简单的学生信息处理程序实现
来源: POJ (Coursera声明:在POJ上完成的习题将不会计入Coursera的最后成绩。)
注意: 总时间限制: 1000ms 内存限制: 65536kB
描述
在一个学生信息处理程序中,要求实现一个代表学生的类,并且所有成员变量都应该是私有的。
(注:评测系统无法自动判断变量是否私有。我们会在结束之后统一对作业进行检查,请同学们严格按照题目要求完成,否则可能会影响作业成绩。)
输入
姓名,年龄,学号,第一学年平均成绩,第二学年平均成绩,第三学年平均成绩, 第四学年平均成绩。其中姓名、学号为字符串,不含空格和逗号;年龄为正整数;成绩为非负整数。各部分内容之间均用单个英文逗号”,”隔开,无多余空格。输出一行,按顺序输出:姓名,年龄,学号,四年平均成绩(向下取整)。
各部分内容之间均用单个英文逗号”,”隔开,无多余空格。
样例输入
Tom,18,7817,80,80,90,70
样例输出
Tom,18,7817,80
习题来源:http://cxsjsxmooc.openjudge.cn/test/C/
扫描二维码关注公众号,回复:
3577731 查看本文章
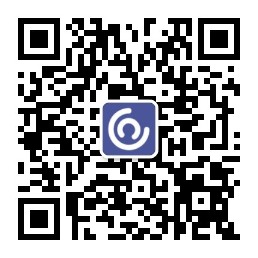
实现代码如下:
#include "stdafx.h"
#include<iostream>
#include <stdlib.h>
#include <string.h>
using namespace std;
class StuInfo
{
private: char *pname = new char(sizeof(char)), *pnum = new char(sizeof(char));
int age, gra1, gra2, gra3, gra4;
int average;
public:
StuInfo()
{
}
~StuInfo()
{
delete(pname);
delete(pnum);
}
int get_average(int a[],int cout);
char get_name(char a);
char get_num(char a);
int get_ingre(char *a);
};
int main()
{
char *na = new char(sizeof(char)), *n = new char(sizeof(char)), a[7][15] = {0};
int age,g, gra[4] = {0};
StuInfo A;
int i = 0;
for (i = 0; i < 6; i++)
cin.getline(a[i], 15, ',');
cin.getline(a[i], 5);
na = a[0];
n = a[1];
age = A.get_ingre(a[2]);
for (i = 0; i < 4; i++)
{
gra[i] = A.get_ingre(a[3+i]);
}
g = A.get_average(gra,i);
cout << na << ',' << n <<',' << age << ',' << g << endl;
delete(na);
delete(n);
system("pause");
return 0;
}
char StuInfo::get_num(char a)
{
return a;
}
char StuInfo::get_name(char a)
{
return a;
}
int StuInfo::get_ingre(char a[])
{
return atoi(a);
}
int StuInfo::get_average(int a[],int cout)
{
int i;
float average = 0;
for (i = 0; i < cout; i++)
{
average += a[i];
}
return floor(average / cout);
}
注释:
common:
private: 私有元素,内部对象函数才可使用。
StuInfo()与~StuInfo(): 分别为构造函数和析构函数。可用于类对象的初始化和类对象的结束解析。
"::": 作用域运算符。表示该函数被声明在类A的作用域内。
getline:
basic_istream& getline( char_type* s, std::streamsize count ); (1)
basic_istream& getline( char_type* s, std::streamsize count, char_type delim ); (2)
s - pointer to the character string to store the characters to
count - size of character string pointed to by s
delim - delimiting character to stop the extraction at. It is extracted but not stored.
int atoi( const char *str );
Interprets an integer value in a byte string pointed to by str
str - pointer to the null-terminated byte string to be interpreted
double floor( double arg );
Computes the largest integer value not greater than arg.
arg - floating point value
void* operator new ( std::size_t count ); (1)
void* operator new[]( std::size_t count ); (2)
(1) Called by non-array new-expressions to allocate storage required for a single object. The standard library implementation allocates count bytes from free store. In case of failure, the standard library implementation calls the function pointer returned by std::get_new_handler and repeats allocation attempts until new handler does not return or becomes a null pointer, at which time it throws std::bad_alloc. This function is required to return a pointer suitably aligned to hold an object of any fundamental alignment.
(2) Called by the array form of new[]-expressions to allocate all storage required for an array (including possible new-expression overhead). The standard library implementation calls version (1)
count - number of bytes to allocate
void operator delete ( void* ptr ); (1)
void operator delete[]( void* ptr ); (2)
1) Called by delete-expressions to deallocate storage previously allocated for a single object. The behavior of the standard library implementation of this function is undefined unless ptr is a null pointer or is a pointer previously obtained from the standard library implementation of operator new(size_t) or operator new(size_t, std::nothrow_t).
2) Called by delete[]-expressions to deallocate storage previously allocated for an array of objects. The behavior of the standard library implementation of this function is undefined unless ptr is a null pointer or is a pointer previously obtained from the standard library implementation of operator new[](size_t) or operator new[](size_t, std::nothrow_t).
ptr - pointer to a memory block to deallocate or a null pointer