Given an array of integers that is already sorted in ascending order, find two numbers such that they add up to a specific target number.
The function twoSum should return indices of the two numbers such that they add up to the target, where index1 must be less than index2.
Note:
- Your returned answers (both index1 and index2) are not zero-based.
- You may assume that each input would have exactly one solution and you may not use the same element twice.
Example:
Input: numbers = [2,7,11,15], target = 9 Output: [1,2] Explanation: The sum of 2 and 7 is 9. Therefore index1 = 1, index2 = 2.
原题地址: Two Sum II - Input array is sorted
难度: Easy
思路1:
采用缓存的方式,用字典记录每一个值的索引
代码:
class Solution(object): def twoSum(self, numbers, target): """ :type numbers: List[int] :type target: int :rtype: List[int] """ d = {} for idx, num in enumerate(numbers): if target - num in d: idx1 = d[target-num] return [idx1+1, idx+1] else: d[num] = idx
时间复杂度: O(n)
空间复杂度: O(n)
扫描二维码关注公众号,回复:
3416626 查看本文章
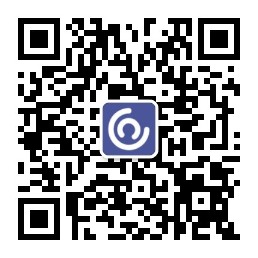
思路2:
因为数组已经排序了,可以分别在数组首尾放置一指针,逐渐向中间夹逼
代码:
class Solution(object): def twoSum(self, numbers, target): """ :type numbers: List[int] :type target: int :rtype: List[int] """ i, j = 0, len(numbers)-1 while i < j: val = numbers[i] + numbers[j] if val == target: return [i+1, j+1] elif val > target: j -= 1 else: i += 1
时间复杂度: O(n)
空间复杂度: O(1)