错误处理
- try...except...finally...机制
try: print('try...') r = 10 / 0 print('result:', r) except ZeroDivisionError as e: print('except:', e) finally: print('finally...') print('END')
- expect代码错误捕捉机制【错误也是class,栈结构错误机制】
- logging模块的错误记录方式
except Exception as e: logging.exception(e)
- 用
raise
语句抛出一个错误
调试
- 简单粗暴的方法:print
- 凡是用
print()
来辅助查看的地方,都可以用断言(assert)来替代
def foo(s): n = int(s) assert n != 0, 'n is zero!' return 10 / n def main(): foo('0')
$ python err.py Traceback (most recent call last): ... AssertionError: n is zero!
- logging:
import logging logging.basicConfig(level=logging.INFO) s = '0' n = int(s) logging.info('n = %d' % n) print(10 / n) # 输出 $ python err.py INFO:root:n = 0 Traceback (most recent call last): File "err.py", line 8, in <module> print(10 / n) ZeroDivisionError: division by zero
这就是
logging
的好处,它允许你指定记录信息的级别,有debug
,info
,warning
,error
等几个级别,当我们指定level=INFO
时,logging.debug
就不起作用了。同理,指定level=WARNING
后,debug
和info
就不起作用了。这样一来,你可以放心地输出不同级别的信息,也不用删除,最后统一控制输出哪个级别的信息。
logging
的另一个好处是通过简单的配置,一条语句可以同时输出到不同的地方,比如console和文件。
- pdb断点调试
- IDE
如果要比较爽地设置断点、单步执行,就需要一个支持调试功能的IDE。目前比较好的Python IDE有: Visual Studio Code:https://code.visualstudio.com/,需要安装Python插件。 PyCharm:http://www.jetbrains.com/pycharm/
单元调试
- 难度较大,可以先了解
-
单元测试可以有效地测试某个程序模块的行为,是未来重构代码的信心保证。
单元测试的测试用例要覆盖常用的输入组合、边界条件和异常。
单元测试代码要非常简单,如果测试代码太复杂,那么测试代码本身就可能有bug。
单元测试通过了并不意味着程序就没有bug了,但是不通过程序肯定有bug。
文档测试
- doctest方法测试
# -*- coding: utf-8 -*- class Dict(dict): ''' Simple dict but also support access as x.y style. >>> d1 = Dict() >>> d1['x'] = 100 >>> d1.x 100 >>> d1.y = 200 >>> d1['y'] 200 >>> d2 = Dict(a=1, b=2, c='3') >>> d2.c '3' >>> d2['empty'] Traceback (most recent call last): ... KeyError: 'empty' >>> d2.empty Traceback (most recent call last): ... AttributeError: 'Dict' object has no attribute 'empty' ''' def __init__(self, **kw): super(Dict, self).__init__(**kw) def __getattr__(self, key): try: return self[key] except KeyError: raise AttributeError(r"'Dict' object has no attribute '%s'" % key) def __setattr__(self, key, value): self[key] = value if __name__=='__main__': import doctest doctest.testmod()
扫描二维码关注公众号,回复:
3455231 查看本文章
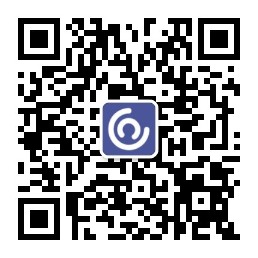