Spring的注入方式:
设值注入:
设值注入就是给该类的属性通过set方法设值。在Spring的配置文件当中,使用<property></property>标签设值。
在<property></property>中,name值对应类中的属性名,且必须一致;ref值则是对应的数据源id,且必须一致。
构造注入:
构造注入就是该该类的属性通过构造方法传参数的方式设值。在Spring配置文件当中使用<constructor-arg></constructor-arg>标签进行设值。
在<constructor-arg></constructor-arg>中,name值对应类中的属性名,且必须一致;ref值则是对应的数据源id,且必须一致。
以打折的实例举例一下:
设置注入:
打折计算的接口:
public interface IDiscounts { public double discount(double price); }
扫描二维码关注公众号,回复:
345763 查看本文章
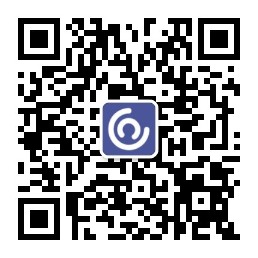
半价实体:
public class HalfPrice implements IDiscounts { @Override public double discount(double price) { return price * 0.5; } }
不打折实体:
public class FullPrice implements IDiscounts { @Override public double discount(double price) { return price; } }
结算实体:
public class Accounts {
private IDiscounts iDiscounts;//打折的接口,必须实现set方法
public double account(double price) {
return iDiscounts.discount(price);
}
}
Spring配置文件:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE beans PUBLIC "-//SPRING/DTD BEAN/EN" "http://www.springframework.org/dtd/spring-beans.dtd"> <beans> <!-- 添加每一个bean --> <!-- id为别号,class为相对应的类 --> <bean id="fullPrice" class="com.usc.geowind.lilin.bean.inflood.FullPrice" /> <bean id="halfPrice" class="com.usc.geowind.lilin.bean.inflood.HalfPrice" /> <bean id="account" class="com.usc.geowind.lilin.bean.inflood.Accounts"> <!-- 设值注入 --> <!-- name是该类对应的属性名,必须一致 ; --> <!-- ref映射:值为该属性参数对应的数据源。比如上面的数据源ID:fullPrice --> <property name="iDiscounts" ref="fullPrice"></property> </bean> </beans>
程序入口:
public static void main(String[] args) { // 读取配置文件 Resource resource = new FileSystemResource("shop.xml"); // 加载配置文件 ,启动IOC容器 BeanFactory factory = new XmlBeanFactory(resource); // 从IOC容器中获取实例 Accounts accounts = factory.getBean(Accounts.class); System.out.println(accounts.account(40.0)); }
构造注入:
结算实体做一下修改:
public class Accounts { private IDiscounts iDiscounts; public Accounts(IDiscounts iDiscounts) { this.iDiscounts = iDiscounts; } public double account(double price) { return iDiscounts.discount(price); } }
Spring配置文件:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE beans PUBLIC "-//SPRING/DTD BEAN/EN" "http://www.springframework.org/dtd/spring-beans.dtd"> <beans> <!-- 添加每一个bean --> <!-- id为别号,class为相对应的类 --> <bean id="fullPrice" class="com.usc.geowind.lilin.bean.inflood.FullPrice" /> <bean id="halfPrice" class="com.usc.geowind.lilin.bean.inflood.HalfPrice" /> <bean id="account" class="com.usc.geowind.lilin.bean.inflood.Accounts"> <!-- 构造注入 --> <!-- 顺序与构造方法参数顺序一致 --> <!-- ref映射:值为该属性参数对应的数据源。比如上面的数据源ID:fullPrice --> <constructor-arg ref="halfPrice"></constructor-arg> </bean> </beans>