我的博客针对新手,写的东西都很简单
不懂网络请求的,不懂json数据解析的,可以看我之前的博客
有什么疑问可以加本人QQ:2034969809
下面我们开始正式写代码:
首先是ViewController.swift
storyBoard:
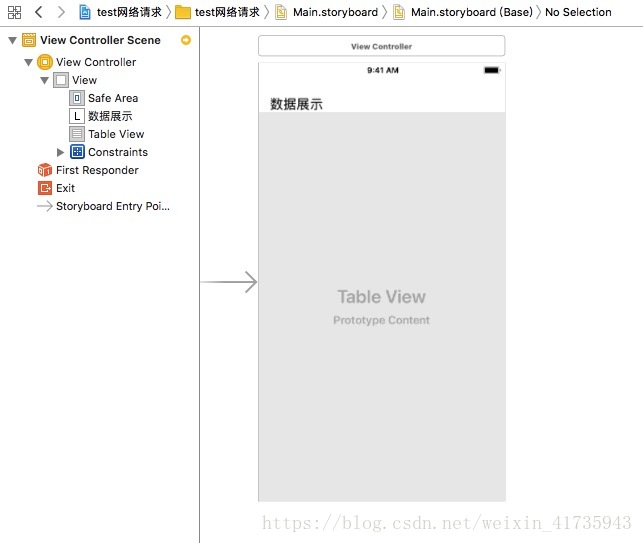
ViewController.swift
//
// ViewController.swift
// URLSesstionTest
//
import UIKit
class ViewController: UIViewController , UITableViewDelegate, UITableViewDataSource {
@IBOutlet weak var tableView: UITableView!
// pragma MARK: === property -- 属性 --- ===
// var tableView:UITableView?
var listDataArray:NSMutableArray?
let name = "许嵩"
override func viewDidLoad() {
super.viewDidLoad()
self.listDataArray = NSMutableArray.init()
tableView.separatorStyle = .none
tableView.register(UINib.init(nibName: "myTableViewCell" , bundle: nil), forCellReuseIdentifier: "myTableViewCellID")
// 请求数据
netWork(keyWord: name)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// pragma MARK: === UITableViewDataSource -- UITableView数据源协议 --- ===
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
if (self.listDataArray?.count)! <= 0 {
return 0
}
return (self.listDataArray?.count)!
// return 5
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let model:MusicModel = self.listDataArray![indexPath.row] as! MusicModel
let cell:myTableViewCell = tableView.dequeueReusableCell(withIdentifier: "myTableViewCellID", for: indexPath) as! myTableViewCell
cell.setCellModelData(model: model)
return cell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
return 75
}
func netWork(keyWord:String) -> (Void) {
// qq音乐搜索接口
var urlString:String = "http://s.music.qq.com/fcgi-bin/music_search_new_platform?t=0&%20n=3&aggr=1&cr=1&loginUin=0&format=json&%20inCharset=GB2312&outCharset=utf-8¬ice=0&%20platform=jqminiframe.json&needNewCode=0&p=1&catZhida=0&%20remoteplace=sizer.newclient.next_song&w="
urlString = urlString + keyWord
// 加密,当传递的参数中含有中文时必须加密
let newUrlString = urlString.addingPercentEncoding(withAllowedCharacters: .urlQueryAllowed)
//创建请求配置
let config = URLSessionConfiguration.default
let url = URL(string: newUrlString!)
let request = URLRequest(url: url!)
let session = URLSession(configuration: config)
// 创建请求任务
let task = session.dataTask(with: request) { (data,response,error) in
do{
// 将二进制数据转换为字典对象
if let jsonObj:NSDictionary = try JSONSerialization.jsonObject(with: data!, options: JSONSerialization.ReadingOptions()) as? NSDictionary
{
// 根据键名,获得字典对象中的键值,并将键值转化为另一个字典对象
if let data:NSDictionary = jsonObj["data"] as? NSDictionary
{
// 根据键名获得第二个字典对象的键值,并将键值转化为一个数组对象
if let song:NSDictionary = data["song"] as? NSDictionary
{
// list 数据
if let list:NSArray = song["list"] as? NSArray
{
let count:Int = list.count
for i in 0 ..< count {
let dic:NSDictionary = list[i] as! NSDictionary
let model:MusicModel = MusicModel()
model.albumName_hilight = (dic["albumName_hilight"] as! String)
model.f = (dic["f"] as! String)
model.fsinger = (dic["fsinger"] as! String)
model.songName_hilight = (dic["songName_hilight"] as! String)
model.singerMID = (dic["singerMID"] as! String)
self.listDataArray?.add(model)
}
print("---- list[9] - myModel.f ------------------------")
let myModel:MusicModel = self.listDataArray![9] as! MusicModel
print(myModel.f ?? "啥都没有")
//这一步很重要!
//这一步很重要!
//这一步很重要!
//在主线程刷新tableView的数据
DispatchQueue.main.async{
self.tableView?.reloadData()
}
}
}
}
}
} catch{
print("Error.")
}
}
// 激活请求任务
task.resume()
// self.tableView?.reloadData()
}
}
然后创建一个myTableViewCell.swift和myTableViewCell.xib文件
myTableViewCell.xib:
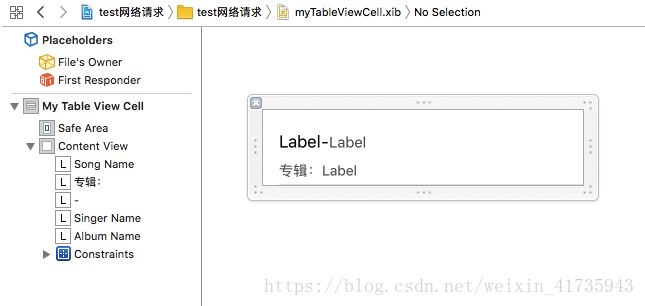
myTableViewCell.swift
//
// myTableViewCell.swift
// test网络请求
//
import UIKit
class myTableViewCell: UITableViewCell {
@IBOutlet weak var songName: UILabel!
@IBOutlet weak var singerName: UILabel!
@IBOutlet weak var albumName: UILabel!
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
public func setCellModelData(model data:MusicModel) -> Void{
self.songName?.text = data.songName_hilight!
self.albumName?.text = data.albumName_hilight!
self.singerName?.text = data.fsinger!
}
}
最后添加一个swift文件用来存放模型
//
// myModel.swift
// test网络请求
//
import UIKit
class MusicModel: NSObject {
var albumName_hilight:String? //专辑名
var f:String?
var fsinger:String? //歌手名
var songName_hilight:String? //歌曲名
var singerMID:String? //歌曲ID
}
写完这些,然后去洗把手,按下command+R
接下来就是见证奇迹的时刻!