Hey Friend, Ari here from Fullstack React. Today, let's talk a bit about props and state , two core concepts in React that we'll use in every React application. The short of it is, props are like the "arguments" (or properties) that you pass into your components and state stores "component-local data". Let's look at an example to make it concrete:
Let's say that we have a <Header /> component that includes a title. The code might look something like this:
class Header extends React.Component {
render() {
return (<h1>A
title</h1>)
}
}
|
|
We want to reuse our <Header /> component in multiple parts of our app. Of course, not every header in our application will have the exact text A title , like this one. It's not convenient to update our component's template directly every time we want a new h1 title. Instead, let's provide the component the header text (the title) as an "argument". props React allows us to send data to a component using the same syntax as HTML: using attributes or properties. This is similar to passing the src attribute to an <img /> tag. We can consider the property of the <img /> tag as a prop that we are setting on a component called img . We can access these properties inside a component using this.props from anywhere inside our component. For example, let's update our <Header /> component so that we can reuse in multiple places.
class Header extends React.Component {
render() {
return <h1>{this.props.title}</h1>
}
}
|
|
Now we can use the <Header /> component and pass a title as an attribute to have a dynamic value show up:
<!-- on the homepage -->
<Header title="Home" />
<!-- this would render as: -->
<h1>Home</h1>
|
|
or on the inbox page:
<!-- on the inbox page -->
<Header title="Inbox" />
<!-- this would render as: -->
<h1>Inbox</h1>
|
|
We can pass more than just strings in a component: can pass numbers, arrays, objects, and even functions! The state of things What if we want to change something in our component after the props have already been set? React does not allow us to modify this.props on our components (because it can introduce data consistency issues). Sometimes a component needs to be able to update it's own data. For example, setting an active status for a user in a chat or updating a timer on a stopwatch. While it's preferable to use props as much as we can (that is, pass the data value down from a parent component), sometimes we need to store the local state of a component, within the component itself. To handle this, React gives us the ability to define (and update) it's state . The state in a component is intended to be completely internal to the individual component and it's children (i.e. accessed only by the component and any children it creates). Similar to how we access this.props , the state can be accessed via this.state in a component. Whenever the state changes, the component re-renders (we change state using a special function this.setState() , more on that in a moment). Let's build a stateful clock component using this idea. In order to create a stateful component, we have to tell React we have a stateful component. We do this by assigning a variable called state on the component. Usually, we'll handle this in the constructor of the class, like so:
class Clock extends React.Component {
constructor(props) {
super(props); // We always need this
line
this.state = { time:
new Date() }
}
render() {
// ...
}
}
|
|
Now that we have the state available in the component, we can use it in our render function. Let's show the current time (from our state ) in our render() function:
render() {
return (
<div>{ this.state.time.toString() }</div>
)
}
|
|
We'll need a way to update the time in our state object once every second.
Lifecycle hooks
React allows us to run functions at the different stages of a component while it's on a page. We refer to these functions as lifecycle hooks. We'll skip over looking at these in detail to focus on state for now.
Let's set this up so that we have a timer that fires in one second and updates the state in our component:
// lifecycle hook function
componentDidMount() {
this.updateClock()
}
updateClock() {
this.timerId = setTimeout(() => {
this.setState({
time: new Date()
})
}, 1000)
}
|
|
Checkout the complete, runnable example in jsbin here. In 1000 milliseconds (1 second), our timeout will fire, which in turn calls this.setState() on the component and React automatically re-renders our component. We'll want to make sure the timeout gets fired on an interval, so every second the state is updated. The this.setState() function accepts a function it will call after the state is updated we can define in the second argument.
updateClock() {
this.timerId = setTimeout(() => {
this.setState({
time: new Date()
// reset the timeout after state updates
}, this.updateClock)
}, 1000)
}
|
|
That's it. Now we have a fully stateful component which we can use inside the <Clock /> component. Now, that we have walked through props and state , we can start building more complex applications. Cheers! Ari 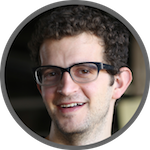 P.S. A lot of folks ask, "what if I need to use the current state in the calculation for the next state?" In that case, you need to use a special form of setState -- we talk about it in our book. |