(errno));
return -1;
}
while(NULL!=fgets(buff,sizeof(buff),fstream))
{
for(i=0;buff[i]!='\0';i++)
{
if(buff[i]=='.'&&flag==0)
{
flag=1;
continue;
}
if(buff[i]=='.'&&flag==1)
{
buff[i]='\0';
break;
}
}
}
printf("kernel version is %s\n",buff);
bit_info[1]=transfer((float)atof(buff));
pclose(fstream);
return (float)atof(buff);
}
//get system version
float getdriverVersion()
{
float temp=1.0;
bit_info[2]=transfer(temp);
return temp;
}
最后写了一个测试程序用ipmitool发送给bmc
/*
============================================================================
Name : ipmi_test.c
Author : felven
Version :
Copyright : Your copyright notice
Description : Hello World in C, Ansi-style
============================================================================
*/
#include <stdio.h>
#include <stdlib.h>
#include<unistd.h>
#include <sys/time.h>
#include <string.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <sys/ioctl.h>
#include <sys/poll.h>
#include <sys/time.h>
#include <sys/socket.h>
#include <fcntl.h>
#include <errno.h>
#include <pthread.h>
#include <math.h>
#include <unistd.h>
#include <signal.h>
#include "version.h"
#include "cpu.h"
#include "mem.h"
#include "disk.h"
#include "net.h"
#include "temp.h"
unsigned char bit_info[32]={0};
int main(void)
{
char buff[1024];
int i;
memset(buff,0,sizeof(buff));
//show version info
getbiosVersion();
getosVersion();
getdriverVersion();
//cpu model
bit_info[3]=0x10;
bit_info[4]=0x11;
bit_info[5]=0x12;
bit_info[6]=0x13;
//ram speed
bit_info[7]=0x00;
bit_info[8]=0x00;
bit_info[9]=0x09;
bit_info[10]=0x60;
//ram size
bit_info[11]=0x00;
bit_info[12]=0x00;
bit_info[13]=0x80;
bit_info[14]=0x00;
//ssd size
bit_info[15]=0x00;
bit_info[16]=0x01;
bit_info[17]=0x00;
bit_info[18]=0x00;
//show disk info
getDiskCapacity();
getDiskUsage();
//show ddr info
getmemInfo();
//show cpu info
getcpuVersion();
getCpuUseRatio();
//show temp info
getCpuTemp();
//show net info
//netStatus();
sprintf(buff,"ipmitool raw 6 0x10");
for(i=0;i<32;i++)
{
sprintf(buff,"%s 0x%x",buff,bit_info[i]);
}
printf("%s\n",buff);
system(buff);
//system("ipmitool raw 6 0x10 0x11 0x12 0x13 0x14 0x15 0x16 0x17 0x18 0x19 0x20 0x21 0x22 0x23 0x24 0x25 0x26 0x27 0x28 0x29 0x30 0x31 0x32 0x33 0x34 0x35 0x36 0x37 0x38 0x39 0x40 0x41 0x42");
return 0;
}
扫描二维码关注公众号,回复:
3385136 查看本文章
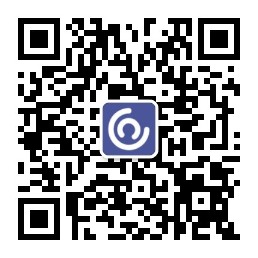